mirror of
https://github.com/reactos/reactos.git
synced 2025-04-22 13:10:39 +00:00
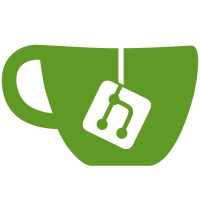
Removed page directory references from machine independant code Implemented page table reference counting Added vm test application svn path=/trunk/; revision=1309
118 lines
2.5 KiB
C
118 lines
2.5 KiB
C
/* $Id: ncache.c,v 1.6 2000/08/20 17:02:08 dwelch Exp $
|
|
*
|
|
* COPYRIGHT: See COPYING in the top level directory
|
|
* PROJECT: ReactOS kernel
|
|
* FILE: ntoskrnl/mm/cont.c
|
|
* PURPOSE: Manages non-cached memory
|
|
* PROGRAMMER: David Welch (welch@cwcom.net)
|
|
* UPDATE HISTORY:
|
|
* Created 22/05/98
|
|
*/
|
|
|
|
/* INCLUDES *****************************************************************/
|
|
|
|
#include <ddk/ntddk.h>
|
|
#include <internal/mm.h>
|
|
#include <internal/ps.h>
|
|
|
|
#define NDEBUG
|
|
#include <internal/debug.h>
|
|
|
|
/* FUNCTIONS *****************************************************************/
|
|
|
|
|
|
/**********************************************************************
|
|
* NAME EXPORTED
|
|
* MmAllocateNonCachedMemory@4
|
|
*
|
|
* DESCRIPTION
|
|
* Allocates a virtual address range of noncached and cache
|
|
* aligned memory.
|
|
*
|
|
* ARGUMENTS
|
|
* NumberOfBytes
|
|
* Size of region to allocate.
|
|
*
|
|
* RETURN VALUE
|
|
* The base address of the range on success;
|
|
* NULL on failure.
|
|
*
|
|
* NOTE
|
|
* Description taken from include/ddk/mmfuncs.h.
|
|
* Code taken from ntoskrnl/mm/special.c.
|
|
*
|
|
* REVISIONS
|
|
*
|
|
*/
|
|
PVOID STDCALL MmAllocateNonCachedMemory(IN ULONG NumberOfBytes)
|
|
{
|
|
PVOID Result;
|
|
MEMORY_AREA* marea;
|
|
NTSTATUS Status;
|
|
ULONG i;
|
|
|
|
Result = NULL;
|
|
Status = MmCreateMemoryArea (NULL,
|
|
MmGetKernelAddressSpace(),
|
|
MEMORY_AREA_NO_CACHE,
|
|
&Result,
|
|
NumberOfBytes,
|
|
0,
|
|
&marea);
|
|
if (!NT_SUCCESS(Status))
|
|
{
|
|
return (NULL);
|
|
}
|
|
for (i = 0; (i <= (NumberOfBytes / PAGESIZE)); i++)
|
|
{
|
|
Status = MmCreateVirtualMapping (NULL,
|
|
(Result + (i * PAGESIZE)),
|
|
PAGE_READWRITE,
|
|
(ULONG)MmAllocPage(0));
|
|
if (!NT_SUCCESS(Status))
|
|
{
|
|
DbgPrint("Unable to create virtual mapping\n");
|
|
KeBugCheck(0);
|
|
}
|
|
}
|
|
return ((PVOID)Result);
|
|
}
|
|
|
|
|
|
|
|
/**********************************************************************
|
|
* NAME EXPORTED
|
|
* MmFreeNonCachedMemory@8
|
|
*
|
|
* DESCRIPTION
|
|
* Releases a range of noncached memory allocated with
|
|
* MmAllocateNonCachedMemory.
|
|
*
|
|
* ARGUMENTS
|
|
* BaseAddress
|
|
* Virtual address to be freed;
|
|
*
|
|
* NumberOfBytes
|
|
* Size of the region to be freed.
|
|
*
|
|
* RETURN VALUE
|
|
* None.
|
|
*
|
|
* NOTE
|
|
* Description taken from include/ddk/mmfuncs.h.
|
|
* Code taken from ntoskrnl/mm/special.c.
|
|
*
|
|
* REVISIONS
|
|
*
|
|
*/
|
|
VOID STDCALL MmFreeNonCachedMemory (IN PVOID BaseAddress,
|
|
IN ULONG NumberOfBytes)
|
|
{
|
|
MmFreeMemoryArea (&PsGetCurrentProcess()->AddressSpace,
|
|
BaseAddress,
|
|
NumberOfBytes,
|
|
TRUE);
|
|
}
|
|
|
|
|
|
/* EOF */
|