mirror of
https://github.com/reactos/reactos.git
synced 2025-05-13 22:30:21 +00:00
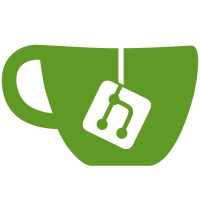
Imported from https://www.nuget.org/packages/Microsoft.Windows.SDK.CRTSource/10.0.22621.3 License: MIT
52 lines
1.1 KiB
C++
52 lines
1.1 KiB
C++
/***
|
|
*mbtokata.c - Converts character to katakana.
|
|
*
|
|
* Copyright (c) Microsoft Corporation. All rights reserved.
|
|
*
|
|
*Purpose:
|
|
* Converts a character from hiragana to katakana.
|
|
*
|
|
*******************************************************************************/
|
|
#ifndef _MBCS
|
|
#error This file should only be compiled with _MBCS defined
|
|
#endif
|
|
|
|
#include <corecrt_internal_mbstring.h>
|
|
#include <locale.h>
|
|
|
|
|
|
/***
|
|
*unsigned short _mbctokata(c) - Converts character to katakana.
|
|
*
|
|
*Purpose:
|
|
* If the character c is hiragana, convert to katakana.
|
|
*
|
|
*Entry:
|
|
* unsigned int c - Character to convert.
|
|
*
|
|
*Exit:
|
|
* Returns converted character.
|
|
*
|
|
*Exceptions:
|
|
*
|
|
*******************************************************************************/
|
|
|
|
extern "C" unsigned int __cdecl _mbctokata_l(
|
|
unsigned int c,
|
|
_locale_t plocinfo
|
|
)
|
|
{
|
|
if (_ismbchira_l(c, plocinfo)) {
|
|
c += 0xa1;
|
|
if (c >= 0x837f)
|
|
c++;
|
|
}
|
|
return(c);
|
|
}
|
|
|
|
extern "C" unsigned int (__cdecl _mbctokata)(
|
|
unsigned int c
|
|
)
|
|
{
|
|
return _mbctokata_l(c, nullptr);
|
|
}
|