mirror of
https://github.com/TotalFreedomMC/TF-ProjectKorra.git
synced 2024-05-15 17:31:26 +00:00
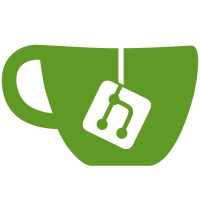
For Spigot 1.14.4+ ## General Gameplay ### Additions - Added default Suffocate cooldown: 6.5 seconds - Added kinetic damage tracker to AirSweep - Added self and others push strength options for AirSuction - Added config option for multiple blocks to be changed per EarthTunnel run, allowing for much faster earth tunnels - Added config to ignore ores completely for EarthTunnel (good for mining) - Added AllowSnow config option for IceBlast - Added AvatarStateCooldown config options for IceBlast and IceBullet - Added config option for ice trapping abilities to not place blocks in players head or feet ### Fixes - Fixed preset command not allowing page numbers higher than 1 - Fixed Catapult and MetalClips not respecting /b invincible - Fixed Charged FireBlast radius and cooldown - Fixed Suffocate being usable through walls - Fixed FireBlast ignoring particle radius options - Fixed FireBurst fragmenting (not looking like a single burst) - Fixed AirSweep knockback to be more consistent (lessened friction) - Fixed AirBlast knockback using wrong push options - Fixed EarthSmash using nonexistent AvatarState options - Fixed error when switching worlds with PhaseChange active - Fixed server crash when hitting falling blocks with high-velocity abilities - Fixed server crash when using EarthGrab over the void - Fixed EarthTunnel not using configurable revert time - Fixed EarthPillars persisting when no entities were around ### Changes - Improved pathing for EarthBlast; works more consistently and can be used from ceilings - Improved aiming for EarthBlast and IceBlast - Changed AirSwipe and AirSweep to originate from the player’s main hand - Changed AirBlast knockback calculations; made the push options affect knockback more directly - Changed EarthTunnel to use tempblocks properly - Moved core combo instructions to config ### Removals - Removed being able to use Lightning while using FireJet - Removed jukeboxes being tempblocks - Removed bending.command.import permission from plugin.yml ## API ### Additions - Added GeneralMethods#getMainHandLocation(Player) - Added GeneralMethods#getClosestEntity(Location, double) - Added GeneralMethods#getClosestLivingEntity(Location, double) - Added "large" abilities collision with each other - Added specific timings for hot spots in the code that should help server owners diagnose lag from PK + player move event + physics event + bending manager - Created local folder repo and update pom for local jar files, this is necessary to use the maven shade plugin. - Added check for concrete powder in move earth - Added PlaceholderAPI support (hopefully more to come) + %projectkorra_slot<1-9>% ability on slot <1-9> + %projectkorra_element% element of the player + %projectkorra_elementcolor% color of the player’s element + %projectkorra_elements% elements the player has - Added "bending" WorldGuard flag. + Used for allowing or denying bending in worldguard regions. Will fall back to the old method of the build flag if the custom flag fails to register or isn't set ### Fixes - Fixed calls to CoreAbility#isEnabled() causing NullPointerExceptions when a CoreAbility is not enabled ### Changes - Changed CoreAbility#getAbility(..) Javadocs to state when it returns null - Formatted codebase - Changed