mirror of
https://github.com/reactos/reactos.git
synced 2024-06-30 18:01:07 +00:00
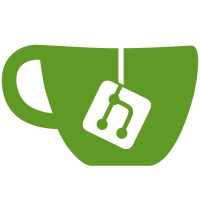
Use correct icon index in SIC_OverlayShortcutImage() to properly load
shortcut overlay icon from registry instead of always using default icon.
This allows to use custom shortcut icon set by user, in case it was
specified there.
As FIXME comment stated, the icon indexes were not implemented in the far
past, so this workaround was badly required. But now they are implemented,
so no need to always use default resource from shell32, enable the correct
code instead.
Also adapt this to CShellLink::CreateShortcutIcon() when the shortcut icon
is being changed in its properties dialog, as well as in CNewMenu class
when displaying menu items for creating a new folder or a shortcut.
Addendum to f9a5344254
. CORE-14758
117 lines
4.1 KiB
C++
117 lines
4.1 KiB
C++
/*
|
|
* provides new shell item service
|
|
*
|
|
* Copyright 2007 Johannes Anderwald (johannes.anderwald@reactos.org)
|
|
* Copyright 2009 Andrew Hill
|
|
* Copyright 2012 Rafal Harabien
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2.1 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library; if not, write to the Free Software
|
|
* Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301, USA
|
|
*/
|
|
|
|
#ifndef _SHV_ITEM_NEW_H_
|
|
#define _SHV_ITEM_NEW_H_
|
|
|
|
const WCHAR ShellNewKey[] = L"SOFTWARE\\Microsoft\\Windows\\CurrentVersion\\Explorer\\Discardable\\PostSetup\\ShellNew";
|
|
|
|
class CNewMenu :
|
|
public CComCoClass<CNewMenu, &CLSID_NewMenu>,
|
|
public CComObjectRootEx<CComMultiThreadModelNoCS>,
|
|
public IObjectWithSite,
|
|
public IContextMenu3,
|
|
public IShellExtInit
|
|
{
|
|
private:
|
|
enum SHELLNEW_TYPE
|
|
{
|
|
SHELLNEW_TYPE_INVALID = -1,
|
|
SHELLNEW_TYPE_COMMAND = 1,
|
|
SHELLNEW_TYPE_DATA = 2,
|
|
SHELLNEW_TYPE_FILENAME = 4,
|
|
SHELLNEW_TYPE_NULLFILE = 8
|
|
};
|
|
|
|
struct SHELLNEW_ITEM
|
|
{
|
|
SHELLNEW_TYPE Type;
|
|
LPWSTR pwszExt;
|
|
PBYTE pData;
|
|
ULONG cbData;
|
|
LPWSTR pwszDesc;
|
|
HICON hIcon;
|
|
SHELLNEW_ITEM *pNext;
|
|
};
|
|
|
|
LPITEMIDLIST m_pidlFolder;
|
|
SHELLNEW_ITEM *m_pItems;
|
|
SHELLNEW_ITEM *m_pLinkItem; // Points to the link handler item in the m_pItems list.
|
|
CComPtr<IUnknown> m_pSite;
|
|
HMENU m_hSubMenu;
|
|
UINT m_idCmdFirst, m_idCmdFolder, m_idCmdLink;
|
|
BOOL m_bCustomIconFolder, m_bCustomIconLink;
|
|
HICON m_hIconFolder, m_hIconLink;
|
|
|
|
SHELLNEW_ITEM *LoadItem(LPCWSTR pwszExt);
|
|
void UnloadItem(SHELLNEW_ITEM *pItem);
|
|
void UnloadAllItems();
|
|
BOOL CacheItems();
|
|
BOOL LoadCachedItems();
|
|
BOOL LoadAllItems();
|
|
UINT InsertShellNewItems(HMENU hMenu, UINT idFirst, UINT idMenu);
|
|
SHELLNEW_ITEM *FindItemFromIdOffset(UINT IdOffset);
|
|
HRESULT CreateNewFolder(LPCMINVOKECOMMANDINFO lpici);
|
|
HRESULT CreateNewItem(SHELLNEW_ITEM *pItem, LPCMINVOKECOMMANDINFO lpcmi);
|
|
HRESULT SelectNewItem(LONG wEventId, UINT uFlags, LPWSTR pszName, BOOL bRename);
|
|
HRESULT NewItemByCommand(SHELLNEW_ITEM *pItem, LPCWSTR wszPath);
|
|
HRESULT NewItemByNonCommand(SHELLNEW_ITEM *pItem, LPWSTR wszName,
|
|
DWORD cchNameMax, LPCWSTR wszPath);
|
|
|
|
public:
|
|
CNewMenu();
|
|
~CNewMenu();
|
|
|
|
// IObjectWithSite
|
|
virtual HRESULT STDMETHODCALLTYPE SetSite(IUnknown *pUnkSite);
|
|
virtual HRESULT STDMETHODCALLTYPE GetSite(REFIID riid, void **ppvSite);
|
|
|
|
// IContextMenu
|
|
virtual HRESULT WINAPI QueryContextMenu(HMENU hMenu, UINT indexMenu, UINT idCmdFirst, UINT idCmdLast, UINT uFlags);
|
|
virtual HRESULT WINAPI InvokeCommand(LPCMINVOKECOMMANDINFO lpcmi);
|
|
virtual HRESULT WINAPI GetCommandString(UINT_PTR idCommand, UINT uFlags, UINT *lpReserved, LPSTR lpszName, UINT uMaxNameLen);
|
|
|
|
// IContextMenu3
|
|
virtual HRESULT WINAPI HandleMenuMsg2(UINT uMsg, WPARAM wParam, LPARAM lParam, LRESULT *plResult);
|
|
|
|
// IContextMenu2
|
|
virtual HRESULT WINAPI HandleMenuMsg(UINT uMsg, WPARAM wParam, LPARAM lParam);
|
|
|
|
// IShellExtInit
|
|
virtual HRESULT STDMETHODCALLTYPE Initialize(PCIDLIST_ABSOLUTE pidlFolder, IDataObject *pdtobj, HKEY hkeyProgID);
|
|
|
|
DECLARE_REGISTRY_RESOURCEID(IDR_NEWMENU)
|
|
DECLARE_NOT_AGGREGATABLE(CNewMenu)
|
|
|
|
DECLARE_PROTECT_FINAL_CONSTRUCT()
|
|
|
|
BEGIN_COM_MAP(CNewMenu)
|
|
COM_INTERFACE_ENTRY_IID(IID_IObjectWithSite, IObjectWithSite)
|
|
COM_INTERFACE_ENTRY_IID(IID_IContextMenu3, IContextMenu3)
|
|
COM_INTERFACE_ENTRY_IID(IID_IContextMenu2, IContextMenu2)
|
|
COM_INTERFACE_ENTRY_IID(IID_IContextMenu, IContextMenu)
|
|
COM_INTERFACE_ENTRY_IID(IID_IShellExtInit, IShellExtInit)
|
|
END_COM_MAP()
|
|
};
|
|
|
|
#endif /* _SHV_ITEM_NEW_H_ */
|