mirror of
https://github.com/TotalFreedomMC/TF-ProjectKorra.git
synced 2024-05-15 17:31:26 +00:00
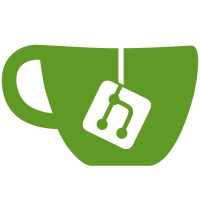
Created a cancellable event that will fire whenever a bending move would alter the velocity of an entity. ## Additions * Adds 1. > The AbilityVelocityAffectEntityEvent > Adds a new method to GeneralMethods -> setEntityVelocity() > Updates existing setVelocity() calls to use the new method in general methods. > checks to see if anything in the event should be modified or if it should be cancelled. ## Fixes * Fixes 2 > The lack of a way to detect when a bending move pushed a player.
76 lines
1.6 KiB
Java
76 lines
1.6 KiB
Java
package com.projectkorra.projectkorra.event;
|
|
|
|
import org.bukkit.entity.Entity;
|
|
import org.bukkit.event.Cancellable;
|
|
import org.bukkit.event.Event;
|
|
import org.bukkit.event.HandlerList;
|
|
import org.bukkit.util.Vector;
|
|
|
|
import com.projectkorra.projectkorra.ability.Ability;
|
|
|
|
/**
|
|
* Cancellable event called when an ability would push or alter the velocity of
|
|
* an entity.
|
|
*
|
|
* the entity can be changed, vector can be modified, and the ability that
|
|
* caused the change can be accessed.
|
|
*
|
|
* @author dNiym
|
|
*
|
|
*/
|
|
|
|
public class AbilityVelocityAffectEntityEvent extends Event implements Cancellable {
|
|
|
|
Entity affected;
|
|
Vector velocity;
|
|
Ability ability;
|
|
boolean cancelled = false;
|
|
|
|
private static final HandlerList handlers = new HandlerList();
|
|
|
|
public AbilityVelocityAffectEntityEvent(Ability ability, Entity entity, Vector vector) {
|
|
this.affected = entity;
|
|
this.ability = ability;
|
|
this.velocity = vector;
|
|
}
|
|
|
|
@Override
|
|
public boolean isCancelled() {
|
|
return cancelled;
|
|
}
|
|
|
|
@Override
|
|
public void setCancelled(boolean cancel) {
|
|
this.cancelled = cancel;
|
|
}
|
|
|
|
@Override
|
|
public HandlerList getHandlers() {
|
|
return handlers;
|
|
}
|
|
|
|
public Entity getAffected() {
|
|
return affected;
|
|
}
|
|
|
|
public void setAffected(Entity affected) {
|
|
this.affected = affected;
|
|
}
|
|
|
|
public Vector getVelocity() {
|
|
return velocity;
|
|
}
|
|
|
|
public void setVelocity(Vector velocity) {
|
|
this.velocity = velocity;
|
|
}
|
|
|
|
public Ability getAbility() {
|
|
return ability;
|
|
}
|
|
|
|
public static HandlerList getHandlerList() {
|
|
return handlers;
|
|
}
|
|
}
|