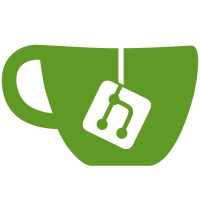
from Ori_B: There were a small number of changes needed from the tarball on spinroot.org: - The mkfile needed to be updated - Memory.h needed to not be included - It needed to invoke /bin/cpp instead of gcc -E - It depended on `yychar`, which our yacc doesn't provide. I'm still figuring out how to use spin, but it seems to do the right thing when testing a few of the examples: % cd $home/src/Spin/Examples/ % spin -a peterson.pml % pcc pan.c -D_POSIX_SOURCE % ./6.out (Spin Version 6.4.7 -- 19 August 2017) + Partial Order Reduction Full statespace search for: never claim - (none specified) assertion violations + acceptance cycles - (not selected) invalid end states + State-vector 32 byte, depth reached 24, errors: 0 40 states, stored 27 states, matched 67 transitions (= stored+matched) 0 atomic steps hash conflicts: 0 (resolved) Stats on memory usage (in Megabytes): 0.002 equivalent memory usage for states (stored*(State-vector + overhead)) 0.292 actual memory usage for states 128.000 memory used for hash table (-w24) 0.534 memory used for DFS stack (-m10000) 128.730 total actual memory usage unreached in proctype user /tmp/Spin/Examples/peterson.pml:20, state 10, "-end-" (1 of 10 states) pan: elapsed time 1.25 seconds pan: rate 32 states/second
118 lines
2.2 KiB
C
118 lines
2.2 KiB
C
/***** tl_spin: tl_mem.c *****/
|
|
|
|
/*
|
|
* This file is part of the public release of Spin. It is subject to the
|
|
* terms in the LICENSE file that is included in this source directory.
|
|
* Tool documentation is available at http://spinroot.com
|
|
*
|
|
* Based on the translation algorithm by Gerth, Peled, Vardi, and Wolper,
|
|
* presented at the PSTV Conference, held in 1995, Warsaw, Poland 1995.
|
|
*/
|
|
|
|
#include "tl.h"
|
|
|
|
#if 1
|
|
#define log(e, u, d) event[e][(int) u] += (long) d;
|
|
#else
|
|
#define log(e, u, d)
|
|
#endif
|
|
|
|
#define A_LARGE 80
|
|
#define A_USER 0x55000000
|
|
#define NOTOOBIG 32768
|
|
|
|
#define POOL 0
|
|
#define ALLOC 1
|
|
#define FREE 2
|
|
#define NREVENT 3
|
|
|
|
extern unsigned long All_Mem;
|
|
extern int tl_verbose;
|
|
|
|
union M {
|
|
long size;
|
|
union M *link;
|
|
};
|
|
|
|
static union M *freelist[A_LARGE];
|
|
static long req[A_LARGE];
|
|
static long event[NREVENT][A_LARGE];
|
|
|
|
void *
|
|
tl_emalloc(int U)
|
|
{ union M *m;
|
|
long r, u;
|
|
void *rp;
|
|
|
|
u = (long) ((U-1)/sizeof(union M) + 2);
|
|
|
|
if (u >= A_LARGE)
|
|
{ log(ALLOC, 0, 1);
|
|
if (tl_verbose)
|
|
printf("tl_spin: memalloc %ld bytes\n", u);
|
|
m = (union M *) emalloc((int) u*sizeof(union M));
|
|
All_Mem += (unsigned long) u*sizeof(union M);
|
|
} else
|
|
{ if (!freelist[u])
|
|
{ r = req[u] += req[u] ? req[u] : 1;
|
|
if (r >= NOTOOBIG)
|
|
r = req[u] = NOTOOBIG;
|
|
log(POOL, u, r);
|
|
freelist[u] = (union M *)
|
|
emalloc((int) r*u*sizeof(union M));
|
|
All_Mem += (unsigned long) r*u*sizeof(union M);
|
|
m = freelist[u] + (r-2)*u;
|
|
for ( ; m >= freelist[u]; m -= u)
|
|
m->link = m+u;
|
|
}
|
|
log(ALLOC, u, 1);
|
|
m = freelist[u];
|
|
freelist[u] = m->link;
|
|
}
|
|
m->size = (u|A_USER);
|
|
|
|
for (r = 1; r < u; )
|
|
(&m->size)[r++] = 0;
|
|
|
|
rp = (void *) (m+1);
|
|
memset(rp, 0, U);
|
|
return rp;
|
|
}
|
|
|
|
void
|
|
tfree(void *v)
|
|
{ union M *m = (union M *) v;
|
|
long u;
|
|
|
|
--m;
|
|
if ((m->size&0xFF000000) != A_USER)
|
|
Fatal("releasing a free block", (char *)0);
|
|
|
|
u = (m->size &= 0xFFFFFF);
|
|
if (u >= A_LARGE)
|
|
{ log(FREE, 0, 1);
|
|
/* free(m); */
|
|
} else
|
|
{ log(FREE, u, 1);
|
|
m->link = freelist[u];
|
|
freelist[u] = m;
|
|
}
|
|
}
|
|
|
|
void
|
|
a_stats(void)
|
|
{ long p, a, f;
|
|
int i;
|
|
|
|
printf(" size\t pool\tallocs\t frees\n");
|
|
for (i = 0; i < A_LARGE; i++)
|
|
{ p = event[POOL][i];
|
|
a = event[ALLOC][i];
|
|
f = event[FREE][i];
|
|
|
|
if(p|a|f)
|
|
printf("%5d\t%6ld\t%6ld\t%6ld\n",
|
|
i, p, a, f);
|
|
}
|
|
}
|