mirror of
https://github.com/reactos/reactos.git
synced 2024-11-04 13:52:30 +00:00
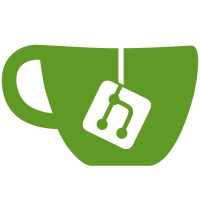
- Define a new macro function KmtGetSystemOrEmbeddedRoutineAddress() which is to be used to get a function address from Mm if it exists system-wide or to fallback to embedded function if it doesn't exist - Use this mechanism to add tests for the newly implemented FsRtlRemoveDotsFromPath() which is Vista+. That allows, with a single build (and thus, same binaries), testing a function in ReactOS and in Windows. svn path=/trunk/; revision=71046
59 lines
2.1 KiB
C
59 lines
2.1 KiB
C
/*
|
|
* PROJECT: ReactOS kernel-mode tests
|
|
* LICENSE: LGPLv2+ - See COPYING.LIB in the top level directory
|
|
* PURPOSE: Tests for FsRtlRemoveDotsFromPath
|
|
* PROGRAMMER: Pierre Schweitzer <pierre@reactos.org>
|
|
*/
|
|
|
|
#include <kmt_test.h>
|
|
|
|
#define NDEBUG
|
|
#include <debug.h>
|
|
|
|
#define InitConstString(s, c) \
|
|
wcscpy(s.Buffer, c); \
|
|
s.Buffer[sizeof(c) / sizeof(WCHAR) - 1] = 0; \
|
|
s.Length = sizeof(c) - sizeof(UNICODE_NULL)
|
|
|
|
NTSTATUS NTAPI FsRtlRemoveDotsFromPath(PWSTR OriginalString,
|
|
USHORT PathLength, USHORT *NewLength);
|
|
|
|
static
|
|
NTSTATUS
|
|
(NTAPI *pFsRtlRemoveDotsFromPath)(PWSTR OriginalString,
|
|
USHORT PathLength, USHORT *NewLength);
|
|
|
|
START_TEST(FsRtlRemoveDotsFromPath)
|
|
{
|
|
WCHAR Buf[255];
|
|
UNICODE_STRING TestString;
|
|
NTSTATUS Status;
|
|
|
|
TestString.Buffer = Buf;
|
|
TestString.MaximumLength = sizeof(Buf);
|
|
KmtGetSystemOrEmbeddedRoutineAddress(FsRtlRemoveDotsFromPath);
|
|
ASSERT(pFsRtlRemoveDotsFromPath);
|
|
|
|
InitConstString(TestString, L"\\..");
|
|
Status = pFsRtlRemoveDotsFromPath(TestString.Buffer, TestString.Length, &TestString.Length);
|
|
ok_eq_hex(Status, STATUS_IO_REPARSE_DATA_INVALID);
|
|
|
|
InitConstString(TestString, L"..");
|
|
Status = pFsRtlRemoveDotsFromPath(TestString.Buffer, TestString.Length, &TestString.Length);
|
|
ok_eq_hex(Status, STATUS_IO_REPARSE_DATA_INVALID);
|
|
|
|
InitConstString(TestString, L"..\\anyOtherContent");
|
|
Status = pFsRtlRemoveDotsFromPath(TestString.Buffer, TestString.Length, &TestString.Length);
|
|
ok_eq_hex(Status, STATUS_IO_REPARSE_DATA_INVALID);
|
|
|
|
InitConstString(TestString, L"\\\\..");
|
|
Status = pFsRtlRemoveDotsFromPath(TestString.Buffer, TestString.Length, &TestString.Length);
|
|
ok_eq_hex(Status, STATUS_SUCCESS);
|
|
ok_eq_wstr(TestString.Buffer, L"\\");
|
|
|
|
InitConstString(TestString, L"\\dir1\\dir2\\..\\dir3\\.\\file.txt");
|
|
Status = pFsRtlRemoveDotsFromPath(TestString.Buffer, TestString.Length, &TestString.Length);
|
|
ok_eq_hex(Status, STATUS_SUCCESS);
|
|
ok_eq_wstr(TestString.Buffer, L"\\dir1\\dir3\\file.txt");
|
|
}
|
|
|