mirror of
https://github.com/TotalFreedomMC/TotalFreedomMod.git
synced 2024-06-21 05:20:37 +00:00
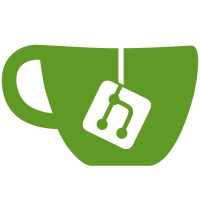
Lombok implementation removal. I have also gone through and replaced things with inline methods and variables, lambdas, and simplified loops down, removed unnecessary guard clauses, and overall cleaned up every single class. This took a long time, please do remember to follow proper naming conventions, don't include unnecessary guard clauses, follow exception rules and comment rules, and please PLEASE remember to use the DIAMOND OPERATOR rather than just inferring RAW TYPES!!! Thank you!!
48 lines
1.8 KiB
Java
48 lines
1.8 KiB
Java
package me.totalfreedom.totalfreedommod.command;
|
|
|
|
import me.totalfreedom.totalfreedommod.rank.Rank;
|
|
import me.totalfreedom.totalfreedommod.util.FUtil;
|
|
import org.bukkit.ChatColor;
|
|
import org.bukkit.Material;
|
|
import org.bukkit.command.Command;
|
|
import org.bukkit.command.CommandSender;
|
|
import org.bukkit.entity.Player;
|
|
import org.bukkit.inventory.ItemStack;
|
|
import org.bukkit.inventory.meta.ItemMeta;
|
|
|
|
@CommandPermissions(level = Rank.ADMIN, source = SourceType.BOTH)
|
|
@CommandParameters(description = "For the people that are still alive - gives a cake to everyone on the server.", usage = "/<command>")
|
|
public class Command_cake extends FreedomCommand
|
|
{
|
|
|
|
public static final String CAKE_LYRICS = "But there's no sense crying over every mistake. You just keep on trying till you run out of cake.";
|
|
|
|
@Override
|
|
public boolean run(final CommandSender sender, final Player playerSender, final Command cmd, final String commandLabel, final String[] args, final boolean senderIsConsole)
|
|
{
|
|
final StringBuilder output = new StringBuilder();
|
|
|
|
for (final String word : CAKE_LYRICS.split(" "))
|
|
{
|
|
output.append(FUtil.randomChatColor()).append(word).append(" ");
|
|
}
|
|
|
|
final ItemStack heldItem = new ItemStack(Material.CAKE);
|
|
final ItemMeta heldItemMeta = heldItem.getItemMeta();
|
|
assert heldItemMeta != null;
|
|
heldItemMeta.setDisplayName(ChatColor.WHITE + "The " + ChatColor.DARK_GRAY + "Lie");
|
|
heldItem.setItemMeta(heldItemMeta);
|
|
|
|
for (Player player : server.getOnlinePlayers())
|
|
{
|
|
final int firstEmpty = player.getInventory().firstEmpty();
|
|
if (firstEmpty >= 0)
|
|
{
|
|
player.getInventory().setItem(firstEmpty, heldItem);
|
|
}
|
|
}
|
|
|
|
FUtil.bcastMsg(output.toString());
|
|
return true;
|
|
}
|
|
} |