mirror of
https://github.com/TotalFreedomMC/TF-ProjectKorra.git
synced 2024-05-16 01:41:22 +00:00
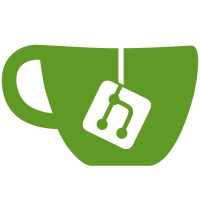
## Additions * Adds a built-in bending board sidebar to visualize bound abilities and cooldowns. * The board respects worlds where bending is disabled. * Players can use the command `/pk board` to toggle the visibility of their board. * Includes an API that community developers can use in BendingBoardManager. * Adds the `"Properties.BendingBoard"` config option to enable or disable the board server. * Adds language file configuration options to control BendingBoard visuals. * `"Board.Title"` * Controls the title at the top of the board. * Supports the standard Minecraft color codes. * `"Board.SelectionPrefix"` * Controls the prefix shown corresponding to your current hot bar slot. * Supports the standard Minecraft color codes. * `"Board.EmptySlot"` * Controls what is shown for empty slots. * Supports the standard Minecraft color codes. * `{slot_number}` can be used as a placeholder for the slot number. * `"Board.MiscSeparator"` * Controls the separation between hot bar binds and temporary cooldowns such as Combos. * Supports the standard Minecraft color codes. * Adds support for KingdomsX version 1.10.19.1 * Adds ability permission check to passive abilities. They should now respect their `bending.ability.<ability name>` permissions. * Adds `AbilityVelocityAffectEntityEvent` * A cancellable event that will fire whenever an ability would alter the velocity of an entity. * Adds the `Abilities.Earth.EarthSmash.Shoot.CollisionRadius` configuration option * Sets the collision radius of shot EarthSmash. ## Fixes * Fixes FireBlast going through liquids. * Fixes duplication involving waterlogged containers. * Fixes being able to not enter the name of a Preset when using the `/pk preset create <name>` command. * Fixes getDayFactor() not being applied correctly and occasionally producing the wrong value. * Fixes a rounding issue with some Fire ability damage configuration options. * Fixes an error when attempting to start EarthGrab. * Fixes PhaseChange error when melting snow. * Fixes a memory/process leak in how cooldowns were removed. * A player's cooldowns could only be removed when they were online. If a player's cooldown expired while they weren't online, their cooldown would attempt to revert every tick until the player rejoined. This has been resolved so cooldowns can revert while a player is offline. * A side effect of this fix is that it is now possible for `PlayerCooldownChangeEvents` to fire while their corresponding Player is offline. * Fixes an issue with `MultiAbilityManager#hasMultiAbilityBound` where it would return true if any MultiAbility is bound, not if the specified MultiAbility was bound. ## Misc Changes * Updates Towny version to 0.96.2.0 * DensityShift sand blocks can now be used as a bendable source. * Changes AvatarState so that its cooldown is applied when the ability ends instead of when it starts. * Changes togglable abilities such as AvatarState, Illumination, and TremorSense to visually show when they are enabled in the BendingBoard and on BendingPreview in the same way as the ChiBlocking Stances. * Updated the text of some ability descriptions and instructions. * Adds new cache to PhaseChange to greatly improve the performance of water/ice updates.
