mirror of
https://github.com/reactos/reactos.git
synced 2024-11-07 07:00:19 +00:00
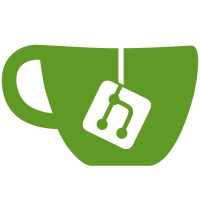
* Create a branch for some evul shell experiments. svn path=/branches/shell-experiments/; revision=61927
51 lines
1.3 KiB
C
51 lines
1.3 KiB
C
/*
|
|
* PROJECT: ReactOS Kernel
|
|
* LICENSE: BSD - See COPYING.ARM in the top level directory
|
|
* FILE: ntoskrnl/ke/i386/context.c
|
|
* PURPOSE: Context Switching Related Code
|
|
* PROGRAMMERS: ReactOS Portable Systems Group
|
|
*/
|
|
|
|
/* INCLUDES *******************************************************************/
|
|
|
|
#include <ntoskrnl.h>
|
|
#define NDEBUG
|
|
#include <debug.h>
|
|
|
|
/* GLOBALS ********************************************************************/
|
|
|
|
/* FUNCTIONS ******************************************************************/
|
|
|
|
VOID
|
|
NTAPI
|
|
KiSwapProcess(IN PKPROCESS NewProcess,
|
|
IN PKPROCESS OldProcess)
|
|
{
|
|
PKIPCR Pcr = (PKIPCR)KeGetPcr();
|
|
#ifdef CONFIG_SMP
|
|
LONG SetMember;
|
|
|
|
/* Update active processor mask */
|
|
SetMember = (LONG)Pcr->SetMember;
|
|
InterlockedXor((PLONG)&NewProcess->ActiveProcessors, SetMember);
|
|
InterlockedXor((PLONG)&OldProcess->ActiveProcessors, SetMember);
|
|
#endif
|
|
|
|
/* Check for new LDT */
|
|
if (NewProcess->LdtDescriptor.LimitLow != OldProcess->LdtDescriptor.LimitLow)
|
|
{
|
|
/* Not handled yet */
|
|
UNIMPLEMENTED_DBGBREAK();
|
|
return;
|
|
}
|
|
|
|
/* Update CR3 */
|
|
__writecr3(NewProcess->DirectoryTableBase[0]);
|
|
|
|
/* Clear GS */
|
|
Ke386SetGs(0);
|
|
|
|
/* Update IOPM offset */
|
|
Pcr->TSS->IoMapBase = NewProcess->IopmOffset;
|
|
}
|
|
|