mirror of
https://github.com/reactos/reactos.git
synced 2024-10-04 00:13:57 +00:00
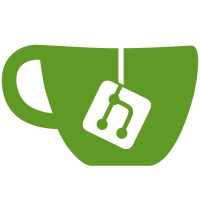
Adds the option to set the address edit box to use the display name or the full path. Also refreshes the window title and edit box in all open explorer windows when changing these settings using the folder options dialog. ## PROPOSED CHANGES ## - Create a new CabinetStateSettings type that inherits from the CABINETSTATE type. This allows us to add additional cabinet state settings not exposed in the CABINETSTATE type as well as adding a Load() method to easily populate the cabinet state settings. - Add a global cabinet state settings object. While most settings in browseui are stored independently in each shellbrowser window, cabinet state settings are global and apply to every shellbrowser window. This can be confirmed on Windows Server 2003 and Windows 7. - When receiving the WM_SETTINGCHANGE window message from the folder options dialog, refresh the title of the window and the text in the address edit box. This is the same behavior as Windows Server 2003 and Windows 7. Add a DWORD registry value to HKCU\...\Explorer\CabinetState\FullPathAddress to allow users to toggle this setting on or off in our folder options. CORE-9277
47 lines
1.8 KiB
C++
47 lines
1.8 KiB
C++
/*
|
|
* PROJECT: ReactOS browseui
|
|
* LICENSE: LGPL-2.1-or-later (https://spdx.org/licenses/LGPL-2.1-or-later)
|
|
* PURPOSE: Stores settings for the file explorer UI.
|
|
* COPYRIGHT: Copyright 2023 Carl Bialorucki <cbialo2@outlook.com>
|
|
*/
|
|
|
|
#include "precomp.h"
|
|
|
|
CabinetStateSettings gCabinetState;
|
|
|
|
void ShellSettings::Save()
|
|
{
|
|
SHRegSetUSValueW(L"Software\\Microsoft\\Internet Explorer\\Main", L"StatusBarOther",
|
|
REG_DWORD, &fStatusBarVisible, sizeof(fStatusBarVisible), SHREGSET_FORCE_HKCU);
|
|
|
|
SHRegSetUSValueW(L"Software\\Microsoft\\Internet Explorer\\Main", L"ShowGoButton",
|
|
REG_DWORD, &fShowGoButton, sizeof(fShowGoButton), SHREGSET_FORCE_HKCU);
|
|
|
|
SHRegSetUSValueW(L"Software\\Microsoft\\Internet Explorer\\Toolbar", L"Locked",
|
|
REG_DWORD, &fLocked, sizeof(fLocked), SHREGSET_FORCE_HKCU);
|
|
}
|
|
|
|
void ShellSettings::Load()
|
|
{
|
|
fStatusBarVisible = SHRegGetBoolUSValueW(L"Software\\Microsoft\\Internet Explorer\\Main",
|
|
L"StatusBarOther", FALSE, FALSE);
|
|
|
|
fShowGoButton = SHRegGetBoolUSValueW(L"Software\\Microsoft\\Internet Explorer\\Main",
|
|
L"ShowGoButton", FALSE, TRUE);
|
|
|
|
fLocked = SHRegGetBoolUSValueW(L"Software\\Microsoft\\Internet Explorer\\Toolbar",
|
|
L"Locked", FALSE, TRUE);
|
|
}
|
|
|
|
void CabinetStateSettings::Load()
|
|
{
|
|
ReadCabinetState(this, this->cLength);
|
|
|
|
/* Overrides */
|
|
fFullPathTitle = SHRegGetBoolUSValueW(L"Software\\Microsoft\\Windows\\CurrentVersion\\Explorer\\CabinetState",
|
|
L"FullPath", FALSE, FALSE);
|
|
|
|
fFullPathAddress = SHRegGetBoolUSValueW(L"Software\\Microsoft\\Windows\\CurrentVersion\\Explorer\\CabinetState",
|
|
L"FullPathAddress", FALSE, TRUE);
|
|
}
|