277 lines
6.7 KiB
Java
277 lines
6.7 KiB
Java
package com.projectkorra.projectkorra.earthbending;
|
|
|
|
import java.util.ArrayList;
|
|
import java.util.List;
|
|
import java.util.concurrent.ConcurrentHashMap;
|
|
|
|
import org.bukkit.Location;
|
|
import org.bukkit.block.Block;
|
|
import org.bukkit.entity.Player;
|
|
import org.bukkit.util.Vector;
|
|
|
|
import com.projectkorra.projectkorra.ability.EarthAbility;
|
|
import com.projectkorra.projectkorra.attribute.Attribute;
|
|
import com.projectkorra.projectkorra.configuration.ConfigManager;
|
|
import com.projectkorra.projectkorra.util.BlockSource;
|
|
import com.projectkorra.projectkorra.util.ClickType;
|
|
import com.projectkorra.projectkorra.util.TempBlock;
|
|
|
|
public class RaiseEarth extends EarthAbility {
|
|
|
|
private int distance;
|
|
@Attribute(Attribute.HEIGHT)
|
|
private int height;
|
|
private long time;
|
|
private long interval;
|
|
@Attribute(Attribute.COOLDOWN)
|
|
private long cooldown;
|
|
@Attribute(Attribute.SELECT_RANGE)
|
|
private double selectRange;
|
|
@Attribute(Attribute.SPEED)
|
|
private double speed;
|
|
private Block block;
|
|
private Vector direction;
|
|
private Location origin;
|
|
private Location location;
|
|
private ConcurrentHashMap<Block, Block> affectedBlocks;
|
|
|
|
public RaiseEarth(final Player player) {
|
|
super(player);
|
|
this.setFields();
|
|
|
|
if (!this.bPlayer.canBend(this) || this.bPlayer.isOnCooldown("RaiseEarthPillar")) {
|
|
return;
|
|
}
|
|
|
|
try {
|
|
if (this.bPlayer.isAvatarState()) {
|
|
this.height = getConfig().getInt("Abilities.Avatar.AvatarState.Earth.RaiseEarth.Column.Height");
|
|
}
|
|
this.block = BlockSource.getEarthSourceBlock(player, this.selectRange, ClickType.LEFT_CLICK);
|
|
if (this.block == null) {
|
|
return;
|
|
}
|
|
|
|
this.origin = this.block.getLocation();
|
|
this.location = this.origin.clone();
|
|
this.distance = this.getEarthbendableBlocksLength(this.block, this.direction.clone().multiply(-1), this.height);
|
|
} catch (final IllegalStateException e) {
|
|
return;
|
|
}
|
|
|
|
this.loadAffectedBlocks();
|
|
|
|
if (this.distance != 0 && this.canInstantiate()) {
|
|
this.bPlayer.addCooldown("RaiseEarthPillar", this.cooldown);
|
|
this.time = System.currentTimeMillis() - this.interval;
|
|
this.start();
|
|
}
|
|
}
|
|
|
|
public RaiseEarth(final Player player, final Location origin) {
|
|
this(player, origin, ConfigManager.getConfig().getInt("Abilities.Earth.RaiseEarth.Column.Height"));
|
|
}
|
|
|
|
public RaiseEarth(final Player player, final Location origin, final int height) {
|
|
super(player);
|
|
this.setFields();
|
|
|
|
this.height = height;
|
|
this.origin = origin;
|
|
this.location = origin.clone();
|
|
this.block = this.location.getBlock();
|
|
this.distance = this.getEarthbendableBlocksLength(this.block, this.direction.clone().multiply(-1), height);
|
|
|
|
this.loadAffectedBlocks();
|
|
|
|
if (this.distance != 0 && this.canInstantiate()) {
|
|
this.time = System.currentTimeMillis() - this.interval;
|
|
this.start();
|
|
}
|
|
}
|
|
|
|
private void setFields() {
|
|
this.speed = getConfig().getDouble("Abilities.Earth.RaiseEarth.Speed");
|
|
this.height = getConfig().getInt("Abilities.Earth.RaiseEarth.Column.Height");
|
|
this.selectRange = getConfig().getDouble("Abilities.Earth.RaiseEarth.Column.SelectRange");
|
|
this.cooldown = getConfig().getLong("Abilities.Earth.RaiseEarth.Column.Cooldown");
|
|
this.direction = new Vector(0, 1, 0);
|
|
this.interval = (long) (1000.0 / this.speed);
|
|
this.affectedBlocks = new ConcurrentHashMap<>();
|
|
}
|
|
|
|
private boolean canInstantiate() {
|
|
for (final Block block : this.affectedBlocks.keySet()) {
|
|
if (!this.isEarthbendable(block) || (TempBlock.isTempBlock(block) && !EarthAbility.isBendableEarthTempBlock(block))) {
