mirror of
https://github.com/reactos/reactos.git
synced 2025-03-10 18:24:02 +00:00
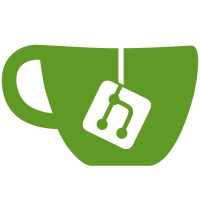
Add : doxgen tags for how NtGdiDdCanCreateD3DBuffer and NtGdiDdCanCreateD3DBuffer works Add : a sort code for the DxEngDrv so we getting the driver functions in sorted list in DxDdStartupDxGraphics, this will be part of optimize the dx code in directx it will allow us call function with gpDxFuncs[DXG_INDEX_DxD3dContextCreate].pfn instead first to search after it. Fix few bugs, as well. rember u can not still build ReactX I have not commit thuse part yet. svn path=/branches/reactx/; revision=29715
181 lines
5.5 KiB
C
181 lines
5.5 KiB
C
|
|
|
|
/*
|
|
* COPYRIGHT: See COPYING in the top level directory
|
|
* PROJECT: ReactOS kernel
|
|
* PURPOSE: Native DirectDraw implementation
|
|
* FILE: subsys/win32k/ntddraw/dvd.c
|
|
* PROGRAMER: Magnus olsen (magnus@greatlord.com)
|
|
* REVISION HISTORY:
|
|
* 19/1-2006 Magnus Olsen
|
|
*/
|
|
|
|
|
|
#include <w32k.h>
|
|
#include <debug.h>
|
|
|
|
/************************************************************************/
|
|
/* HeapVidMemAllocAligned */
|
|
/************************************************************************/
|
|
FLATPTR
|
|
STDCALL
|
|
HeapVidMemAllocAligned(LPVIDMEM lpVidMem,
|
|
DWORD dwWidth,
|
|
DWORD dwHeight,
|
|
LPSURFACEALIGNMENT lpAlignment,
|
|
LPLONG lpNewPitch)
|
|
{
|
|
PGD_HEAPVIDMEMALLOCALIGNED pfnHeapVidMemAllocAligned = NULL;
|
|
INT i;
|
|
|
|
DXG_GET_INDEX_FUNCTION(DXG_INDEX_DxDdHeapVidMemAllocAligned, pfnHeapVidMemAllocAligned);
|
|
|
|
if (pfnHeapVidMemAllocAligned == NULL)
|
|
{
|
|
DPRINT1("Warring no pfnHeapVidMemAllocAligned");
|
|
return NULL;
|
|
}
|
|
|
|
DPRINT1("Calling on dxg.sys pfnHeapVidMemAllocAligned");
|
|
return pfnHeapVidMemAllocAligned(lpVidMem, dwWidth, dwHeight, lpAlignment, lpNewPitch);
|
|
}
|
|
|
|
/************************************************************************/
|
|
/* VidMemFree */
|
|
/************************************************************************/
|
|
VOID
|
|
STDCALL
|
|
VidMemFree(LPVMEMHEAP pvmh,
|
|
FLATPTR ptr)
|
|
{
|
|
PGD_VIDMEMFREE pfnVidMemFree = NULL;
|
|
INT i;
|
|
|
|
DXG_GET_INDEX_FUNCTION(DXG_INDEX_DxDdHeapVidMemFree, pfnVidMemFree);
|
|
|
|
if (pfnVidMemFree == NULL)
|
|
{
|
|
DPRINT1("Warring no pfnVidMemFree");
|
|
}
|
|
else
|
|
{
|
|
DPRINT1("Calling on dxg.sys pfnVidMemFree");
|
|
pfnVidMemFree(pvmh, ptr);
|
|
}
|
|
}
|
|
|
|
/************************************************************************/
|
|
/* EngAllocPrivateUserMem */
|
|
/************************************************************************/
|
|
PVOID
|
|
STDCALL
|
|
EngAllocPrivateUserMem(PDD_SURFACE_LOCAL psl,
|
|
SIZE_T cj,
|
|
ULONG tag)
|
|
{
|
|
PGD_ENGALLOCPRIVATEUSERMEM pfnEngAllocPrivateUserMem = NULL;
|
|
INT i;
|
|
|
|
DXG_GET_INDEX_FUNCTION(DXG_INDEX_DxDdAllocPrivateUserMem, pfnEngAllocPrivateUserMem);
|
|
|
|
if (pfnEngAllocPrivateUserMem == NULL)
|
|
{
|
|
DPRINT1("Warring no pfnEngAllocPrivateUserMem");
