mirror of
https://github.com/TotalFreedomMC/TF-ProjectKorra.git
synced 2024-05-15 17:31:26 +00:00
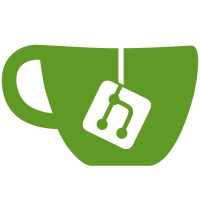
For Spigot 1.14.4+ ## General Gameplay ### Additions - Added default Suffocate cooldown: 6.5 seconds - Added kinetic damage tracker to AirSweep - Added self and others push strength options for AirSuction - Added config option for multiple blocks to be changed per EarthTunnel run, allowing for much faster earth tunnels - Added config to ignore ores completely for EarthTunnel (good for mining) - Added AllowSnow config option for IceBlast - Added AvatarStateCooldown config options for IceBlast and IceBullet - Added config option for ice trapping abilities to not place blocks in players head or feet ### Fixes - Fixed preset command not allowing page numbers higher than 1 - Fixed Catapult and MetalClips not respecting /b invincible - Fixed Charged FireBlast radius and cooldown - Fixed Suffocate being usable through walls - Fixed FireBlast ignoring particle radius options - Fixed FireBurst fragmenting (not looking like a single burst) - Fixed AirSweep knockback to be more consistent (lessened friction) - Fixed AirBlast knockback using wrong push options - Fixed EarthSmash using nonexistent AvatarState options - Fixed error when switching worlds with PhaseChange active - Fixed server crash when hitting falling blocks with high-velocity abilities - Fixed server crash when using EarthGrab over the void - Fixed EarthTunnel not using configurable revert time - Fixed EarthPillars persisting when no entities were around ### Changes - Improved pathing for EarthBlast; works more consistently and can be used from ceilings - Improved aiming for EarthBlast and IceBlast - Changed AirSwipe and AirSweep to originate from the player’s main hand - Changed AirBlast knockback calculations; made the push options affect knockback more directly - Changed EarthTunnel to use tempblocks properly - Moved core combo instructions to config ### Removals - Removed being able to use Lightning while using FireJet - Removed jukeboxes being tempblocks - Removed bending.command.import permission from plugin.yml ## API ### Additions - Added GeneralMethods#getMainHandLocation(Player) - Added GeneralMethods#getClosestEntity(Location, double) - Added GeneralMethods#getClosestLivingEntity(Location, double) - Added "large" abilities collision with each other - Added specific timings for hot spots in the code that should help server owners diagnose lag from PK + player move event + physics event + bending manager - Created local folder repo and update pom for local jar files, this is necessary to use the maven shade plugin. - Added check for concrete powder in move earth - Added PlaceholderAPI support (hopefully more to come) + %projectkorra_slot<1-9>% ability on slot <1-9> + %projectkorra_element% element of the player + %projectkorra_elementcolor% color of the player’s element + %projectkorra_elements% elements the player has - Added "bending" WorldGuard flag. + Used for allowing or denying bending in worldguard regions. Will fall back to the old method of the build flag if the custom flag fails to register or isn't set ### Fixes - Fixed calls to CoreAbility#isEnabled() causing NullPointerExceptions when a CoreAbility is not enabled ### Changes - Changed CoreAbility#getAbility(..) Javadocs to state when it returns null - Formatted codebase - Changed GeneralMethods#getEntitiesAroundPoint() use spigot method for entities around point, should be more optimized - Optimizations to water and air spout to not continually set flying - Optimized revertchecker to not use block.getChunk; this will load the chunk on the server and instead use hashmap of coords - Optimized revertchecker to use paperlib to allow servers running paperspigot to load the chunk for a revert block async - Optimized tempblock revert to load chunk async before updating stateOptimize move event to ignore head movements without directional movement - Optimized physics event to check for air prior to checking for tempblocks - Optimized tempblock set/revert to not apply physics for blocks that don't emit light - Optimize isWater to check for actual water blocks first - Optimize PhaseChange list check, hot spot due to being called in physics event ### Removals - Removed BindChangeEvent; never called or used - Removed HorizontalVelocityTracker that has lasted over 30 seconds, also don't create a tracker for non-living entities
22 lines
512 B
Java
22 lines
512 B
Java
package com.projectkorra.projectkorra.ability;
|
|
|
|
public interface PassiveAbility {
|
|
|
|
/**
|
|
* This is a check to see if the passive requires some form of activation,
|
|
* such as sneaking, clicking, etc. <br>
|
|
* <b>If false, the passive should not call start!</b>
|
|
*
|
|
* @return false if this passive should always be on
|
|
*/
|
|
public boolean isInstantiable();
|
|
|
|
/**
|
|
* This is used if the passive should progress
|
|
*
|
|
* @return false if progress() shouldn't be called;
|
|
*/
|
|
public boolean isProgressable();
|
|
|
|
}
|