mirror of
https://github.com/TotalFreedomMC/TotalFreedomMod.git
synced 2024-05-15 17:11:26 +00:00
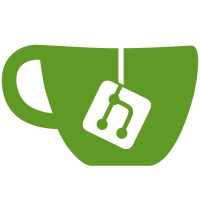
Here's a list of things I've fixed/mitigated: - Range bans in ban-related commands (FS-209) - Bug in /tempban where the duration in the broadcast is always wrong - Over-complicated handling of /tempban durations (instead of trying to process them and silently fail, it'll flat out say that an invalid duration is well, invalid) - Inconsistent "player not found" messages in banning commands - (Mitigates) weird issue with case-sensitive usernames in banning commands when the server has restarted since a player last joined - (Mitigates) banned IPs being inaccurate when a player was banned offline whilst containing multiple IPs in their playerdata - Redoing how MovementValidator handled positive and negative infinity exploit items, completely removing the need for Mojangson in the first place and allowing the plugin to run without Essentials once more in the process (related to FS-406) Here is what I've done in addition: - Merged /tban and /noob into /tempban, which now bans for 5 minutes by default (FS-205)
134 lines
4.4 KiB
Java
134 lines
4.4 KiB
Java
package me.totalfreedom.totalfreedommod.command;
|
|
|
|
import me.totalfreedom.totalfreedommod.admin.Admin;
|
|
import me.totalfreedom.totalfreedommod.banning.Ban;
|
|
import me.totalfreedom.totalfreedommod.config.ConfigEntry;
|
|
import me.totalfreedom.totalfreedommod.discord.Discord;
|
|
import me.totalfreedom.totalfreedommod.punishments.Punishment;
|
|
import me.totalfreedom.totalfreedommod.punishments.PunishmentType;
|
|
import me.totalfreedom.totalfreedommod.rank.Rank;
|
|
import me.totalfreedom.totalfreedommod.util.FUtil;
|
|
import org.apache.commons.lang.ArrayUtils;
|
|
import org.apache.commons.lang.StringUtils;
|
|
import org.bukkit.ChatColor;
|
|
import org.bukkit.GameMode;
|
|
import org.bukkit.command.Command;
|
|
import org.bukkit.command.CommandSender;
|
|
import org.bukkit.entity.Player;
|
|
import org.bukkit.scheduler.BukkitRunnable;
|
|
import org.bukkit.util.Vector;
|
|
|
|
@CommandPermissions(level = Rank.SENIOR_ADMIN, source = SourceType.ONLY_CONSOLE, blockHostConsole = true)
|
|
@CommandParameters(description = "Sends the specified player to their doom.", usage = "/<command> <playername> [reason]")
|
|
public class Command_doom extends FreedomCommand
|
|
{
|
|
|
|
@Override
|
|
public boolean run(final CommandSender sender, Player playerSender, Command cmd, String commandLabel, String[] args, boolean senderIsConsole)
|
|
{
|
|
if (args.length == 0)
|
|
{
|
|
return false;
|
|
}
|
|
|
|
final Player player = getPlayer(args[0]);
|
|
|
|
if (player == null)
|
|
{
|
|
msg(PLAYER_NOT_FOUND);
|
|
return true;
|
|
}
|
|
|
|
FUtil.adminAction(sender.getName(), "Casting oblivion over " + player.getName(), true);
|
|
FUtil.bcastMsg(player.getName() + " will be completely obliviated!", ChatColor.RED);
|
|
|
|
final String ip = FUtil.getIp(player);
|
|
|
|
// Remove from admin
|
|
Admin admin = getAdmin(player);
|
|
if (admin != null)
|
|
{
|
|
FUtil.adminAction(sender.getName(), "Removing " + player.getName() + " from the admin list", true);
|
|
admin.setActive(false);
|
|
plugin.al.save(admin);
|
|
plugin.al.updateTables();
|
|
plugin.ptero.updateAccountStatus(admin);
|
|
if (plugin.dc.enabled && ConfigEntry.DISCORD_ROLE_SYNC.getBoolean())
|
|
{
|
|
Discord.syncRoles(admin, plugin.pl.getData(admin.getName()).getDiscordID());
|
|
}
|
|
}
|
|
|
|
// Remove from whitelist
|
|
player.setWhitelisted(false);
|
|
|
|
// Deop
|
|
player.setOp(false);
|
|
|
|
String reason = null;
|
|
|
|
if (args.length > 1)
|
|
{
|
|
reason = StringUtils.join(ArrayUtils.subarray(args, 1, args.length), " ");
|
|
}
|
|
|
|
// Ban player
|
|
Ban ban = Ban.forPlayer(player, sender);
|
|
ban.setReason((reason == null ? "FUCKOFF" : reason));
|
|
ban.addIp(ip);
|
|
plugin.bm.addBan(ban);
|
|
|
|
// Set gamemode to survival
|
|
player.setGameMode(GameMode.SURVIVAL);
|
|
|
|
// Clear inventory
|
|
player.closeInventory();
|
|
player.getInventory().clear();
|
|
|
|
// Ignite player
|
|
player.setFireTicks(10000);
|
|
|
|
// Generate explosion
|
|
player.getWorld().createExplosion(player.getLocation(), 0F, false);
|
|
|
|
// Shoot the player in the sky
|
|
player.setVelocity(player.getVelocity().clone().add(new Vector(0, 20, 0)));
|
|
|
|
final String kickReason = (reason == null ? "FUCKOFF, and get your shit together!" : reason);
|
|
|
|
// Log doom
|
|
plugin.pul.logPunishment(new Punishment(player.getName(), FUtil.getIp(player), sender.getName(), PunishmentType.DOOM, reason));
|
|
|
|
new BukkitRunnable()
|
|
{
|
|
@Override
|
|
public void run()
|
|
{
|
|
// strike lightning
|
|
player.getWorld().strikeLightningEffect(player.getLocation());
|
|
|
|
// kill (if not done already)
|
|
player.setHealth(0.0);
|
|
}
|
|
}.runTaskLater(plugin, 2L * 20L);
|
|
|
|
new BukkitRunnable()
|
|
{
|
|
@Override
|
|
public void run()
|
|
{
|
|
// message
|
|
FUtil.adminAction(sender.getName(), "Banning " + player.getName(), true);
|
|
msg(sender, player.getName() + " has been banned and IP is: " + ip);
|
|
|
|
// generate explosion
|
|
player.getWorld().createExplosion(player.getLocation(), 0F, false);
|
|
|
|
// kick player
|
|
player.kickPlayer(ChatColor.RED + kickReason);
|
|
}
|
|
}.runTaskLater(plugin, 3L * 20L);
|
|
|
|
return true;
|
|
}
|
|
} |