mirror of
https://github.com/reactos/reactos.git
synced 2024-07-06 12:45:16 +00:00
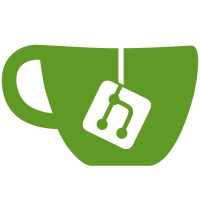
I created an "applications/screensavers" directory for that, eventually rosapps should get a similar directory structure to reactos. Also changed the appropriate parts in reactos.dff. svn path=/trunk/; revision=29961
70 lines
1.4 KiB
C
70 lines
1.4 KiB
C
//
|
|
// password.c
|
|
//
|
|
// Password support for Win9x
|
|
//
|
|
#include <windows.h>
|
|
#include <tchar.h>
|
|
|
|
typedef BOOL (WINAPI *VERIFYSCREENSAVEPWD)(HWND hwnd);
|
|
typedef VOID (WINAPI *PWDCHANGEPASSWORD)(LPCTSTR lpcRegkeyname, HWND hwnd,UINT uiReserved1,UINT uiReserved2);
|
|
|
|
BOOL VerifyPassword(HWND hwnd)
|
|
{
|
|
// Under NT, we return TRUE immediately. This lets the saver quit,
|
|
// and the system manages passwords. Under '95, we call VerifyScreenSavePwd.
|
|
// This checks the appropriate registry key and, if necessary,
|
|
// pops up a verify dialog
|
|
|
|
HINSTANCE hpwdcpl;
|
|
VERIFYSCREENSAVEPWD VerifyScreenSavePwd;
|
|
BOOL fResult;
|
|
|
|
if(GetVersion() < 0x80000000)
|
|
return TRUE;
|
|
|
|
hpwdcpl = LoadLibrary(_T("PASSWORD.CPL"));
|
|
|
|
if(hpwdcpl == NULL)
|
|
{
|
|
return FALSE;
|
|
}
|
|
|
|
|
|
VerifyScreenSavePwd = (VERIFYSCREENSAVEPWD)GetProcAddress(hpwdcpl, "VerifyScreenSavePwd");
|
|
|
|
if(VerifyScreenSavePwd == NULL)
|
|
{
|
|
FreeLibrary(hpwdcpl);
|
|
return FALSE;
|
|
}
|
|
|
|
fResult = VerifyScreenSavePwd(hwnd);
|
|
FreeLibrary(hpwdcpl);
|
|
|
|
return fResult;
|
|
}
|
|
|
|
BOOL ChangePassword(HWND hwnd)
|
|
{
|
|
// This only ever gets called under '95, when started with the /a option.
|
|
HINSTANCE hmpr = LoadLibrary(_T("MPR.DLL"));
|
|
PWDCHANGEPASSWORD PwdChangePassword;
|
|
|
|
if(hmpr == NULL)
|
|
return FALSE;
|
|
|
|
PwdChangePassword = (PWDCHANGEPASSWORD)GetProcAddress(hmpr, "PwdChangePasswordA");
|
|
|
|
if(PwdChangePassword == NULL)
|
|
{
|
|
FreeLibrary(hmpr);
|
|
return FALSE;
|
|
}
|
|
|
|
PwdChangePassword(_T("SCRSAVE"), hwnd, 0, 0);
|
|
FreeLibrary(hmpr);
|
|
|
|
return TRUE;
|
|
}
|