mirror of
https://github.com/reactos/reactos.git
synced 2024-10-22 05:46:19 +00:00
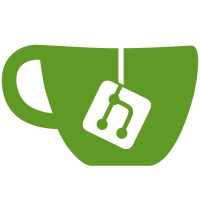
changes are: 1.add some new functions to ascii.h and unicode.h 2.add some definitions to base.h 3.add a bunch of functions to funcs.h 4.add a few structures to structs.h 5.add definitions for some missing stuff to winddi.h 6.general fixes and updates to structures and definitions in winddi.h 7.add some other structures and definitions to winddi.h 8.add some Engxxx functions to winddi.h 9.a small fix in crtdll.def 10.add all the new defs to gdi32.def and gdi32.edf 11.add all the new stubs to gdi32 stubs.c 12.implement PolyPolygon and PolyPolyline 13.some fixes to msafd.def/msafd.edf 14.change WahCloseNotificationHelper to WahCloseNotificationHandleHelper in ws2help to match the microsoft definition 15.add d3d.h, d3dcaps.h, d3dtypes.h, ddraw.h, d3dhal.h, ddrawi.h to include and include\ddk (needed for the GdiEntryxx and DdEntryxx functions in gdi32.dll). Headers are modified versions of those from WINE (stuff removed that wont compile without OLE headers, some stuff added) 16.add ddentry.h which is used with the DdEntry and GdiEntry functions and 17.fix some stuff in stubs.c of win32k A fair few of these definitions could be wrong (some of them are educated guesses, like some of the Gdixxx functions) If anyone has any corrections, any answers for the FIXMEs and structures I dont have definitions for, definitions for the remaining stuff in gdi32.def with a ; in front of it or whatever, do let me know. Or if anyone wants to comment about my changes, please let me know. svn path=/trunk/; revision=5867
129 lines
6.3 KiB
C
129 lines
6.3 KiB
C
/*
|
|
* Copyright (C) the Wine project
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2.1 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with this library; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
|
|
*/
|
|
|
|
#ifndef __WINE_D3D_H
|
|
#define __WINE_D3D_H
|
|
|
|
#include <ddraw.h>
|
|
#include <d3dtypes.h> /* must precede d3dcaps.h */
|
|
#include <d3dcaps.h>
|
|
|
|
/* ********************************************************************
|
|
Error Codes
|
|
******************************************************************** */
|
|
#define D3D_OK DD_OK
|
|
#define D3DERR_BADMAJORVERSION MAKE_DDHRESULT(700)
|
|
#define D3DERR_BADMINORVERSION MAKE_DDHRESULT(701)
|
|
#define D3DERR_INVALID_DEVICE MAKE_DDHRESULT(705)
|
|
#define D3DERR_INITFAILED MAKE_DDHRESULT(706)
|
|
#define D3DERR_DEVICEAGGREGATED MAKE_DDHRESULT(707)
