mirror of
https://github.com/reactos/reactos.git
synced 2024-10-06 17:35:07 +00:00
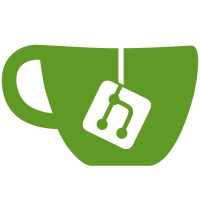
- Implement CWaitCursor class in newly-added "waitcursor.h". - Use CWaitCursor to manage the wait cursor. - Improve WM_SETCURSOR handlings. CORE-19094
55 lines
1.2 KiB
C++
55 lines
1.2 KiB
C++
/*
|
|
* PROJECT: PAINT for ReactOS
|
|
* LICENSE: LGPL-2.0-or-later (https://spdx.org/licenses/LGPL-2.0-or-later)
|
|
* PURPOSE: Wait cursor management
|
|
* COPYRIGHT: Copyright 2023 Katayama Hirofumi MZ <katayama.hirofumi.mz@gmail.com>
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
class CWaitCursor
|
|
{
|
|
public:
|
|
CWaitCursor()
|
|
{
|
|
if (s_nLock++ == 0)
|
|
{
|
|
if (!s_hWaitCursor)
|
|
s_hWaitCursor = ::LoadCursor(NULL, IDC_WAIT);
|
|
s_hOldCursor = ::SetCursor(s_hWaitCursor);
|
|
}
|
|
else
|
|
{
|
|
::SetCursor(s_hWaitCursor);
|
|
}
|
|
}
|
|
~CWaitCursor()
|
|
{
|
|
if (--s_nLock == 0)
|
|
{
|
|
::SetCursor(s_hOldCursor);
|
|
s_hOldCursor = NULL;
|
|
}
|
|
}
|
|
CWaitCursor(const CWaitCursor&) = delete;
|
|
CWaitCursor& operator=(const CWaitCursor&) = delete;
|
|
|
|
static BOOL IsWaiting()
|
|
{
|
|
return s_nLock > 0;
|
|
}
|
|
static void KeepWait()
|
|
{
|
|
::SetCursor(s_hWaitCursor);
|
|
}
|
|
|
|
protected:
|
|
static LONG s_nLock;
|
|
static HCURSOR s_hOldCursor;
|
|
static HCURSOR s_hWaitCursor;
|
|
};
|
|
|
|
DECLSPEC_SELECTANY LONG CWaitCursor::s_nLock = 0;
|
|
DECLSPEC_SELECTANY HCURSOR CWaitCursor::s_hOldCursor = NULL;
|
|
DECLSPEC_SELECTANY HCURSOR CWaitCursor::s_hWaitCursor = NULL;
|