GeneralMethods#getEntitiesAroundPoint() use spigot method for entities around point, should be more optimized - Optimizations to water and air spout to not continually set flying - Optimized revertchecker to not use block.getChunk; this will load the chunk on the server and instead use hashmap of coords - Optimized revertchecker to use paperlib to allow servers running paperspigot to load the chunk for a revert block async - Optimized tempblock revert to load chunk async before updating stateOptimize move event to ignore head movements without directional movement - Optimized physics event to check for air prior to checking for tempblocks - Optimized tempblock set/revert to not apply physics for blocks that don't emit light - Optimize isWater to check for actual water blocks first - Optimize PhaseChange list check, hot spot due to being called in physics event ### Removals - Removed BindChangeEvent; never called or used - Removed HorizontalVelocityTracker that has lasted over 30 seconds, also don't create a tracker for non-living entities
303 lines
8.5 KiB
Java
303 lines
8.5 KiB
Java
package com.projectkorra.projectkorra.airbending.combo;
|
|
|
|
import java.util.ArrayList;
|
|
|
|
import org.bukkit.Location;
|
|
import org.bukkit.entity.Entity;
|
|
import org.bukkit.entity.Player;
|
|
import org.bukkit.scheduler.BukkitRunnable;
|
|
import org.bukkit.util.Vector;
|
|
|
|
import com.projectkorra.projectkorra.GeneralMethods;
|
|
import com.projectkorra.projectkorra.ProjectKorra;
|
|
import com.projectkorra.projectkorra.ability.AirAbility;
|
|
import com.projectkorra.projectkorra.ability.ComboAbility;
|
|
import com.projectkorra.projectkorra.ability.util.ComboManager.AbilityInformation;
|
|
import com.projectkorra.projectkorra.attribute.Attribute;
|
|
import com.projectkorra.projectkorra.command.Commands;
|
|
import com.projectkorra.projectkorra.util.ClickType;
|
|
|
|
public class AirStream extends AirAbility implements ComboAbility {
|
|
|
|
@Attribute(Attribute.COOLDOWN)
|
|
private long cooldown;
|
|
private long time;
|
|
@Attribute(Attribute.SPEED)
|
|
private double speed;
|
|
@Attribute(Attribute.RANGE)
|
|
private double range;
|
|
@Attribute("EntityCarry" + Attribute.HEIGHT)
|
|
private double airStreamMaxEntityHeight;
|
|
@Attribute("EntityCarry" + Attribute.DURATION)
|
|
private double airStreamEntityCarryDuration;
|
|
private Location origin;
|
|
private Location currentLoc;
|
|
private Location destination;
|
|
private Vector direction;
|
|
private ArrayList<Entity> affectedEntities;
|
|
private ArrayList<BukkitRunnable> tasks;
|
|
|
|
public AirStream(final Player player) {
|
|
super(player);
|
|
|
|
this.affectedEntities = new ArrayList<>();
|
|
this.tasks = new ArrayList<>();
|
|
|
|
if (!this.bPlayer.canBendIgnoreBindsCooldowns(this)) {
|
|
return;
|
|
}
|
|
|
|
if (this.bPlayer.isOnCooldown(this)) {
|
|
return;
|
|
}
|
|
|
|
this.range = getConfig().getDouble("Abilities.Air.AirStream.Range");
|
|
this.speed = getConfig().getDouble("Abilities.Air.AirStream.Speed");
|
|
this.cooldown = getConfig().getLong("Abilities.Air.AirStream.Cooldown");
|
|
this.airStreamMaxEntityHeight = getConfig().getDouble("Abilities.Air.AirStream.EntityCarry.Height");
|
|
this.airStreamEntityCarryDuration = getConfig().getLong("Abilities.Air.AirStream.EntityCarry.Duration");
|
|
|
|
if (this.bPlayer.isAvatarState()) {
|
|
this.cooldown = 0;
|
|
