mirror of
https://github.com/reactos/reactos.git
synced 2025-07-10 09:24:21 +00:00
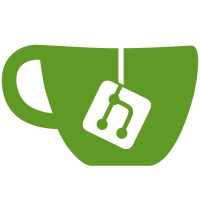
Addendum to commit 32e6eed760
(r63715)
CORE-5982
The function assumed that the directory path name to be created
always starts with a harddisk-partition root device name of the form:
\Device\HarddiskX\PartitionY\
Indeed, it can be (when using the volume manager) of the form:
\Device\HarddiskVolumeN\
and could even have a different format if trying to install ReactOS
on an external removable drive or other weird device.
Since the format of this prefix is not 100% always the same,
a different way to create the sub-directories is needed.
The nested-directory creation algorithm is changed as follows:
Suppose that the directory to be created is:
\Device\HarddiskVolume1\ReactOS\system32\drivers
The function first loops backwards each path component in order
to find the deepest existing sub-directory: it will try to verify
whether each of the following sub-directories exist, successively:
\Device\HarddiskVolume1\ReactOS\system32\drivers
\Device\HarddiskVolume1\ReactOS\system32\
\Device\HarddiskVolume1\ReactOS\
\Device\HarddiskVolume1\
(Notice the trailing path separators kept in this step.)
In principle, this root device FS directory must exist (since the
volume has been formatted previously). Once found, the function will
then create each of the sub-directories in turn:
\Device\HarddiskVolume1\ReactOS
\Device\HarddiskVolume1\ReactOS\system32
\Device\HarddiskVolume1\ReactOS\system32\drivers
----
An alternative to the fix could be to always specify the root device
name in a separate parameter, but this hasn't been pursued here so as
to not modify all the callers of this function.
127 lines
2.8 KiB
C
127 lines
2.8 KiB
C
/*
|
|
* PROJECT: ReactOS Setup Library
|
|
* LICENSE: GPL-2.0+ (https://spdx.org/licenses/GPL-2.0+)
|
|
* PURPOSE: File support functions.
|
|
* COPYRIGHT: Casper S. Hornstrup (chorns@users.sourceforge.net)
|
|
* Copyright 2017-2018 Hermes Belusca-Maito
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
NTSTATUS
|
|
SetupCreateDirectory(
|
|
_In_ PCWSTR PathName);
|
|
|
|
NTSTATUS
|
|
SetupDeleteFile(
|
|
IN PCWSTR FileName,
|
|
IN BOOLEAN ForceDelete); // ForceDelete can be used to delete read-only files
|
|
|
|
NTSTATUS
|
|
SetupCopyFile(
|
|
IN PCWSTR SourceFileName,
|
|
IN PCWSTR DestinationFileName,
|
|
IN BOOLEAN FailIfExists);
|
|
|
|
#ifndef _WINBASE_
|
|
|
|
#define MOVEFILE_REPLACE_EXISTING 1
|
|
#define MOVEFILE_COPY_ALLOWED 2
|
|
#define MOVEFILE_WRITE_THROUGH 8
|
|
|
|
#endif
|
|
|
|
NTSTATUS
|
|
SetupMoveFile(
|
|
IN PCWSTR ExistingFileName,
|
|
IN PCWSTR NewFileName,
|
|
IN ULONG Flags);
|
|
|
|
NTSTATUS
|
|
ConcatPathsV(
|
|
IN OUT PWSTR PathBuffer,
|
|
IN SIZE_T cchPathSize,
|
|
IN ULONG NumberOfPathComponents,
|
|
IN va_list PathComponentsList);
|
|
|
|
NTSTATUS
|
|
CombinePathsV(
|
|
OUT PWSTR PathBuffer,
|
|
IN SIZE_T cchPathSize,
|
|
IN ULONG NumberOfPathComponents,
|
|
IN va_list PathComponentsList);
|
|
|
|
NTSTATUS
|
|
ConcatPaths(
|
|
IN OUT PWSTR PathBuffer,
|
|
IN SIZE_T cchPathSize,
|
|
IN ULONG NumberOfPathComponents,
|
|
IN /* PCWSTR */ ...);
|
|
|
|
NTSTATUS
|
|
CombinePaths(
|
|
OUT PWSTR PathBuffer,
|
|
IN SIZE_T cchPathSize,
|
|
IN ULONG NumberOfPathComponents,
|
|
IN /* PCWSTR */ ...);
|
|
|
|
BOOLEAN
|
|
DoesPathExist_UStr(
|
|
_In_opt_ HANDLE RootDirectory,
|
|
_In_ PCUNICODE_STRING PathName,
|
|
_In_ BOOLEAN IsDirectory);
|
|
|
|
BOOLEAN
|
|
DoesPathExist(
|
|
_In_opt_ HANDLE RootDirectory,
|
|
_In_ PCWSTR PathName,
|
|
_In_ BOOLEAN IsDirectory);
|
|
|
|
#define DoesDirExist(RootDirectory, DirName) \
|
|
DoesPathExist((RootDirectory), (DirName), TRUE)
|
|
|
|
#define DoesFileExist(RootDirectory, FileName) \
|
|
DoesPathExist((RootDirectory), (FileName), FALSE)
|
|
|
|
// FIXME: DEPRECATED! HACKish function that needs to be deprecated!
|
|
BOOLEAN
|
|
DoesFileExist_2(
|
|
IN PCWSTR PathName OPTIONAL,
|
|
IN PCWSTR FileName);
|
|
|
|
BOOLEAN
|
|
NtPathToDiskPartComponents(
|
|
IN PCWSTR NtPath,
|
|
OUT PULONG pDiskNumber,
|
|
OUT PULONG pPartNumber,
|
|
OUT PCWSTR* PathComponent OPTIONAL);
|
|
|
|
NTSTATUS
|
|
OpenAndMapFile(
|
|
_In_opt_ HANDLE RootDirectory,
|
|
_In_ PCWSTR PathNameToFile,
|
|
_Out_opt_ PHANDLE FileHandle,
|
|
_Out_opt_ PULONG FileSize,
|
|
_Out_ PHANDLE SectionHandle,
|
|
_Out_ PVOID* BaseAddress,
|
|
_In_ BOOLEAN ReadWriteAccess);
|
|
|
|
NTSTATUS
|
|
MapFile(
|
|
_In_ HANDLE FileHandle,
|
|
_Out_ PHANDLE SectionHandle,
|
|
_Out_ PVOID* BaseAddress,
|
|
_In_ BOOLEAN ReadWriteAccess);
|
|
|
|
BOOLEAN
|
|
UnMapFile(
|
|
_In_ HANDLE SectionHandle,
|
|
_In_ PVOID BaseAddress);
|
|
|
|
#define UnMapAndCloseFile(FileHandle, SectionHandle, BaseAddress) \
|
|
do { \
|
|
UnMapFile((SectionHandle), (BaseAddress)); \
|
|
NtClose(FileHandle); \
|
|
} while (0)
|
|
|
|
/* EOF */
|