mirror of
https://github.com/reactos/reactos.git
synced 2024-07-07 05:05:09 +00:00
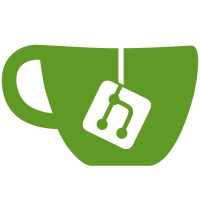
From the existing IniCacheLoad() function, introduce a IniCacheLoadFromMemory() function that just does the same (initialize an INI file cache and parse the INI file), but takes the input from a memory buffer. Then, rewrite the IniCacheLoad() function to just open the file given in input, and then fall back to calling IniCacheLoadFromMemory. The IniCacheLoadFromMemory() function will be used later. svn path=/branches/setup_improvements/; revision=74620
143 lines
2.9 KiB
C
143 lines
2.9 KiB
C
/*
|
|
* ReactOS kernel
|
|
* Copyright (C) 2002 ReactOS Team
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License along
|
|
* with this program; if not, write to the Free Software Foundation, Inc.,
|
|
* 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
|
|
*/
|
|
/*
|
|
* COPYRIGHT: See COPYING in the top level directory
|
|
* PROJECT: ReactOS text-mode setup
|
|
* FILE: base/setup/usetup/inicache.h
|
|
* PURPOSE: INI file parser that caches contents of INI file in memory
|
|
* PROGRAMMER: Royce Mitchell III
|
|
* Eric Kohl
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
typedef struct _INICACHEKEY
|
|
{
|
|
PWCHAR Name;
|
|
PWCHAR Data;
|
|
|
|
struct _INICACHEKEY *Next;
|
|
struct _INICACHEKEY *Prev;
|
|
} INICACHEKEY, *PINICACHEKEY;
|
|
|
|
|
|
typedef struct _INICACHESECTION
|
|
{
|
|
PWCHAR Name;
|
|
|
|
PINICACHEKEY FirstKey;
|
|
PINICACHEKEY LastKey;
|
|
|
|
struct _INICACHESECTION *Next;
|
|
struct _INICACHESECTION *Prev;
|
|
} INICACHESECTION, *PINICACHESECTION;
|
|
|
|
|
|
typedef struct _INICACHE
|
|
{
|
|
PINICACHESECTION FirstSection;
|
|
PINICACHESECTION LastSection;
|
|
} INICACHE, *PINICACHE;
|
|
|
|
|
|
typedef struct _PINICACHEITERATOR
|
|
{
|
|
PINICACHESECTION Section;
|
|
PINICACHEKEY Key;
|
|
} INICACHEITERATOR, *PINICACHEITERATOR;
|
|
|
|
|
|
typedef enum
|
|
{
|
|
INSERT_FIRST,
|
|
INSERT_BEFORE,
|
|
INSERT_AFTER,
|
|
INSERT_LAST
|
|
} INSERTION_TYPE;
|
|
|
|
/* FUNCTIONS ****************************************************************/
|
|
|
|
NTSTATUS
|
|
IniCacheLoadFromMemory(
|
|
PINICACHE *Cache,
|
|
PCHAR FileBuffer,
|
|
ULONG FileLength,
|
|
BOOLEAN String);
|
|
|
|
NTSTATUS
|
|
IniCacheLoad(
|
|
PINICACHE *Cache,
|
|
PWCHAR FileName,
|
|
BOOLEAN String);
|
|
|
|
VOID
|
|
IniCacheDestroy(
|
|
PINICACHE Cache);
|
|
|
|
PINICACHESECTION
|
|
IniCacheGetSection(
|
|
PINICACHE Cache,
|
|
PWCHAR Name);
|
|
|
|
NTSTATUS
|
|
IniCacheGetKey(
|
|
PINICACHESECTION Section,
|
|
PWCHAR KeyName,
|
|
PWCHAR *KeyData);
|
|
|
|
PINICACHEITERATOR
|
|
IniCacheFindFirstValue(
|
|
PINICACHESECTION Section,
|
|
PWCHAR *KeyName,
|
|
PWCHAR *KeyData);
|
|
|
|
BOOLEAN
|
|
IniCacheFindNextValue(
|
|
PINICACHEITERATOR Iterator,
|
|
PWCHAR *KeyName,
|
|
PWCHAR *KeyData);
|
|
|
|
VOID
|
|
IniCacheFindClose(
|
|
PINICACHEITERATOR Iterator);
|
|
|
|
|
|
PINICACHEKEY
|
|
IniCacheInsertKey(
|
|
PINICACHESECTION Section,
|
|
PINICACHEKEY AnchorKey,
|
|
INSERTION_TYPE InsertionType,
|
|
PWCHAR Name,
|
|
PWCHAR Data);
|
|
|
|
PINICACHE
|
|
IniCacheCreate(VOID);
|
|
|
|
NTSTATUS
|
|
IniCacheSave(
|
|
PINICACHE Cache,
|
|
PWCHAR FileName);
|
|
|
|
PINICACHESECTION
|
|
IniCacheAppendSection(
|
|
PINICACHE Cache,
|
|
PWCHAR Name);
|
|
|
|
/* EOF */
|