mirror of
https://github.com/reactos/reactos.git
synced 2024-09-13 22:31:34 +00:00
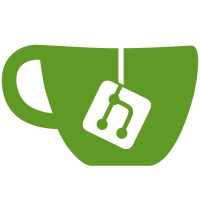
2. RtlGetVersion needs to be implemented differently in ntoskrnl and ntdll, ntoskrnl's version must not access the PEB (which might not be present) while ntdlls gets most information from the PEB structure 3. can't use spinlocks to serialize access to the security descriptor cache since it calls sd rtl functions which require to run < apc level svn path=/trunk/; revision=13712
120 lines
3.5 KiB
C
120 lines
3.5 KiB
C
/*
|
|
* ReactOS kernel
|
|
* Copyright (C) 2004 ReactOS Team
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 675 Mass Ave, Cambridge, MA 02139, USA.
|
|
*/
|
|
/* $Id$
|
|
*
|
|
* PROJECT: ReactOS kernel
|
|
* PURPOSE: Runtime code
|
|
* FILE: lib/rtl/version.c
|
|
* PROGRAMER: Filip Navara
|
|
*/
|
|
|
|
/* INCLUDES *****************************************************************/
|
|
|
|
#define __USE_W32API
|
|
|
|
#include <ddk/ntddk.h>
|
|
#include <ntdll/rtl.h>
|
|
|
|
#define NDEBUG
|
|
#include <debug.h>
|
|
|
|
/* GLOBALS ******************************************************************/
|
|
|
|
|
|
/* FUNCTIONS ****************************************************************/
|
|
|
|
/*
|
|
* @unimplemented
|
|
*/
|
|
/*
|
|
NTSTATUS
|
|
STDCALL
|
|
RtlVerifyVersionInfo(
|
|
IN PRTL_OSVERSIONINFOEXW VersionInfo,
|
|
IN ULONG TypeMask,
|
|
IN ULONGLONG ConditionMask
|
|
)
|
|
{
|
|
UNIMPLEMENTED;
|
|
return STATUS_NOT_IMPLEMENTED;
|
|
}
|
|
*/
|
|
|
|
/*
|
|
Header hell made me do it, don't blame me. Please move these somewhere more
|
|
sensible
|
|
*/
|
|
#define VER_EQUAL 1
|
|
#define VER_GREATER 2
|
|
#define VER_GREATER_EQUAL 3
|
|
#define VER_LESS 4
|
|
#define VER_LESS_EQUAL 5
|
|
#define VER_AND 6
|
|
#define VER_OR 7
|
|
|
|
#define VER_CONDITION_MASK 7
|
|
#define VER_NUM_BITS_PER_CONDITION_MASK 3
|
|
|
|
#define VER_MINORVERSION 0x0000001
|
|
#define VER_MAJORVERSION 0x0000002
|
|
#define VER_BUILDNUMBER 0x0000004
|
|
#define VER_PLATFORMID 0x0000008
|
|
#define VER_SERVICEPACKMINOR 0x0000010
|
|
#define VER_SERVICEPACKMAJOR 0x0000020
|
|
#define VER_SUITENAME 0x0000040
|
|
#define VER_PRODUCT_TYPE 0x0000080
|
|
|
|
/*
|
|
* @implemented
|
|
*/
|
|
ULONGLONG NTAPI
|
|
VerSetConditionMask(IN ULONGLONG dwlConditionMask,
|
|
IN DWORD dwTypeBitMask,
|
|
IN BYTE dwConditionMask)
|
|
{
|
|
if(dwTypeBitMask == 0)
|
|
return dwlConditionMask;
|
|
|
|
dwConditionMask &= VER_CONDITION_MASK;
|
|
|
|
if(dwConditionMask == 0)
|
|
return dwlConditionMask;
|
|
|
|
if(dwTypeBitMask & VER_PRODUCT_TYPE)
|
|
dwlConditionMask |= dwConditionMask << 7 * VER_NUM_BITS_PER_CONDITION_MASK;
|
|
else if(dwTypeBitMask & VER_SUITENAME)
|
|
dwlConditionMask |= dwConditionMask << 6 * VER_NUM_BITS_PER_CONDITION_MASK;
|
|
else if(dwTypeBitMask & VER_SERVICEPACKMAJOR)
|
|
dwlConditionMask |= dwConditionMask << 5 * VER_NUM_BITS_PER_CONDITION_MASK;
|
|
else if(dwTypeBitMask & VER_SERVICEPACKMINOR)
|
|
dwlConditionMask |= dwConditionMask << 4 * VER_NUM_BITS_PER_CONDITION_MASK;
|
|
else if(dwTypeBitMask & VER_PLATFORMID)
|
|
dwlConditionMask |= dwConditionMask << 3 * VER_NUM_BITS_PER_CONDITION_MASK;
|
|
else if(dwTypeBitMask & VER_BUILDNUMBER)
|
|
dwlConditionMask |= dwConditionMask << 2 * VER_NUM_BITS_PER_CONDITION_MASK;
|
|
else if(dwTypeBitMask & VER_MAJORVERSION)
|
|
dwlConditionMask |= dwConditionMask << 1 * VER_NUM_BITS_PER_CONDITION_MASK;
|
|
else if(dwTypeBitMask & VER_MINORVERSION)
|
|
dwlConditionMask |= dwConditionMask << 0 * VER_NUM_BITS_PER_CONDITION_MASK;
|
|
|
|
return dwlConditionMask;
|
|
}
|
|
|
|
/* EOF */
|