mirror of
https://github.com/TotalFreedomMC/TF-ProjectKorra.git
synced 2024-05-15 17:31:26 +00:00
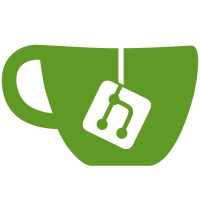
## Additions * Adds a built-in bending board sidebar to visualize bound abilities and cooldowns. * The board respects worlds where bending is disabled. * Players can use the command `/pk board` to toggle the visibility of their board. * Includes an API that community developers can use in BendingBoardManager. * Adds the `"Properties.BendingBoard"` config option to enable or disable the board server. * Adds language file configuration options to control BendingBoard visuals. * `"Board.Title"` * Controls the title at the top of the board. * Supports the standard Minecraft color codes. * `"Board.SelectionPrefix"` * Controls the prefix shown corresponding to your current hot bar slot. * Supports the standard Minecraft color codes. * `"Board.EmptySlot"` * Controls what is shown for empty slots. * Supports the standard Minecraft color codes. * `{slot_number}` can be used as a placeholder for the slot number. * `"Board.MiscSeparator"` * Controls the separation between hot bar binds and temporary cooldowns such as Combos. * Supports the standard Minecraft color codes. * Adds support for KingdomsX version 1.10.19.1 * Adds ability permission check to passive abilities. They should now respect their `bending.ability.<ability name>` permissions. * Adds `AbilityVelocityAffectEntityEvent` * A cancellable event that will fire whenever an ability would alter the velocity of an entity. * Adds the `Abilities.Earth.EarthSmash.Shoot.CollisionRadius` configuration option * Sets the collision radius of shot EarthSmash. ## Fixes * Fixes FireBlast going through liquids. * Fixes duplication involving waterlogged containers. * Fixes being able to not enter the name of a Preset when using the `/pk preset create <name>` command. * Fixes getDayFactor() not being applied correctly and occasionally producing the wrong value. * Fixes a rounding issue with some Fire ability damage configuration options. * Fixes an error when attempting to start EarthGrab. * Fixes PhaseChange error when melting snow. * Fixes a memory/process leak in how cooldowns were removed. * A player's cooldowns could only be removed when they were online. If a player's cooldown expired while they weren't online, their cooldown would attempt to revert every tick until the player rejoined. This has been resolved so cooldowns can revert while a player is offline. * A side effect of this fix is that it is now possible for `PlayerCooldownChangeEvents` to fire while their corresponding Player is offline. * Fixes an issue with `MultiAbilityManager#hasMultiAbilityBound` where it would return true if any MultiAbility is bound, not if the specified MultiAbility was bound. ## Misc Changes * Updates Towny version to 0.96.2.0 * DensityShift sand blocks can now be used as a bendable source. * Changes AvatarState so that its cooldown is applied when the ability ends instead of when it starts. * Changes togglable abilities such as AvatarState, Illumination, and TremorSense to visually show when they are enabled in the BendingBoard and on BendingPreview in the same way as the ChiBlocking Stances. * Updated the text of some ability descriptions and instructions. * Adds new cache to PhaseChange to greatly improve the performance of water/ice updates.
122 lines
5.4 KiB
Java
122 lines
5.4 KiB
Java
package com.projectkorra.projectkorra.command;
|
|
|
|
import java.util.ArrayList;
|
|
import java.util.Arrays;
|
|
import java.util.HashSet;
|
|
import java.util.List;
|
|
import java.util.Set;
|
|
|
|
import org.bukkit.command.CommandExecutor;
|
|
import org.bukkit.command.PluginCommand;
|
|
|
|
import com.projectkorra.projectkorra.ProjectKorra;
|
|
|
|
public class Commands {
|
|
|
|
private final ProjectKorra plugin;
|
|
|
|
public static Set<String> invincible = new HashSet<String>();
|
|
public static boolean debugEnabled = false;
|
|
public static boolean isToggledForAll = false;
|
|
|
|
public Commands(final ProjectKorra plugin) {
|
|
this.plugin = plugin;
|
|
debugEnabled = ProjectKorra.plugin.getConfig().getBoolean("debug");
|
|
this.init();
|
|
}
|
|
|
|
// Element Aliases.
|
|
public static String[] airaliases = { "air", "a", "airbending", "airbender" };
|
|
public static String[] chialiases = { "chi", "c", "chiblocking", "chiblocker" };
|
|
public static String[] earthaliases = { "earth", "e", "earthbending", "earthbender" };
|
|
public static String[] firealiases = { "fire", "f", "firebending", "firebender" };
|
|
public static String[] wateraliases = { "water", "w", "waterbending", "waterbender" };
|
|
public static String[] elementaliases = { "air", "a", "airbending", "airbender", "chi", "c", "chiblocking", "chiblocker", "earth", "e", "earthbending", "earthbender", "fire", "f", "firebending", "firebender", "water", "w", "waterbending", "waterbender" };
|
|
public static String[] avataraliases = { "avatar", "av", "avy", "aang", "korra" };
|
|
|
|
// Combo Aliases.