|
|
#define D3DERR_EXECUTE_CREATE_FAILED MAKE_DDHRESULT(710)
|
|
#define D3DERR_EXECUTE_DESTROY_FAILED MAKE_DDHRESULT(711)
|
|
#define D3DERR_EXECUTE_LOCK_FAILED MAKE_DDHRESULT(712)
|
|
#define D3DERR_EXECUTE_UNLOCK_FAILED MAKE_DDHRESULT(713)
|
|
#define D3DERR_EXECUTE_LOCKED MAKE_DDHRESULT(714)
|
|
#define D3DERR_EXECUTE_NOT_LOCKED MAKE_DDHRESULT(715)
|
|
#define D3DERR_EXECUTE_FAILED MAKE_DDHRESULT(716)
|
|
#define D3DERR_EXECUTE_CLIPPED_FAILED MAKE_DDHRESULT(717)
|
|
#define D3DERR_TEXTURE_NO_SUPPORT MAKE_DDHRESULT(720)
|
|
#define D3DERR_TEXTURE_CREATE_FAILED MAKE_DDHRESULT(721)
|
|
#define D3DERR_TEXTURE_DESTROY_FAILED MAKE_DDHRESULT(722)
|
|
#define D3DERR_TEXTURE_LOCK_FAILED MAKE_DDHRESULT(723)
|
|
#define D3DERR_TEXTURE_UNLOCK_FAILED MAKE_DDHRESULT(724)
|
|
#define D3DERR_TEXTURE_LOAD_FAILED MAKE_DDHRESULT(725)
|
|
#define D3DERR_TEXTURE_SWAP_FAILED MAKE_DDHRESULT(726)
|
|
#define D3DERR_TEXTURE_LOCKED MAKE_DDHRESULT(727)
|
|
#define D3DERR_TEXTURE_NOT_LOCKED MAKE_DDHRESULT(728)
|
|
#define D3DERR_TEXTURE_GETSURF_FAILED MAKE_DDHRESULT(729)
|
|
#define D3DERR_MATRIX_CREATE_FAILED MAKE_DDHRESULT(730)
|
|
#define D3DERR_MATRIX_DESTROY_FAILED MAKE_DDHRESULT(731)
|
|
#define D3DERR_MATRIX_SETDATA_FAILED MAKE_DDHRESULT(732)
|
|
#define D3DERR_MATRIX_GETDATA_FAILED MAKE_DDHRESULT(733)
|
|
#define D3DERR_SETVIEWPORTDATA_FAILED MAKE_DDHRESULT(734)
|
|
#define D3DERR_INVALIDCURRENTVIEWPORT MAKE_DDHRESULT(735)
|
|
#define D3DERR_INVALIDPRIMITIVETYPE MAKE_DDHRESULT(736)
|
|
#define D3DERR_INVALIDVERTEXTYPE MAKE_DDHRESULT(737)
|
|
#define D3DERR_TEXTURE_BADSIZE MAKE_DDHRESULT(738)
|
|
#define D3DERR_INVALIDRAMPTEXTURE MAKE_DDHRESULT(739)
|
|
#define D3DERR_MATERIAL_CREATE_FAILED MAKE_DDHRESULT(740)
|
|
#define D3DERR_MATERIAL_DESTROY_FAILED MAKE_DDHRESULT(741)
|
|
#define D3DERR_MATERIAL_SETDATA_FAILED MAKE_DDHRESULT(742)
|
|
#define D3DERR_MATERIAL_GETDATA_FAILED MAKE_DDHRESULT(743)
|
|
#define D3DERR_INVALIDPALETTE MAKE_DDHRESULT(744)
|
|
#define D3DERR_ZBUFF_NEEDS_SYSTEMMEMORY MAKE_DDHRESULT(745)
|
|
#define D3DERR_ZBUFF_NEEDS_VIDEOMEMORY MAKE_DDHRESULT(746)
|
|
#define D3DERR_SURFACENOTINVIDMEM MAKE_DDHRESULT(747)
|
|
#define D3DERR_LIGHT_SET_FAILED MAKE_DDHRESULT(750)
|
|
#define D3DERR_LIGHTHASVIEWPORT MAKE_DDHRESULT(751)
|
|
#define D3DERR_LIGHTNOTINTHISVIEWPORT MAKE_DDHRESULT(752)
|
|
#define D3DERR_SCENE_IN_SCENE MAKE_DDHRESULT(760)
|
|
#define D3DERR_SCENE_NOT_IN_SCENE MAKE_DDHRESULT(761)
|
|
#define D3DERR_SCENE_BEGIN_FAILED MAKE_DDHRESULT(762)
|
|
#define D3DERR_SCENE_END_FAILED MAKE_DDHRESULT(763)
