mirror of
https://github.com/reactos/reactos.git
synced 2024-11-01 04:11:30 +00:00
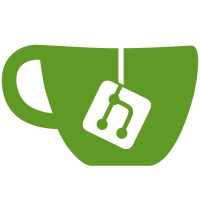
- Apart from allowing a UI cache variable that may be used when displaying GENERIC_LIST_ENTRY-ies, do not store any display strings associated to these list entries. They should be instead computed only when initializing a list UI (or a combo-box or list control if the code is used in Win32 environment). For this matter a callback is provided to InitGenericListUi() that does the job of computing the displayed string corresponding to a given GENERIC_LIST_ENTRY. - Simplify the calls to InitGenericListUi(), and refactor the RestoreGenericListUiState() function. - Use for-loops for iterating over GENERIC_LIST items. - Adapt the storage data format for lists of settings items. - The txtsetup.sif INF format specified in LoadSetupInf() should not be INF_STYLE_WIN4 (to be investigated...).
95 lines
2.1 KiB
C
95 lines
2.1 KiB
C
/*
|
|
* ReactOS kernel
|
|
* Copyright (C) 2004 ReactOS Team
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License along
|
|
* with this program; if not, write to the Free Software Foundation, Inc.,
|
|
* 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
|
|
*/
|
|
/*
|
|
* COPYRIGHT: See COPYING in the top level directory
|
|
* PROJECT: ReactOS text-mode setup
|
|
* FILE: base/setup/usetup/settings.h
|
|
* PURPOSE: Device settings support functions
|
|
* PROGRAMMERS: Colin Finck
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
/* Settings entries with simple 1:1 mapping */
|
|
typedef struct _GENENTRY
|
|
{
|
|
PCWSTR Id;
|
|
PCWSTR Value;
|
|
} GENENTRY, *PGENENTRY;
|
|
|
|
PGENERIC_LIST
|
|
CreateComputerTypeList(
|
|
IN HINF InfFile);
|
|
|
|
PGENERIC_LIST
|
|
CreateDisplayDriverList(
|
|
IN HINF InfFile);
|
|
|
|
BOOLEAN
|
|
ProcessComputerFiles(
|
|
IN HINF InfFile,
|
|
IN PGENERIC_LIST List,
|
|
OUT PWSTR* AdditionalSectionName);
|
|
|
|
BOOLEAN
|
|
ProcessDisplayRegistry(
|
|
IN HINF InfFile,
|
|
IN PGENERIC_LIST List);
|
|
|
|
PGENERIC_LIST
|
|
CreateKeyboardDriverList(
|
|
IN HINF InfFile);
|
|
|
|
PGENERIC_LIST
|
|
CreateKeyboardLayoutList(
|
|
IN HINF InfFile,
|
|
IN PCWSTR LanguageId,
|
|
OUT PWSTR DefaultKBLayout);
|
|
|
|
PGENERIC_LIST
|
|
CreateLanguageList(
|
|
IN HINF InfFile,
|
|
OUT PWSTR DefaultLanguage);
|
|
|
|
ULONG
|
|
GetDefaultLanguageIndex(VOID);
|
|
|
|
BOOLEAN
|
|
ProcessKeyboardLayoutRegistry(
|
|
IN PGENERIC_LIST List,
|
|
IN PCWSTR LanguageId);
|
|
|
|
BOOLEAN
|
|
ProcessKeyboardLayoutFiles(
|
|
IN PGENERIC_LIST List);
|
|
|
|
BOOLEAN
|
|
ProcessLocaleRegistry(
|
|
IN PGENERIC_LIST List);
|
|
|
|
BOOLEAN
|
|
SetGeoID(
|
|
IN PCWSTR Id);
|
|
|
|
BOOLEAN
|
|
SetDefaultPagefile(
|
|
IN WCHAR Drive);
|
|
|
|
/* EOF */
|