mirror of
https://github.com/reactos/reactos.git
synced 2024-06-27 16:31:30 +00:00
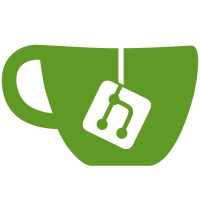
so that they wrap the needed init steps for formatting/chkdsk'ing. These helpers now accept a PPARTENTRY, together with the usual formatting/chkdsk parameters. The helpers now determine the actual NT path to use, and can perform the init steps on the partition before performing the actual operation. In particular, FormatPartition() is now made GPT-compliant. The partition type retrieved by FileSystemToMBRPartitionType() is now used as a hint for choosing FAT32 over FAT12/16, and only in the case of a MBR partition that is *NOT* a recognized OEM partition, it is used for updating the corresponding partition type. (OEM partitions must retain their original type.) The OEM partition types we (and NT) can recognize are specified e.g. in the Microsoft Open-Specification [MS-DMRP] Appendix B https://docs.microsoft.com/en-us/openspecs/windows_protocols/ms-dmrp/5f5043a3-9e6d-40cc-a05b-1a4a3617df32 Introduce an IsOEMPartition() macro to help checking for these types (its name is based on the Is***Partition() macros from ntdddisk.h, and from a dmdskmgr.dll export of similar name).
128 lines
3.5 KiB
C
128 lines
3.5 KiB
C
/*
|
|
* ReactOS kernel
|
|
* Copyright (C) 2003 ReactOS Team
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License along
|
|
* with this program; if not, write to the Free Software Foundation, Inc.,
|
|
* 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
|
|
*/
|
|
/*
|
|
* COPYRIGHT: See COPYING in the top level directory
|
|
* PROJECT: ReactOS text-mode setup
|
|
* FILE: base/setup/usetup/format.c
|
|
* PURPOSE: Filesystem format support functions
|
|
* PROGRAMMER: Casper S. Hornstrup (chorns@users.sourceforge.net)
|
|
*/
|
|
|
|
/* INCLUDES *****************************************************************/
|
|
|
|
#include "usetup.h"
|
|
|
|
#define NDEBUG
|
|
#include <debug.h>
|
|
|
|
static PPROGRESSBAR FormatProgressBar = NULL;
|
|
|
|
/* FUNCTIONS ****************************************************************/
|
|
|
|
static
|
|
BOOLEAN
|
|
NTAPI
|
|
FormatCallback(
|
|
IN CALLBACKCOMMAND Command,
|
|
IN ULONG Modifier,
|
|
IN PVOID Argument)
|
|
{
|
|
switch (Command)
|
|
{
|
|
case PROGRESS:
|
|
{
|
|
PULONG Percent;
|
|
|
|
Percent = (PULONG)Argument;
|
|
DPRINT("%lu percent completed\n", *Percent);
|
|
|
|
ProgressSetStep(FormatProgressBar, *Percent);
|
|
break;
|
|
}
|
|
|
|
#if 0
|
|
case OUTPUT:
|
|
{
|
|
PTEXTOUTPUT Output;
|
|
output = (PTEXTOUTPUT) Argument;
|
|
DPRINT("%s\n", output->Output);
|
|
break;
|
|
}
|
|
#endif
|
|
|
|
case DONE:
|
|
{
|
|
// PBOOLEAN Success;
|
|
DPRINT("Done\n");
|
|
#if 0
|
|
Success = (PBOOLEAN)Argument;
|
|
if (*Success == FALSE)
|
|
{
|
|
DPRINT("FormatEx was unable to complete successfully.\n\n");
|
|
}
|
|
#endif
|
|
break;
|
|
}
|
|
|
|
default:
|
|
DPRINT("Unknown callback %lu\n", (ULONG)Command);
|
|
break;
|
|
}
|
|
|
|
return TRUE;
|
|
}
|
|
|
|
NTSTATUS
|
|
DoFormat(
|
|
IN PPARTENTRY PartEntry,
|
|
IN PCWSTR FileSystemName,
|
|
IN BOOLEAN QuickFormat)
|
|
{
|
|
NTSTATUS Status;
|
|
|
|
FormatProgressBar = CreateProgressBar(6,
|
|
yScreen - 14,
|
|
xScreen - 7,
|
|
yScreen - 10,
|
|
10,
|
|
24,
|
|
TRUE,
|
|
MUIGetString(STRING_FORMATTINGDISK));
|
|
|
|
ProgressSetStepCount(FormatProgressBar, 100);
|
|
|
|
// TODO: Think about which values could be defaulted...
|
|
Status = FormatPartition(PartEntry,
|
|
FileSystemName,
|
|
FMIFS_HARDDISK, /* MediaFlag */
|
|
NULL, /* Label */
|
|
QuickFormat, /* QuickFormat */
|
|
0, /* ClusterSize */
|
|
FormatCallback); /* Callback */
|
|
|
|
DestroyProgressBar(FormatProgressBar);
|
|
FormatProgressBar = NULL;
|
|
|
|
DPRINT("FormatPartition() finished with status 0x%08lx\n", Status);
|
|
|
|
return Status;
|
|
}
|
|
|
|
/* EOF */
|