mirror of
https://github.com/reactos/reactos.git
synced 2024-09-20 01:31:34 +00:00
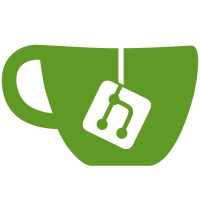
When calling CsrClientConnectToServer with a valid connection info user buffer, after capturing the buffer and calling CsrClientCallServer, do not forget to copy back to the user buffer the updated connection info and to free the capture buffer ! svn path=/branches/ros-csrss/; revision=57743
89 lines
2.2 KiB
C
89 lines
2.2 KiB
C
/*
|
|
* COPYRIGHT: See COPYING in the top level directory
|
|
* PROJECT: ReactOS kernel
|
|
* FILE: lib/ntdll/csr/api.c
|
|
* PURPOSE: CSR APIs exported through NTDLL
|
|
* PROGRAMMER: Alex Ionescu (alex@relsoft.net)
|
|
*/
|
|
|
|
/* INCLUDES *******************************************************************/
|
|
|
|
#include <ntdll.h>
|
|
#define NDEBUG
|
|
#include <debug.h>
|
|
|
|
/* GLOBALS ********************************************************************/
|
|
|
|
extern HANDLE CsrApiPort;
|
|
|
|
/* FUNCTIONS ******************************************************************/
|
|
|
|
/*
|
|
* @implemented
|
|
*/
|
|
NTSTATUS
|
|
NTAPI
|
|
CsrNewThread(VOID)
|
|
{
|
|
/* Register the termination port to CSR's */
|
|
return NtRegisterThreadTerminatePort(CsrApiPort);
|
|
}
|
|
|
|
/*
|
|
* @implemented
|
|
*/
|
|
NTSTATUS
|
|
NTAPI
|
|
CsrSetPriorityClass(HANDLE hProcess,
|
|
PULONG PriorityClass)
|
|
{
|
|
NTSTATUS Status;
|
|
CSR_API_MESSAGE ApiMessage;
|
|
PCSR_SET_PRIORITY_CLASS SetPriorityClass = &ApiMessage.Data.SetPriorityClass;
|
|
|
|
/* Set up the data for CSR */
|
|
DbgBreakPoint();
|
|
SetPriorityClass->hProcess = hProcess;
|
|
SetPriorityClass->PriorityClass = *PriorityClass;
|
|
|
|
/* Call it */
|
|
Status = CsrClientCallServer(&ApiMessage,
|
|
NULL,
|
|
CSR_CREATE_API_NUMBER(CSRSRV_SERVERDLL_INDEX, CsrpSetPriorityClass),
|
|
sizeof(CSR_SET_PRIORITY_CLASS));
|
|
|
|
/* Return what we got, if requested */
|
|
if (*PriorityClass) *PriorityClass = SetPriorityClass->PriorityClass;
|
|
|
|
/* Return to caller */
|
|
return Status;
|
|
}
|
|
|
|
/*
|
|
* @implemented
|
|
*/
|
|
NTSTATUS
|
|
NTAPI
|
|
CsrIdentifyAlertableThread(VOID)
|
|
{
|
|
NTSTATUS Status;
|
|
CSR_API_MESSAGE ApiMessage;
|
|
PCSR_IDENTIFY_ALTERTABLE_THREAD IdentifyAlertableThread;
|
|
|
|
/* Set up the data for CSR */
|
|
DbgBreakPoint();
|
|
IdentifyAlertableThread = &ApiMessage.Data.IdentifyAlertableThread;
|
|
IdentifyAlertableThread->Cid = NtCurrentTeb()->ClientId;
|
|
|
|
/* Call it */
|
|
Status = CsrClientCallServer(&ApiMessage,
|
|
NULL,
|
|
CSR_CREATE_API_NUMBER(CSRSRV_SERVERDLL_INDEX, CsrpIdentifyAlertable),
|
|
sizeof(CSR_IDENTIFY_ALTERTABLE_THREAD));
|
|
|
|
/* Return to caller */
|
|
return Status;
|
|
}
|
|
|
|
/* EOF */
|