|
|
return false;
|
|
}
|
|
}
|
|
return true;
|
|
}
|
|
|
|
private void loadAffectedBlocks() {
|
|
this.affectedBlocks.clear();
|
|
Block thisBlock;
|
|
for (int i = 0; i <= this.distance; i++) {
|
|
thisBlock = this.block.getWorld().getBlockAt(this.location.clone().add(this.direction.clone().multiply(-i)));
|
|
this.affectedBlocks.put(thisBlock, thisBlock);
|
|
if (Collapse.blockInAllAffectedBlocks(thisBlock)) {
|
|
Collapse.revert(thisBlock);
|
|
}
|
|
}
|
|
}
|
|
|
|
@Override
|
|
public void progress() {
|
|
if (System.currentTimeMillis() - this.time >= this.interval) {
|
|
this.time = System.currentTimeMillis();
|
|
final Block block = this.location.getBlock();
|
|
this.location = this.location.add(this.direction);
|
|
if (!block.isLiquid()) {
|
|
this.moveEarth(block, this.direction, this.distance);
|
|
}
|
|
|
|
this.loadAffectedBlocks();
|
|
|
|
if (this.location.distanceSquared(this.origin) >= this.distance * this.distance) {
|
|
this.remove();
|
|
return;
|
|
}
|
|
}
|
|
}
|
|
|
|
public static boolean blockInAllAffectedBlocks(final Block block) {
|
|
for (RaiseEarth raiseEarth : getAbilities(RaiseEarth.class)) {
|
|
if (raiseEarth.affectedBlocks.contains(block)) {
|
|
return true;
|
|
}
|
|
}
|
|
return false;
|
|
}
|
|
|
|
public static void revertAffectedBlock(final Block block) {
|
|
for (final RaiseEarth raiseEarth : getAbilities(RaiseEarth.class)) {
|
|
raiseEarth.affectedBlocks.remove(block);
|
|
}
|
|
}
|
|
|
|
@Override
|
|
public String getName() {
|
|
return "RaiseEarth";
|
|
}
|
|
|
|
@Override
|
|
public Location getLocation() {
|
|
return this.location;
|
|
}
|
|
|
|
@Override
|
|
public long getCooldown() {
|
|
return this.cooldown;
|
|
}
|
|
|
|
@Override
|
|
public boolean isSneakAbility() {
|
|
return true;
|
|
}
|
|
|
|
@Override
|
|
public boolean isHarmlessAbility() {
|
|
return false;
|
|
}
|
|
|
|
@Override
|
|
public List<Location> getLocations() {
|
|
final ArrayList<Location> locations = new ArrayList<>();
|
|
for (final Block block : this.affectedBlocks.values()) {
|
|
locations.add(block.getLocation());
|
|
}
|
|
return locations;
|
|
}
|
|
|
|
public int getDistance() {
|
|
return this.distance;
|
|
}
|
|
|
|
public void setDistance(final int distance) {
|
|
this.distance = distance;
|
|
}
|
|
|
|
public int getHeight() {
|
|
return this.height;
|
|
}
|
|
|
|
public void setHeight(final int height) {
|
|
this.height = height;
|
|
}
|
|
|
|
public long getTime() {
|
|
return this.time;
|
|
}
|
|
|
|
public void setTime(final long time) {
|
|
this.time = time;
|
|
}
|
|
|
|
public long getInterval() {
|
|
return this.interval;
|
|
}
|
|
|
|
public void setInterval(final long interval) {
|
|
this.interval = interval;
|
|
}
|
|
|
|
public double getSpeed() {
|
|
return this.speed;
|
|
}
|
|
|
|
public void setSpeed(final double speed) {
|
|
this.speed = speed;
|
|
}
|
|
|
|
public Block getBlock() {
|
|
return this.block;
|
|
}
|
|
|
|
public void setBlock(final Block block) {
|
|
this.block = block;
|
|
}
|
|
|
|
public Vector getDirection() {
|
|
return this.direction;
|
|
}
|
|
|
|
public void setDirection(final Vector direction) {
|
|
this.direction = direction;
|
|
}
|
|
|
|
public Location getOrigin() {
|
|
return this.origin;
|
|
}
|
|
|
|
public void setOrigin(final Location origin) {
|
|
this.origin = origin;
|
|
}
|
|
|
|
public ConcurrentHashMap<Block, Block> getAffectedBlocks() {
|
|
return this.affectedBlocks;
|
|
}
|
|
|
|
public void setCooldown(final long cooldown) {
|
|
this.cooldown = cooldown;
|
|
}
|
|
|
|
public void setLocation(final Location location) {
|
|
this.location = location;
|
|
}
|
|
|
|
public double getSelectRange() {
|
|
return this.selectRange;
|
|
}
|
|
|
|
public void setSelectRange(final double selectRange) {
|
|
this.selectRange = selectRange;
|
|
}
|
|
|
|
}
|