mirror of
https://github.com/reactos/reactos.git
synced 2024-07-02 18:54:25 +00:00
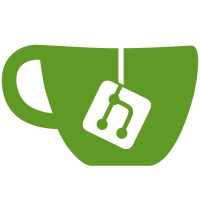
Introduce BiDi (bi-directional text) support for ExtTextOut and GetCharacterPlacement, using Wine's GDI BIDI_Reorder function. Solves the main issue with CORE-7003. To be compatible with Win2k3+, introduce the "Language Pack" (LPK) dll. - All the bidi code is removed from gdi32 and replaced by calls to LPK. Gdi32 uses dynamic linking to lpk.dll. In case of linking failure no bidi processing will be available. - Implemented LpkGetCharacterPlacement. - Implement LpkExtTextOut. - Add a demo test program to show how the apis should function. - Added all the remaining code, added special case for lpDx calculation if also GCP_GLYPHSHAPE flag was called. Applications that call GCP that use GCP_GLYPHSHAPE flags should also use the GCP_REORDER flag. (As written in https://msdn.microsoft.com/en-us/library/windows/desktop/dd144860(v=vs.85).aspx ) - Add ETO_RTLREADING flag handling. Imported the ETO_RTLREADING flag handling from wine, which changes the string part order (runs). A RRR1LLLRRR2 string without will show as RRR1LLLRRR2 without it, with it RRR2LLLRRR1.
163 lines
2.9 KiB
C
163 lines
2.9 KiB
C
/*
|
|
* COPYRIGHT: See COPYING in the top level directory
|
|
* PROJECT: ReactOS
|
|
* PURPOSE: LPK Library
|
|
* PROGRAMMER: Magnus Olsen (greatlrd)
|
|
*
|
|
*/
|
|
|
|
#include "ros_lpk.h"
|
|
|
|
#include <stubs.h>
|
|
|
|
#define UNIMPLEMENTED DbgPrint("LPK: %s is unimplemented, please try again later.\n", __FUNCTION__);
|
|
|
|
/*
|
|
* @unimplemented
|
|
*/
|
|
DWORD WINAPI LpkInitialize(DWORD x1)
|
|
{
|
|
UNIMPLEMENTED
|
|
return 0;
|
|
}
|
|
|
|
/*
|
|
* @unimplemented
|
|
*/
|
|
DWORD WINAPI LpkTabbedTextOut(DWORD x1,DWORD x2,DWORD x3,DWORD x4,DWORD x5,DWORD x6,DWORD x7,DWORD x8,DWORD x9,DWORD x10,DWORD x11,DWORD x12)
|
|
{
|
|
UNIMPLEMENTED
|
|
return 0;
|
|
}
|
|
|
|
/*
|
|
* @unimplemented
|
|
*/
|
|
DWORD WINAPI LpkDrawTextEx(DWORD x1,DWORD x2,DWORD x3,DWORD x4,DWORD x5,DWORD x6,DWORD x7,DWORD x8,DWORD x9, DWORD x10)
|
|
{
|
|
UNIMPLEMENTED
|
|
return 0;
|
|
}
|
|
|
|
/*
|
|
* @unimplemented
|
|
*/
|
|
DWORD WINAPI LpkGetTextExtentExPoint(DWORD x1,DWORD x2,DWORD x3,DWORD x4,DWORD x5,DWORD x6,DWORD x7,DWORD x8,DWORD x9)
|
