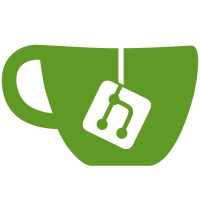
a portable SG_NOEXEC segment attribute was added to allow non-executable (physical) segments. which will set the PTENOEXEC bits for putmmu(). in the future, this can be used to make non-executable stack / bss segments. the SG_DEVICE attribute was added to distinguish between mmio regions and uncached memory. only matterns on arm64. on arm, theres the issue that PTEUNCACHED would have no bits set when using the hardware bit definitions. this is the reason bcm, kw, teg2 and omap kernels use arteficial PTE constants. on zynq, the XN bit was used as a hack to give PTEUNCACHED a non-zero value and when the bit is clear then cache attributes where added to the pte. to fix this, PTECACHED constant was added. the portable mmu code in fault.c will now explicitely set PTECACHED bits for cached memory and PTEUNCACHED for uncached memory. that way the hardware bit definitions can be used everywhere.
101 lines
2.7 KiB
C
101 lines
2.7 KiB
C
/*
|
|
* Memory and machine-specific definitions. Used in C and assembler.
|
|
*/
|
|
#define KiB 1024u /* Kibi 0x0000000000000400 */
|
|
#define MiB 1048576u /* Mebi 0x0000000000100000 */
|
|
#define GiB 1073741824u /* Gibi 000000000040000000 */
|
|
|
|
/*
|
|
* Sizes
|
|
*/
|
|
#define BY2PG (4*KiB) /* bytes per page */
|
|
#define PGSHIFT 12 /* log(BY2PG) */
|
|
#define PGROUND(s) ROUND(s, BY2PG)
|
|
#define ROUND(s, sz) (((s)+(sz-1))&~(sz-1))
|
|
|
|
#define MAXMACH 4 /* max # cpus system can run */
|
|
#define MACHSIZE BY2PG
|
|
#define L1SIZE (4 * BY2PG)
|
|
|
|
#define KSTACK (8*KiB)
|
|
#define STACKALIGN(sp) ((sp) & ~3) /* bug: assure with alloc */
|
|
|
|
/*
|
|
* Magic registers
|
|
*/
|
|
|
|
#define USER 9 /* R9 is up-> */
|
|
#define MACH 10 /* R10 is m-> */
|
|
|
|
/*
|
|
* Address spaces.
|
|
* KTZERO is used by kprof and dumpstack (if any).
|
|
*
|
|
* KZERO is mapped to physical 0 (start of ram).
|
|
*
|
|
* vectors are at 0, plan9.ini is at KZERO+256 and is limited to 16K by
|
|
* devenv.
|
|
*/
|
|
|
|
#define KSEG0 0x80000000 /* kernel segment */
|
|
/* mask to check segment; good for 1GB dram */
|
|
#define KSEGM 0xC0000000
|
|
#define KZERO KSEG0 /* kernel address space */
|
|
#define CONFADDR (KZERO+0x100) /* unparsed plan9.ini */
|
|
#define REBOOTADDR (0x1c00) /* reboot code - physical address */
|
|
#define MACHADDR (KZERO+0x2000) /* Mach structure */
|
|
#define L2 (KZERO+0x3000) /* L2 ptes for vectors etc */
|
|
#define VCBUFFER (KZERO+0x3400) /* videocore mailbox buffer */
|
|
#define FIQSTKTOP (KZERO+0x4000) /* FIQ stack */
|
|
#define L1 (KZERO+0x4000) /* tt ptes: 16KiB aligned */
|
|
#define KTZERO (KZERO+0x8000) /* kernel text start */
|
|
#define VIRTIO (0x7E000000) /* i/o registers */
|
|
#define ARMLOCAL (0x7F000000) /* armv7 only */
|
|
#define VGPIO (ARMLOCAL+MiB) /* virtual gpio for pi3 ACT LED */
|
|
#define FRAMEBUFFER 0xC0000000 /* video framebuffer */
|
|
|
|
#define UZERO 0 /* user segment */
|
|
#define UTZERO (UZERO+BY2PG) /* user text start */
|
|
#define USTKTOP 0x40000000 /* user segment end +1 */
|
|
#define USTKSIZE (8*1024*1024) /* user stack size */
|
|
|
|
/*
|
|
* Legacy...
|
|
*/
|
|
#define BLOCKALIGN 64 /* only used in allocb.c */
|
|
|
|
/*
|
|
* Sizes
|
|
*/
|
|
#define BI2BY 8 /* bits per byte */
|
|
#define BY2SE 4
|
|
#define BY2WD 4
|
|
#define BY2V 8 /* only used in xalloc.c */
|
|
|
|
#define PTEMAPMEM (1024*1024)
|
|
#define PTEPERTAB (PTEMAPMEM/BY2PG)
|
|
#define SEGMAPSIZE 1984
|
|
#define SSEGMAPSIZE 16
|
|
#define PPN(x) ((x)&~(BY2PG-1))
|
|
|
|
/*
|
|
* These bits are completely artificial.
|
|
* With a little work these move to port.
|
|
*/
|
|
#define PTEVALID (1<<0)
|
|
#define PTERONLY 0
|
|
#define PTEWRITE (1<<1)
|
|
#define PTECACHED 0
|
|
#define PTEUNCACHED (1<<2)
|
|
#define PTENOEXEC (1<<4)
|
|
|
|
/*
|
|
* Physical machine information from here on.
|
|
* PHYS addresses as seen from the arm cpu.
|
|
* BUS addresses as seen from the videocore gpu.
|
|
*/
|
|
#define PHYSDRAM 0
|
|
|
|
#define MIN(a, b) ((a) < (b)? (a): (b))
|
|
#define MAX(a, b) ((a) > (b)? (a): (b))
|