|
|
return DDHAL_DRIVER_NOTHANDLED;
|
|
}
|
|
|
|
DPRINT1("Calling on dxg.sys pfnEngAllocPrivateUserMem");
|
|
return pfnEngAllocPrivateUserMem(psl, cj, tag);
|
|
}
|
|
|
|
/************************************************************************/
|
|
/* EngFreePrivateUserMem */
|
|
/************************************************************************/
|
|
VOID
|
|
STDCALL
|
|
EngFreePrivateUserMem(PDD_SURFACE_LOCAL psl,
|
|
PVOID pv)
|
|
{
|
|
PGD_ENGFREEPRIVATEUSERMEM pfnEngFreePrivateUserMem = NULL;
|
|
INT i;
|
|
|
|
DXG_GET_INDEX_FUNCTION(DXG_INDEX_DxDdFreePrivateUserMem, pfnEngFreePrivateUserMem);
|
|
|
|
if (pfnEngFreePrivateUserMem == NULL)
|
|
{
|
|
DPRINT1("Warring no pfnEngFreePrivateUserMem");
|
|
return DDHAL_DRIVER_NOTHANDLED;
|
|
}
|
|
|
|
DPRINT1("Calling on dxg.sys pfnEngFreePrivateUserMem");
|
|
return pfnEngFreePrivateUserMem(psl, pv);
|
|
}
|
|
|
|
/************************************************************************/
|
|
/* EngDxIoctl */
|
|
/************************************************************************/
|
|
DWORD
|
|
STDCALL
|
|
EngDxIoctl(ULONG ulIoctl,
|
|
PVOID pBuffer,
|
|
ULONG ulBufferSize)
|
|
{
|
|
PGD_ENGDXIOCTL pfnEngDxIoctl = NULL;
|
|
INT i;
|
|
|
|
DXG_GET_INDEX_FUNCTION(DXG_INDEX_DxDdIoctl, pfnEngDxIoctl);
|
|
|
|
if (pfnEngDxIoctl == NULL)
|
|
{
|
|
DPRINT1("Warring no pfnEngDxIoctl");
|
|
return DDHAL_DRIVER_NOTHANDLED;
|
|
}
|
|
|
|
DPRINT1("Calling on dxg.sys pfnEngDxIoctl");
|
|
return pfnEngFreePrivateUserMem(psl, pv);
|
|
}
|
|
|
|
/************************************************************************/
|
|
/* EngLockDirectDrawSurface */
|
|
/************************************************************************/
|
|
PDD_SURFACE_LOCAL
|
|
STDCALL
|
|
EngLockDirectDrawSurface(HANDLE hSurface)
|
|
{
|
|
PGD_ENGLOCKDIRECTDRAWSURFACE pfnEngLockDirectDrawSurface = NULL;
|
|
INT i;
|
|
|
|
DXG_GET_INDEX_FUNCTION(DXG_INDEX_DxDdLockDirectDrawSurface, pfnEngLockDirectDrawSurface);
|
|
|
|
if (pfnEngLockDirectDrawSurface == NULL)
|
|
{
|
|
DPRINT1("Warring no pfnEngLockDirectDrawSurface");
|
|
return DDHAL_DRIVER_NOTHANDLED;
|
|
}
|
|
|
|
DPRINT1("Calling on dxg.sys pfnEngLockDirectDrawSurface");
|
|
return pfnEngLockDirectDrawSurface(hSurface);
|
|
}
|
|
|
|
/************************************************************************/
|
|
/* EngUnlockDirectDrawSurface */
|
|
/************************************************************************/
|
|
BOOL
|
|
STDCALL
|
|
EngUnlockDirectDrawSurface(PDD_SURFACE_LOCAL pSurface)
|
|
{
|
|
PGD_ENGUNLOCKDIRECTDRAWSURFACE pfnEngUnlockDirectDrawSurface = NULL;
|
|
INT i;
|
|
|
|
DXG_GET_INDEX_FUNCTION(DXG_INDEX_DxDdUnlockDirectDrawSurface, pfnEngUnlockDirectDrawSurface);
|
|
|
|
if (pfnEngUnlockDirectDrawSurface == NULL)
|
|
{
|
|
DPRINT1("Warring no pfnEngUnlockDirectDrawSurface");
|
|
return DDHAL_DRIVER_NOTHANDLED;
|
|
}
|
|
|
|
DPRINT1("Calling on dxg.sys pfnEngUnlockDirectDrawSurface");
|
|
return pfnEngUnlockDirectDrawSurface(pSurface);
|
|
}
|
|
|