|
|
public static String[] aircomboaliases = { "aircombo", "ac", "aircombos", "airbendingcombos" };
|
|
public static String[] chicomboaliases = { "chicombo", "cc", "chicombos", "chiblockingcombos", "chiblockercombos" };
|
|
public static String[] earthcomboaliases = { "earthcombo", "ec", "earthcombos", "earthbendingcombos" };
|
|
public static String[] firecomboaliases = { "firecombo", "fc", "firecombos", "firebendingcombos" };
|
|
public static String[] watercomboaliases = { "watercombo", "wc", "watercombos", "waterbendingcombos" };
|
|
|
|
public static String[] comboaliases = { "aircombo", "ac", "aircombos", "airbendingcombos", "chicombo", "cc", "chicombos", "chiblockingcombos", "chiblockercombos", "earthcombo", "ec", "earthcombos", "earthbendingcombos", "firecombo", "fc", "firecombos", "firebendingcombos", "watercombo", "wc", "watercombos", "waterbendingcombos" };
|
|
|
|
// Passive Aliases.
|
|
public static String[] passivealiases = { "airpassive", "ap", "airpassives", "airbendingpassives", "chipassive", "cp", "chipassives", "chiblockingpassives", "chiblockerpassives", "earthpassive", "ep", "earthpassives", "earthbendingpassives", "firepassive", "fp", "firepassives", "firebendingpassives", "waterpassive", "wp", "waterpassives", "waterbendingpassives" };
|
|
|
|
// Subelement Aliases.
|
|
public static String[] subelementaliases = { "flight", "fl", "spiritualprojection", "sp", "spiritual", "bloodbending", "bb", "healing", "heal", "icebending", "ice", "ib", "plantbending", "plant", "metalbending", "mb", "metal", "lavabending", "lb", "lava", "sandbending", "sb", "sand", "combustionbending", "combustion", "cb", "lightningbending", "lightning" };
|
|
|
|
// Air.
|
|
public static String[] flightaliases = { "flight", "fl" };
|
|
public static String[] spiritualprojectionaliases = { "spiritualprojection", "sp", "spiritual" };
|
|
|
|
// Water.
|
|
public static String[] bloodaliases = { "bloodbending", "bb" };
|
|
public static String[] healingaliases = { "healing", "heal" };
|
|
public static String[] icealiases = { "icebending", "ice", "ib" };
|
|
public static String[] plantaliases = { "plantbending", "plant" };
|
|
|
|
// Earth.
|
|
public static String[] metalbendingaliases = { "metalbending", "mb", "metal" };
|
|
public static String[] lavabendingaliases = { "lavabending", "lb", "lava" };
|
|
public static String[] sandbendingaliases = { "sandbending", "sb", "sand" };
|
|
|
|
// Fire.
|
|
public static String[] combustionaliases = { "combustionbending", "combustion", "cb" };
|
|
public static String[] lightningaliases = { "lightningbending", "lightning" };
|
|
|
|
// Miscellaneous.
|
|
public static String[] commandaliases = { "b", "pk", "projectkorra", "bending", "mtla", "tla", "korra", "bend" };
|
|
|
|
private void init() {
|
|
final PluginCommand projectkorra = this.plugin.getCommand("projectkorra");
|
|
|
|
/**
|
|
* Set of all of the Classes which extend Command
|
|
*/
|
|
new AddCommand();
|
|
new BindCommand();
|
|
new CheckCommand();
|
|
new BoardCommand();
|
|
new ChooseCommand();
|
|
new ClearCommand();
|
|
new CopyCommand();
|
|
new DebugCommand();
|
|
new DisplayCommand();
|
|
new HelpCommand();
|
|
new InvincibleCommand();
|
|
new PermaremoveCommand();
|
|
new PresetCommand();
|
|
new ReloadCommand();
|
|
new RemoveCommand();
|
|
new StatsCommand();
|
|
new ToggleCommand();
|
|
new VersionCommand();
|
|
new WhoCommand();
|
|
|
|
final CommandExecutor exe = (s, c, label, args) -> {
|
|
if (Arrays.asList(commandaliases).contains(label.toLowerCase())) {
|
|
if (args.length > 0) {
|
|
final List<String> sendingArgs = Arrays.asList(args).subList(1, args.length);
|
|
for (final PKCommand command : PKCommand.instances.values()) {
|
|
if (Arrays.asList(command.getAliases()).contains(args[0].toLowerCase())) {
|
|
command.execute(s, sendingArgs);
|
|
return true;
|
|
}
|
|
}
|
|
}
|
|
|
|
PKCommand.instances.get("help").execute(s, new ArrayList<String>());
|
|
return true;
|
|
}
|
|
|
|
return false;
|
|
};
|
|
projectkorra.setExecutor(exe);
|
|
projectkorra.setTabCompleter(new BendingTabComplete());
|
|
}
|
|
}
|