mirror of
https://github.com/TotalFreedomMC/TF-ProjectKorra.git
synced 2024-05-16 01:41:22 +00:00
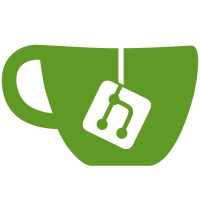
## Fixes * Fixed Combos and possibly Passives appearing in `/pk b <Ability>` auto-tabbing. * Fixed Combos not loading properly on certain servers. * Fixed issue with `PreciousStones` by updating to the latest version to resolve API change issues. * Fixed `RapidPunch` damage. * Fixed incorrect summation of chiblocking chance. * Fixed possible issue in PKListener#onPlayerInteraction() * Fixed `Earth.LavaSound`. * Fixed Chi attempting to chiblock targets with any move. * Fixed hitting an entity with `TempArmor` not ignoring armor. * Fixed `Immobilize` config path. ## Additions * Added "Contributing" section to the `README` to help guide active community members. * Added more detail to the `PULL_REQUEST_TEMPLATE` to allow for more uniform pull requests. * Added many new blocks to our ability block interaction. * Added check to combo collisions to discard dead entities. * Added functionality to allow chiblocking abilities to affect all entities. * Added exception handling to the configurable `Sound` options to prevent `IllegalArgumentExcpetions`. * Added sounds and `ActionBar` messages to being Bloodbent, Electrocuted, Immobilized, MetalClipped, and Paralyzed. (Abilities: `Bloodbending`, `Lightning`, `Immobilize`, `MetalClips`, and `Paralyze`) * Added sound and `ActionBar` message for being Chiblocked. * Added interval config option to `RapidPunch`. (time between each punch) ## API Changes * Updated to `Spigot 1.12.1`. * Confirmed to be backward compatible with `Spigot 1.12` and `Spigot 1.11.2`. * Renamed `ElementalAbility#getTransparentMaterial()` to `ElementalAbility#getTransparentMaterials()`. * Converted most `byte`/`int` dependent `Material` logic to use `Material` instead. * `ElementalAbility#getTransparentMaterialSet()` now returns a `HashSet<Material>` instead of a `HashSet<Byte>`. * `ElementalAbility#getTransparentMaterials()` and `GeneralMethods.NON_OPAQUE` now return `Material[]` instead of `Integer[]`. * `GeneralMethods#getTargetedLocation()` now takes a `varargs Material[]` instead of a `varargs Integer[]`. * Removed `ElementalAbility.TRANSPARENT_MATERIAL`. It was outdated and became irrelevent after `GeneralMethods.NON_OPAQUE` was updated. * Removed `Java 7` Travi-CI JDK check. * Updated `pom.xml` to build in `Java 8`. * Added new `MovementHandler` utility class to control entity movement. (currently only capable of stopping movement.
104 lines
3.3 KiB
Java
104 lines
3.3 KiB
Java
package com.projectkorra.projectkorra.chiblocking.passive;
|
|
|
|
import org.bukkit.Sound;
|
|
import org.bukkit.entity.Player;
|
|
import org.bukkit.scheduler.BukkitRunnable;
|
|
|
|
import com.projectkorra.projectkorra.BendingPlayer;
|
|
import com.projectkorra.projectkorra.Element;
|
|
import com.projectkorra.projectkorra.ProjectKorra;
|
|
import com.projectkorra.projectkorra.ability.ChiAbility;
|
|
import com.projectkorra.projectkorra.ability.CoreAbility;
|
|
import com.projectkorra.projectkorra.airbending.Suffocate;
|
|
import com.projectkorra.projectkorra.chiblocking.AcrobatStance;
|
|
import com.projectkorra.projectkorra.chiblocking.QuickStrike;
|
|
import com.projectkorra.projectkorra.chiblocking.SwiftKick;
|
|
import com.projectkorra.projectkorra.configuration.ConfigManager;
|
|
import com.projectkorra.projectkorra.util.ActionBar;
|
|
|
|
public class ChiPassive {
|
|
|
|
public static boolean willChiBlock(Player attacker, Player player) {
|
|
BendingPlayer bPlayer = BendingPlayer.getBendingPlayer(player);
|
|
if (bPlayer == null) {
|
|
return false;
|
|
}
|
|
|
|
ChiAbility stance = bPlayer.getStance();
|
|
QuickStrike quickStrike = CoreAbility.getAbility(player, QuickStrike.class);
|
|
SwiftKick swiftKick = CoreAbility.getAbility(player, SwiftKick.class);
|
|
double newChance = getChance();
|
|
|
|
if (stance != null && stance instanceof AcrobatStance) {
|
|
newChance += ((AcrobatStance) stance).getChiBlockBoost();
|
|
}
|
|
|
|
if (quickStrike != null) {
|
|
newChance += quickStrike.getBlockChance();
|
|
} else if (swiftKick != null) {
|
|
newChance += swiftKick.getBlockChance();
|
|
}
|
|
|
|
if (Math.random() > newChance / 100.0) {
|
|
return false;
|
|
} else if (bPlayer.isChiBlocked()) {
|
|
return false;
|
|
}
|
|
return true;
|
|
}
|
|
|
|
public static void blockChi(final Player player) {
|
|
if (Suffocate.isChannelingSphere(player)) {
|
|
Suffocate.remove(player);
|
|
}
|
|
|
|
final BendingPlayer bPlayer = BendingPlayer.getBendingPlayer(player);
|
|
if (bPlayer == null) {
|
|
return;
|
|
}
|
|
bPlayer.blockChi();
|
|
player.getWorld().playSound(player.getLocation(), Sound.ENTITY_ENDERDRAGON_HURT, 2, 0);
|
|
|
|
long start = System.currentTimeMillis();
|
|
new BukkitRunnable() {
|
|
@Override
|
|
public void run() {
|
|
ActionBar.sendActionBar(Element.CHI.getColor() + "* Chiblocked *", player);
|
|
if (System.currentTimeMillis() >= start + getDuration()) {
|
|
bPlayer.unblockChi();
|
|
cancel();
|
|
}
|
|
}
|
|
}.runTaskTimer(ProjectKorra.plugin, 0, 1);
|
|
}
|
|
|
|
public static double getExhaustionFactor() {
|
|
return ConfigManager.getConfig().getDouble("Abilities.Chi.Passive.ChiSaturation.ExhaustionFactor");
|
|
}
|
|
|
|
public static double getFallReductionFactor() {
|
|
return ConfigManager.getConfig().getDouble("Abilities.Chi.Passive.Acrobatics.FallReductionFactor");
|
|
}
|
|
|
|
public static int getJumpPower() {
|
|
return ConfigManager.getConfig().getInt("Abilities.Chi.Passive.ChiAgility.JumpPower");
|
|
}
|
|
|
|
public static int getSpeedPower() {
|
|
return ConfigManager.getConfig().getInt("Abilities.Chi.Passive.ChiAgility.SpeedPower");
|
|
}
|
|
|
|
public static double getChance() {
|
|
return ConfigManager.getConfig().getDouble("Abilities.Chi.Passive.BlockChi.Chance");
|
|
}
|
|
|
|
public static int getDuration() {
|
|
return ConfigManager.getConfig().getInt("Abilities.Chi.Passive.BlockChi.Duration");
|
|
}
|
|
|
|
public static long getTicks() {
|
|
return (getDuration() / 1000) * 20;
|
|
}
|
|
|
|
}
|