mirror of
https://github.com/TotalFreedomMC/TF-ProjectKorra.git
synced 2024-05-15 17:31:26 +00:00
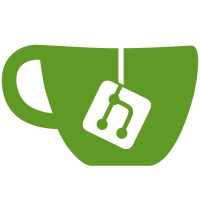
## Additions * Adds support for Minecraft 1.17! * Adds vanished player support to the `/pk who <Player>` command. * Should work with most common vanishing plugins, tested using Essentials vanish. * Affects `/pk who` and `/pk who <Player>`. * Adds new `"Abilities.Earth.EarthSmash.Lift.Knockup"` configuration option to control the velocity applied to players standing where an EarthSmash is created. * Adds new `"Abilities.Earth.EarthSmash.Lift.Range"` configuration option to control the range in which entities need to be standing in relation to where an EarthSmash is created to get the Knockup applied. ## Fixes * Fixes players sometimes "falling through" their EarthSmash if they try to create it underneath themselves. * Reduces the overall push so it feels more natural. * Expands the push radius so it can affect entities within one block of the location. * Fixes IceWave activating sometimes when PhaseChange wasn't clicked. * Fixes IceWave users getting stuck on their ice midair. * Fixes the display command showing hidden combos and passives * Fixes an error caused by adding attribute modifiers to abilities sometimes. * Fixes an error preventing the plugin from working on Minecraft 1.17. * ClassDefNotFoundError resulting from EntityHuman being moved during the 1.17 Minecraft update. We no longer rely on NMS for this area as a proper API has been introduced. As such, GeneralMethods and EarthArmor have been updated to reflect these changes.
80 lines
2.6 KiB
Java
80 lines
2.6 KiB
Java
package com.projectkorra.projectkorra.attribute;
|
|
|
|
public enum AttributeModifier {
|
|
|
|
ADDITION((oldValue, modifier) -> {
|
|
if (oldValue instanceof Double) {
|
|
return oldValue.doubleValue() + modifier.doubleValue();
|
|
} else if (oldValue instanceof Float) {
|
|
return oldValue.floatValue() + modifier.floatValue();
|
|
} else if (oldValue instanceof Long) {
|
|
return oldValue.longValue() + modifier.longValue();
|
|
} else if (oldValue instanceof Integer) {
|
|
return oldValue.intValue() + modifier.intValue();
|
|
}
|
|
return 0;
|
|
}), SUBTRACTION((oldValue, modifier) -> {
|
|
if (oldValue instanceof Double) {
|
|
return oldValue.doubleValue() - modifier.doubleValue();
|
|
} else if (oldValue instanceof Float) {
|
|
return oldValue.floatValue() - modifier.floatValue();
|
|
} else if (oldValue instanceof Long) {
|
|
return oldValue.longValue() - modifier.longValue();
|
|
} else if (oldValue instanceof Integer) {
|
|
return oldValue.intValue() - modifier.intValue();
|
|
}
|
|
return 0;
|
|
}), MULTIPLICATION((oldValue, modifier) -> {
|
|
if (oldValue instanceof Double) {
|
|
return oldValue.doubleValue() * modifier.doubleValue();
|
|
} else if (oldValue instanceof Float) {
|
|
return oldValue.floatValue() * modifier.floatValue();
|
|
} else if (oldValue instanceof Long) {
|
|
return oldValue.longValue() * modifier.longValue();
|
|
} else if (oldValue instanceof Integer) {
|
|
return oldValue.intValue() * modifier.intValue();
|
|
}
|
|
return 0;
|
|
}), DIVISION((oldValue, modifier) -> {
|
|
if (oldValue instanceof Double) {
|
|
return oldValue.doubleValue() / modifier.doubleValue();
|
|
} else if (oldValue instanceof Float) {
|
|
return oldValue.floatValue() / modifier.floatValue();
|
|
} else if (oldValue instanceof Long) {
|
|
return oldValue.longValue() / modifier.longValue();
|
|
} else if (oldValue instanceof Integer) {
|
|
return oldValue.intValue() / modifier.intValue();
|
|
}
|
|
return 0;
|
|
});
|
|
|
|
private AttributeModifierMethod modifier;
|
|
|
|
private AttributeModifier(final AttributeModifierMethod modifier) {
|
|
this.modifier = modifier;
|
|
}
|
|
|
|
public AttributeModifierMethod getModifier() {
|
|
return this.modifier;
|
|
}
|
|
|
|
public Number performModification(final Number oldValue, final Number modifier) {
|
|
if (this == DIVISION && modifier.doubleValue() == 0) {
|
|
throw new IllegalArgumentException("Attribute modifier for DIVISION cannot be zero!");
|
|
}
|
|
return this.modifier.performModification(oldValue, modifier);
|
|
}
|
|
|
|
/**
|
|
* Functional interface for modifying fields with the {@link Attribute}
|
|
* annotation
|
|
*/
|
|
@FunctionalInterface
|
|
public interface AttributeModifierMethod {
|
|
|
|
public Number performModification(Number oldValue, Number modifier);
|
|
|
|
}
|
|
|
|
}
|