|
{
|
|
UNIMPLEMENTED
|
|
return 0;
|
|
}
|
|
|
|
/*
|
|
* @unimplemented
|
|
*/
|
|
DWORD WINAPI LpkPSMTextOut(DWORD x1,DWORD x2,DWORD x3,DWORD x4,DWORD x5,DWORD x6)
|
|
{
|
|
UNIMPLEMENTED
|
|
return 0;
|
|
}
|
|
|
|
/*
|
|
* @unimplemented
|
|
*/
|
|
DWORD WINAPI LpkUseGDIWidthCache(DWORD x1,DWORD x2,DWORD x3,DWORD x4,DWORD x5)
|
|
{
|
|
UNIMPLEMENTED
|
|
return 0;
|
|
}
|
|
|
|
/*
|
|
* @unimplemented
|
|
*/
|
|
DWORD WINAPI ftsWordBreak(DWORD x1,DWORD x2,DWORD x3,DWORD x4,DWORD x5)
|
|
{
|
|
UNIMPLEMENTED
|
|
return 0;
|
|
}
|
|
|
|
DWORD WINAPI EditCreate( DWORD x1, DWORD x2)
|
|
{
|
|
UNIMPLEMENTED
|
|
return 0;
|
|
}
|
|
|
|
DWORD WINAPI EditIchToXY( DWORD x1, DWORD x2, DWORD x3, DWORD x4, DWORD x5)
|
|
{
|
|
UNIMPLEMENTED
|
|
return 0;
|
|
}
|
|
|
|
DWORD WINAPI EditMouseToIch( DWORD x1, DWORD x2, DWORD x3, DWORD x4, DWORD x5)
|
|
{
|
|
UNIMPLEMENTED
|
|
return 0;
|
|
}
|
|
|
|
DWORD WINAPI EditCchInWidth( DWORD x1, DWORD x2, DWORD x3, DWORD x4, DWORD x5)
|
|
{
|
|
UNIMPLEMENTED
|
|
return 0;
|
|
}
|
|
|
|
|
|
DWORD WINAPI EditGetLineWidth( DWORD x1, DWORD x2, DWORD x3, DWORD x4)
|
|
{
|
|
UNIMPLEMENTED
|
|
return 0;
|
|
}
|
|
|
|
DWORD WINAPI EditDrawText( DWORD x1, DWORD x2, DWORD x3, DWORD x4, DWORD x5, DWORD x6, DWORD x7)
|
|
{
|
|
UNIMPLEMENTED
|
|
return 0;
|
|
}
|
|
|
|
DWORD WINAPI EditHScroll( DWORD x1, DWORD x2, DWORD x3)
|
|
{
|
|
UNIMPLEMENTED
|
|
return 0;
|
|
}
|
|
|
|
DWORD WINAPI EditMoveSelection( DWORD x1, DWORD x2, DWORD x3, DWORD x4, DWORD x5)
|
|
{
|
|
UNIMPLEMENTED
|
|
return 0;
|
|
}
|
|
|
|
DWORD WINAPI EditVerifyText( DWORD x1, DWORD x2, DWORD x3, DWORD x4, DWORD x5, DWORD x6)
|
|
{
|
|
UNIMPLEMENTED
|
|
return 0;
|
|
}
|
|
|
|
DWORD WINAPI EditNextWord(DWORD x1, DWORD x2, DWORD x3, DWORD x4, DWORD x5, DWORD x6, DWORD x7)
|
|
{
|
|
UNIMPLEMENTED
|
|
return 0;
|
|
}
|
|
|
|
DWORD WINAPI EditSetMenu(DWORD x1, DWORD x2)
|
|
{
|
|
UNIMPLEMENTED
|
|
return 0;
|
|
}
|
|
|
|
DWORD WINAPI EditProcessMenu(DWORD x1, DWORD x2)
|
|
{
|
|
UNIMPLEMENTED
|
|
return 0;
|
|
}
|
|
|
|
DWORD WINAPI EditCreateCaret(DWORD x1, DWORD x2, DWORD x3, DWORD x4, DWORD x5)
|
|
{
|
|
UNIMPLEMENTED
|
|
return 0;
|
|
}
|
|
|
|
DWORD WINAPI EditAdjustCaret(DWORD x1, DWORD x2, DWORD x3, DWORD x5)
|
|
{
|
|
UNIMPLEMENTED
|
|
return 0;
|
|
}
|
|
|