mirror of
https://github.com/reactos/reactos.git
synced 2024-10-30 03:27:31 +00:00
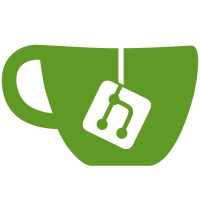
* [COMPILER_APITEST] Import MS EH/SEH tests Taken from https://github.com/microsoft/compiler-tests * [CRT] Add missing declaration of _longjmpex * [COMPILER_APITEST] Add cmake build files for MS SEH test It is built as a static library * [COMPILER_APITEST] Fix GCC build of MS SEH tests There are a number of hacks in there now. Also the volatile hacks should be separated and sent upstream. * [COMPILER_APITEST] Fix x64 build of MS SEH tests * [COMPILER_APITEST] Fix clang build of MS SEH tests * [COMPILER_APITEST] Include MS SEH tests
58 lines
885 B
C++
58 lines
885 B
C++
// Copyright (c) Microsoft. All rights reserved.
|
|
// Licensed under the MIT license. See LICENSE file in the project root for
|
|
// full license information.
|
|
|
|
// Write to volatiles in the catch to try and verify that we have
|
|
// a correct catch frame prolog and epilog.
|
|
|
|
#include <stdio.h>
|
|
#include <malloc.h>
|
|
|
|
__declspec(align(64)) struct X
|
|
{
|
|
X() : x(3) { }
|
|
~X() { }
|
|
volatile int x;
|
|
};
|
|
|
|
void t(char * c)
|
|
{
|
|
c[4] = 'a';
|
|
throw 123;
|
|
}
|
|
|
|
bool f()
|
|
{
|
|
char * buf = (char *) _alloca(10);
|
|
X x;
|
|
volatile bool caught = false;
|
|
|
|
try
|
|
{
|
|
t(buf);
|
|
}
|
|
catch(int)
|
|
{
|
|
caught = true;
|
|
x.x = 2;
|
|
}
|
|
|
|
return caught;
|
|
}
|
|
|
|
int main()
|
|
{
|
|
bool result = false;
|
|
|
|
__try {
|
|
result = f();
|
|
}
|
|
__except(1)
|
|
{
|
|
printf("ERROR - Unexpectedly caught an exception\n");
|
|
}
|
|
|
|
printf(result ? "passed\n" : "failed\n");
|
|
return result ? 0 : -1;
|
|
}
|