mirror of
https://github.com/reactos/reactos.git
synced 2024-07-12 15:45:20 +00:00
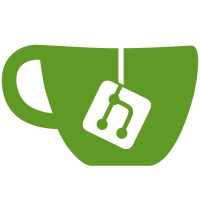
If the last chunk of the string sent to StringOut isn't a whole line and forcePrint is set to false, send back what's left after processing. The caller is then responsible for prepending that string next time it calls StringOut. Should fix the rest of debug log corruptions. svn path=/trunk/; revision=55670
53 lines
1.5 KiB
C++
53 lines
1.5 KiB
C++
/*
|
|
* PROJECT: ReactOS Automatic Testing Utility
|
|
* LICENSE: GNU GPLv2 or any later version as published by the Free Software Foundation
|
|
* PURPOSE: Helper function for shutting down the system
|
|
* COPYRIGHT: Copyright 2008-2009 Colin Finck <colin@reactos.org>
|
|
*/
|
|
|
|
#include "precomp.h"
|
|
|
|
/**
|
|
* Shuts down the system.
|
|
*
|
|
* @return
|
|
* true if everything went well, false if there was a problem while trying to shut down the system.
|
|
*/
|
|
bool ShutdownSystem()
|
|
{
|
|
HANDLE hToken;
|
|
TOKEN_PRIVILEGES Privileges;
|
|
|
|
if (!OpenProcessToken(GetCurrentProcess(), TOKEN_ADJUST_PRIVILEGES, &hToken))
|
|
{
|
|
StringOut("OpenProcessToken failed\n", TRUE);
|
|
return false;
|
|
}
|
|
|
|
/* Get the LUID for the Shutdown privilege */
|
|
if (!LookupPrivilegeValueW(NULL, SE_SHUTDOWN_NAME, &Privileges.Privileges[0].Luid))
|
|
{
|
|
StringOut("LookupPrivilegeValue failed\n", TRUE);
|
|
return false;
|
|
}
|
|
|
|
/* Assign the Shutdown privilege to our process */
|
|
Privileges.PrivilegeCount = 1;
|
|
Privileges.Privileges[0].Attributes = SE_PRIVILEGE_ENABLED;
|
|
|
|
if (!AdjustTokenPrivileges(hToken, FALSE, &Privileges, 0, NULL, NULL))
|
|
{
|
|
StringOut("AdjustTokenPrivileges failed\n", TRUE);
|
|
return false;
|
|
}
|
|
|
|
/* Finally shut down the system */
|
|
if(!ExitWindowsEx(EWX_POWEROFF, SHTDN_REASON_MAJOR_OTHER | SHTDN_REASON_MINOR_OTHER | SHTDN_REASON_FLAG_PLANNED))
|
|
{
|
|
StringOut("ExitWindowsEx failed\n", TRUE);
|
|
return false;
|
|
}
|
|
|
|
return true;
|
|
}
|