mirror of
https://github.com/reactos/reactos.git
synced 2024-10-30 11:35:58 +00:00
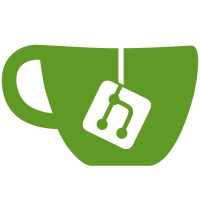
- Add a test for SendMessageTimeout failure case - Remove a pointless (and broken) memset (Coverity) CORE-8699 svn path=/trunk/; revision=64948
41 lines
1.1 KiB
C
41 lines
1.1 KiB
C
/*
|
|
* PROJECT: ReactOS API tests
|
|
* LICENSE: LGPLv2.1+ - See COPYING.LIB in the top level directory
|
|
* PURPOSE: Test for SendMessageTimeout
|
|
* PROGRAMMERS: Thomas Faber <thomas.faber@reactos.org>
|
|
*/
|
|
|
|
#include <apitest.h>
|
|
#include <winuser.h>
|
|
|
|
static
|
|
void
|
|
TestSendMessageTimeout(HWND hWnd, UINT Msg)
|
|
{
|
|
LRESULT ret;
|
|
DWORD_PTR result;
|
|
|
|
ret = SendMessageTimeoutW(hWnd, Msg, 0, 0, SMTO_NORMAL, 0, NULL);
|
|
ok(ret == 0, "ret = %Id\n", ret);
|
|
|
|
result = 0x55555555;
|
|
ret = SendMessageTimeoutW(hWnd, Msg, 0, 0, SMTO_NORMAL, 0, &result);
|
|
ok(ret == 0, "ret = %Id\n", ret);
|
|
ok(result == 0, "result = %Iu\n", result);
|
|
|
|
ret = SendMessageTimeoutA(hWnd, Msg, 0, 0, SMTO_NORMAL, 0, NULL);
|
|
ok(ret == 0, "ret = %Id\n", ret);
|
|
|
|
result = 0x55555555;
|
|
ret = SendMessageTimeoutA(hWnd, Msg, 0, 0, SMTO_NORMAL, 0, &result);
|
|
ok(ret == 0, "ret = %Id\n", ret);
|
|
ok(result == 0, "result = %Iu\n", result);
|
|
}
|
|
|
|
START_TEST(SendMessageTimeout)
|
|
{
|
|
TestSendMessageTimeout(NULL, WM_USER);
|
|
TestSendMessageTimeout(NULL, WM_PAINT);
|
|
TestSendMessageTimeout(NULL, WM_GETICON);
|
|
}
|