|
|
#define D3DERR_INBEGIN MAKE_DDHRESULT(770)
|
|
#define D3DERR_NOTINBEGIN MAKE_DDHRESULT(771)
|
|
#define D3DERR_NOVIEWPORTS MAKE_DDHRESULT(772)
|
|
#define D3DERR_VIEWPORTDATANOTSET MAKE_DDHRESULT(773)
|
|
#define D3DERR_VIEWPORTHASNODEVICE MAKE_DDHRESULT(774)
|
|
#define D3DERR_NOCURRENTVIEWPORT MAKE_DDHRESULT(775)
|
|
#define D3DERR_INVALIDVERTEXFORMAT MAKE_DDHRESULT(2048)
|
|
#define D3DERR_VERTEXBUFFEROPTIMIZED MAKE_DDHRESULT(2060)
|
|
#define D3DERR_VBUF_CREATE_FAILED MAKE_DDHRESULT(2061)
|
|
#define D3DERR_VERTEXBUFFERLOCKED MAKE_DDHRESULT(2062)
|
|
#define D3DERR_VERTEXBUFFERUNLOCKFAILED MAKE_DDHRESULT(2063)
|
|
#define D3DERR_ZBUFFER_NOTPRESENT MAKE_DDHRESULT(2070)
|
|
#define D3DERR_STENCILBUFFER_NOTPRESENT MAKE_DDHRESULT(2071)
|
|
|
|
#define D3DERR_WRONGTEXTUREFORMAT MAKE_DDHRESULT(2072)
|
|
#define D3DERR_UNSUPPORTEDCOLOROPERATION MAKE_DDHRESULT(2073)
|
|
#define D3DERR_UNSUPPORTEDCOLORARG MAKE_DDHRESULT(2074)
|
|
#define D3DERR_UNSUPPORTEDALPHAOPERATION MAKE_DDHRESULT(2075)
|
|
#define D3DERR_UNSUPPORTEDALPHAARG MAKE_DDHRESULT(2076)
|
|
#define D3DERR_TOOMANYOPERATIONS MAKE_DDHRESULT(2077)
|
|
#define D3DERR_CONFLICTINGTEXTUREFILTER MAKE_DDHRESULT(2078)
|
|
#define D3DERR_UNSUPPORTEDFACTORVALUE MAKE_DDHRESULT(2079)
|
|
#define D3DERR_CONFLICTINGRENDERSTATE MAKE_DDHRESULT(2081)
|
|
#define D3DERR_UNSUPPORTEDTEXTUREFILTER MAKE_DDHRESULT(2082)
|
|
#define D3DERR_TOOMANYPRIMITIVES MAKE_DDHRESULT(2083)
|
|
#define D3DERR_INVALIDMATRIX MAKE_DDHRESULT(2084)
|
|
#define D3DERR_TOOMANYVERTICES MAKE_DDHRESULT(2085)
|
|
#define D3DERR_CONFLICTINGTEXTUREPALETTE MAKE_DDHRESULT(2086)
|
|
|
|
#define D3DERR_INVALIDSTATEBLOCK MAKE_DDHRESULT(2100)
|
|
#define D3DERR_INBEGINSTATEBLOCK MAKE_DDHRESULT(2101)
|
|
#define D3DERR_NOTINBEGINSTATEBLOCK MAKE_DDHRESULT(2102)
|
|
|
|
/* ********************************************************************
|
|
Enums
|
|
******************************************************************** */
|
|
#define D3DNEXT_NEXT 0x01l
|
|
#define D3DNEXT_HEAD 0x02l
|
|
#define D3DNEXT_TAIL 0x04l
|
|
|
|
#define D3DDP_WAIT 0x00000001l
|
|
#define D3DDP_OUTOFORDER 0x00000002l
|
|
#define D3DDP_DONOTCLIP 0x00000004l
|
|
#define D3DDP_DONOTUPDATEEXTENTS 0x00000008l
|
|
#define D3DDP_DONOTLIGHT 0x00000010l
|
|
|
|
/* ********************************************************************
|
|
Types and structures
|
|
******************************************************************** */
|
|
typedef DWORD D3DVIEWPORTHANDLE, *LPD3DVIEWPORTHANDLE;
|
|
#endif
|