mirror of
https://github.com/reactos/reactos.git
synced 2025-04-09 23:37:40 +00:00
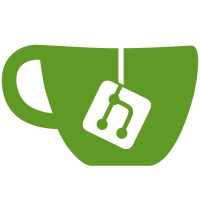
Optimize for speed and memory. JIRA issue: CORE-13950 CDirectoryList class exists just for remembering which file item is a directory or not, in order to notify the filesystem item changes. This information can become a tree data structure. - Add CFSPathIterator and CFSNode helper classes. - CFSNode is a class for tree nodes. - Re-implement CDirectoryList class by using tree nodes. - Delete CDirectoryItem class.
34 lines
903 B
C++
34 lines
903 B
C++
/*
|
|
* PROJECT: shell32
|
|
* LICENSE: LGPL-2.1-or-later (https://spdx.org/licenses/LGPL-2.1-or-later)
|
|
* PURPOSE: Shell change notification
|
|
* COPYRIGHT: Copyright 2024 Katayama Hirofumi MZ (katayama.hirofumi.mz@gmail.com)
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <atlsimpcoll.h> // for CSimpleArray
|
|
|
|
//////////////////////////////////////////////////////////////////////////////
|
|
|
|
class CFSNode;
|
|
|
|
// the directory list
|
|
class CDirectoryList
|
|
{
|
|
public:
|
|
CDirectoryList(CFSNode *pRoot);
|
|
CDirectoryList(CFSNode *pRoot, LPCWSTR pszDirectoryPath, BOOL fRecursive);
|
|
~CDirectoryList();
|
|
|
|
BOOL ContainsPath(LPCWSTR pszPath) const;
|
|
BOOL AddPath(LPCWSTR pszPath);
|
|
BOOL AddPathsFromDirectory(LPCWSTR pszDirectoryPath);
|
|
BOOL RenamePath(LPCWSTR pszPath1, LPCWSTR pszPath2);
|
|
BOOL DeletePath(LPCWSTR pszPath);
|
|
void RemoveAll();
|
|
|
|
protected:
|
|
CFSNode *m_pRoot;
|
|
BOOL m_fRecursive;
|
|
};
|