this.range = getConfig().getDouble("Abilities.Avatar.AvatarState.Air.AirStream.Range");
|
|
this.airStreamMaxEntityHeight = getConfig().getDouble("Abilities.Avatar.AvatarState.Air.AirStream.EntityCarry.Height");
|
|
this.airStreamEntityCarryDuration = getConfig().getDouble("Abilities.Avatar.AvatarState.Air.AirStream.EntityCarry.Duration");
|
|
}
|
|
|
|
this.bPlayer.addCooldown(this);
|
|
this.start();
|
|
}
|
|
|
|
@Override
|
|
public String getName() {
|
|
return "AirStream";
|
|
}
|
|
|
|
@Override
|
|
public boolean isCollidable() {
|
|
return true;
|
|
}
|
|
|
|
@Override
|
|
public void progress() {
|
|
if (this.player.isDead() || !this.player.isOnline()) {
|
|
this.remove();
|
|
return;
|
|
} else if (this.currentLoc != null && GeneralMethods.isRegionProtectedFromBuild(this, this.currentLoc)) {
|
|
this.remove();
|
|
return;
|
|
}
|
|
|
|
if (this.destination == null) {
|
|
this.origin = this.player.getEyeLocation();
|
|
this.currentLoc = this.origin.clone();
|
|
}
|
|
final Entity target = GeneralMethods.getTargetedEntity(this.player, this.range);
|
|
|
|
if (target != null && target.getLocation().distanceSquared(this.currentLoc) > 49) {
|
|
this.destination = target.getLocation();
|
|
} else {
|
|
this.destination = GeneralMethods.getTargetedLocation(this.player, this.range, getTransparentMaterials());
|
|
}
|
|
|
|
if (GeneralMethods.locationEqualsIgnoreDirection(this.currentLoc, this.destination)) {
|
|
this.remove();
|
|
return;
|
|
}
|
|
|
|
this.direction = GeneralMethods.getDirection(this.currentLoc, this.destination).normalize();
|
|
this.currentLoc.add(this.direction.clone().multiply(this.speed));
|
|
|
|
if (this.player.getWorld() != this.currentLoc.getWorld()) {
|
|
this.remove();
|
|
return;
|
|
} else if (!this.player.isSneaking()) {
|
|
this.remove();
|
|
return;
|
|
} else if (this.player.getWorld().equals(this.currentLoc.getWorld()) && Math.abs(this.player.getLocation().distanceSquared(this.currentLoc)) > this.range * this.range) {
|
|
this.remove();
|
|
return;
|
|
} else if (this.affectedEntities.size() > 0 && System.currentTimeMillis() - this.time >= this.airStreamEntityCarryDuration) {
|
|
this.remove();
|
|
return;
|
|
} else if (!this.isTransparent(this.currentLoc.getBlock())) {
|
|
this.remove();
|
|
return;
|
|
} else if (this.currentLoc.getY() - this.origin.getY() > this.airStreamMaxEntityHeight) {
|
|
this.remove();
|
|
return;
|
|
} else if (GeneralMethods.isRegionProtectedFromBuild(this, this.currentLoc)) {
|
|
this.remove();
|
|
return;
|
|
} else if (!this.isTransparent(this.currentLoc.getBlock())) {
|
|
this.currentLoc.subtract(this.direction.clone().multiply(this.speed));
|
|
}
|
|
|
|
for (int i = 0; i < 10; i++) {
|
|
final BukkitRunnable br = new BukkitRunnable() {
|
|
final Location loc = AirStream.this.currentLoc.clone();
|
|
final Vector dir = AirStream.this.direction.clone();
|
|
|
|
@Override
|
|
public void run() {
|
|
for (int angle = -180; angle <= 180; angle += 45) {
|
|
final Vector orthog = GeneralMethods.getOrthogonalVector(this.dir.clone(), angle, 0.5);
|
|
playAirbendingParticles(this.loc.clone().add(orthog), 1, 0F, 0F, 0F);
|
|
}
|
|
}
|
|
};
|
|
br.runTaskLater(ProjectKorra.plugin, i * 2);
|
|
this.tasks.add(br);
|
|
}
|
|
|
|
for (final Entity entity : GeneralMethods.getEntitiesAroundPoint(this.currentLoc, 2.8)) {
|
|
if (this.affectedEntities.size() == 0) {
|
|
// Set the timer to remove the ability.
|
|
this.time = System.currentTimeMillis();
|
|
}
|
|
if (!entity.equals(this.player) && !this.affectedEntities.contains(entity)) {
|
|
this.affectedEntities.add(entity);
|
|
}
|
|
}
|
|
|
|
for (final Entity entity : this.affectedEntities) {
|
|
if (GeneralMethods.isRegionProtectedFromBuild(this, entity.getLocation()) || ((entity instanceof Player) && Commands.invincible.contains(((Player) entity).getName()))) {
|
|
continue;
|
|
}
|
|
final Vector force = GeneralMethods.getDirection(entity.getLocation(), this.currentLoc);
|
|
entity.setVelocity(force.clone().normalize().multiply(this.speed));
|
|
entity.setFallDistance(0F);
|
|
}
|
|
}
|
|
|
|
@Override
|
|
public void remove() {
|
|
super.remove();
|
|
for (final BukkitRunnable task : this.tasks) {
|
|
task.cancel();
|
|
}
|
|
}
|
|
|
|
@Override
|
|
public boolean isSneakAbility() {
|
|
return true;
|
|
}
|
|
|
|
@Override
|
|
public boolean isHarmlessAbility() {
|
|
return false;
|
|
}
|
|
|
|
@Override
|
|
public long getCooldown() {
|
|
return this.cooldown;
|
|
}
|
|
|
|
@Override
|
|
public Location getLocation() {
|
|
return this.currentLoc;
|
|
}
|
|
|
|
@Override
|
|
public Object createNewComboInstance(final Player player) {
|
|
return new AirStream(player);
|
|
}
|
|
|
|
@Override
|
|
public ArrayList<AbilityInformation> getCombination() {
|
|
final ArrayList<AbilityInformation> airStream = new ArrayList<>();
|
|
airStream.add(new AbilityInformation("AirShield", ClickType.SHIFT_DOWN));
|
|
airStream.add(new AbilityInformation("AirSuction", ClickType.LEFT_CLICK));
|
|
airStream.add(new AbilityInformation("AirBlast", ClickType.LEFT_CLICK));
|
|
return airStream;
|
|
}
|
|
|
|
public Location getOrigin() {
|
|
return this.origin;
|
|
}
|
|
|
|
public void setOrigin(final Location origin) {
|
|
this.origin = origin;
|
|
}
|
|
|
|
public Location getCurrentLoc() {
|
|
return this.currentLoc;
|
|
}
|
|
|
|
public void setCurrentLoc(final Location currentLoc) {
|
|
this.currentLoc = currentLoc;
|
|
}
|
|
|
|
public Location getDestination() {
|
|
return this.destination;
|
|
}
|
|
|
|
public void setDestination(final Location destination) {
|
|
this.destination = destination;
|
|
}
|
|
|
|
public Vector getDirection() {
|
|
return this.direction;
|
|
}
|
|
|
|
public void setDirection(final Vector direction) {
|
|
this.direction = direction;
|
|
}
|
|
|
|
public long getTime() {
|
|
return this.time;
|
|
}
|
|
|
|
public void setTime(final long time) {
|
|
this.time = time;
|
|
}
|
|
|
|
public double getSpeed() {
|
|
return this.speed;
|
|
}
|
|
|
|
public void setSpeed(final double speed) {
|
|
this.speed = speed;
|
|
}
|
|
|
|
public double getRange() {
|
|
return this.range;
|
|
}
|
|
|
|
public void setRange(final double range) {
|
|
this.range = range;
|
|
}
|
|
|
|
public double getAirStreamMaxEntityHeight() {
|
|
return this.airStreamMaxEntityHeight;
|
|
}
|
|
|
|
public void setAirStreamMaxEntityHeight(final double airStreamMaxEntityHeight) {
|
|
this.airStreamMaxEntityHeight = airStreamMaxEntityHeight;
|
|
}
|
|
|
|
public double getAirStreamEntityCarryDuration() {
|
|
return this.airStreamEntityCarryDuration;
|
|
}
|
|
|
|
public void setAirStreamEntityCarryDuration(final double airStreamEntityCarryDuration) {
|
|
this.airStreamEntityCarryDuration = airStreamEntityCarryDuration;
|
|
}
|
|
|
|
public ArrayList<Entity> getAffectedEntities() {
|
|
return this.affectedEntities;
|
|
}
|
|
|
|
public ArrayList<BukkitRunnable> getTasks() {
|
|
return this.tasks;
|
|
}
|
|
|
|
public void setTasks(final ArrayList<BukkitRunnable> tasks) {
|
|
this.tasks = tasks;
|
|
}
|
|
|
|
public void setCooldown(final long cooldown) {
|
|
this.cooldown = cooldown;
|
|
}
|
|
}
|