mirror of
https://github.com/reactos/reactos.git
synced 2025-01-01 03:54:02 +00:00
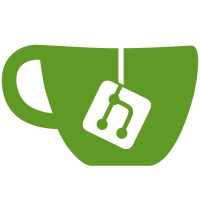
Implement volume level changing for Aux/MidiOut/WaveOut devices. It's represented the following WINMM functions: - auxGetVolume, - auxSetVolume, - midiOutGetVolume, - midiOutSetVolume, - waveOutGetVolume, - waveOutSetVolume, which are calling the followind messages appropriately: - AUXDM_GETVOLUME, - AUXDM_SETVOLUME, - MODM_GETVOLUME, - MODM_SETVOLUME, - WODM_GETVOLUME, - WODM_SETVOLUME. This fixes volume control for several 3rd-party programs (like Fox Audio Player 0.10.2 from Rapps, Winamp 2.95 with WaveOut plugin). However it does not fix changing the volume in system volume mixers (SndVol32, MMSys), since they are using their own functionality instead. They technically do the same things, but apart from the functions mentioned above. CORE-14780
269 lines
5.8 KiB
C
269 lines
5.8 KiB
C
#ifndef __WDMAUD_H__
|
|
#define __WDMAUD_H__
|
|
|
|
#include <stdarg.h>
|
|
|
|
#define WIN32_NO_STATUS
|
|
#define _INC_WINDOWS
|
|
#define COM_NO_WINDOWS_H
|
|
|
|
#include <windef.h>
|
|
#include <winbase.h>
|
|
#include <winreg.h>
|
|
|
|
#include <winuser.h>
|
|
#include <mmddk.h>
|
|
#include <mmebuddy.h>
|
|
#include <ks.h>
|
|
#include <ksmedia.h>
|
|
#include <interface.h>
|
|
#include <devioctl.h>
|
|
#include <setupapi.h>
|
|
|
|
BOOL
|
|
WdmAudInitUserModeMixer(VOID);
|
|
|
|
ULONG
|
|
WdmAudGetWaveOutCount(VOID);
|
|
|
|
ULONG
|
|
WdmAudGetWaveInCount(VOID);
|
|
|
|
ULONG
|
|
WdmAudGetMixerCount(VOID);
|
|
|
|
MMRESULT
|
|
WdmAudGetNumWdmDevsByMMixer(
|
|
IN MMDEVICE_TYPE DeviceType,
|
|
OUT DWORD* DeviceCount);
|
|
|
|
MMRESULT
|
|
WdmAudCommitWaveBufferByLegacy(
|
|
IN PSOUND_DEVICE_INSTANCE SoundDeviceInstance,
|
|
IN PVOID OffsetPtr,
|
|
IN DWORD Length,
|
|
IN PSOUND_OVERLAPPED Overlap,
|
|
IN LPOVERLAPPED_COMPLETION_ROUTINE CompletionRoutine);
|
|
|
|
MMRESULT
|
|
WriteFileEx_Remixer(
|
|
IN PSOUND_DEVICE_INSTANCE SoundDeviceInstance,
|
|
IN PVOID OffsetPtr,
|
|
IN DWORD Length,
|
|
IN PSOUND_OVERLAPPED Overlap,
|
|
IN LPOVERLAPPED_COMPLETION_ROUTINE CompletionRoutine);
|
|
|
|
MMRESULT
|
|
WdmAudGetCapabilitiesByMMixer(
|
|
IN PSOUND_DEVICE SoundDevice,
|
|
IN DWORD DeviceId,
|
|
OUT PVOID Capabilities,
|
|
IN DWORD CapabilitiesSize);
|
|
|
|
MMRESULT
|
|
WdmAudOpenSoundDeviceByMMixer(
|
|
IN struct _SOUND_DEVICE* SoundDevice,
|
|
OUT PVOID* Handle);
|
|
|
|
MMRESULT
|
|
WdmAudCloseSoundDeviceByMMixer(
|
|
IN struct _SOUND_DEVICE_INSTANCE* SoundDeviceInstance,
|
|
IN PVOID Handle);
|
|
|
|
MMRESULT
|
|
WdmAudGetLineInfo(
|
|
IN HANDLE hMixer,
|
|
IN DWORD MixerId,
|
|
IN LPMIXERLINEW MixLine,
|
|
IN ULONG Flags);
|
|
|
|
MMRESULT
|
|
WdmAudGetLineControls(
|
|
IN HANDLE hMixer,
|
|
IN DWORD MixerId,
|
|
IN LPMIXERLINECONTROLSW MixControls,
|
|
IN ULONG Flags);
|
|
|
|
MMRESULT
|
|
WdmAudSetControlDetails(
|
|
IN HANDLE hMixer,
|
|
IN DWORD MixerId,
|
|
IN LPMIXERCONTROLDETAILS MixDetails,
|
|
IN ULONG Flags);
|
|
|
|
MMRESULT
|
|
WdmAudGetControlDetails(
|
|
IN HANDLE hMixer,
|
|
IN DWORD MixerId,
|
|
IN LPMIXERCONTROLDETAILS MixDetails,
|
|
IN ULONG Flags);
|
|
|
|
MMRESULT
|
|
WdmAudSetWaveDeviceFormatByMMixer(
|
|
IN PSOUND_DEVICE_INSTANCE Instance,
|
|
IN DWORD DeviceId,
|
|
IN PWAVEFORMATEX WaveFormat,
|
|
IN DWORD WaveFormatSize);
|
|
|
|
MMRESULT
|
|
WdmAudGetDeviceInterfaceStringByMMixer(
|
|
IN MMDEVICE_TYPE DeviceType,
|
|
IN DWORD DeviceId,
|
|
IN LPWSTR Interface,
|
|
IN DWORD InterfaceLength,
|
|
OUT DWORD * InterfaceSize);
|
|
|
|
MMRESULT
|
|
WdmAudSetMixerDeviceFormatByMMixer(
|
|
IN PSOUND_DEVICE_INSTANCE Instance,
|
|
IN DWORD DeviceId,
|
|
IN PWAVEFORMATEX WaveFormat,
|
|
IN DWORD WaveFormatSize);
|
|
|
|
MMRESULT
|
|
WdmAudQueryMixerInfoByMMixer(
|
|
IN struct _SOUND_DEVICE_INSTANCE* SoundDeviceInstance,
|
|
IN DWORD DeviceId,
|
|
IN UINT uMsg,
|
|
IN LPVOID Parameter,
|
|
IN DWORD Flags);
|
|
|
|
MMRESULT
|
|
WdmAudSetWaveStateByMMixer(
|
|
IN struct _SOUND_DEVICE_INSTANCE* SoundDeviceInstance,
|
|
IN BOOL bStart);
|
|
|
|
MMRESULT
|
|
WdmAudResetStreamByMMixer(
|
|
IN struct _SOUND_DEVICE_INSTANCE* SoundDeviceInstance,
|
|
IN MMDEVICE_TYPE DeviceType,
|
|
IN BOOLEAN bStartReset);
|
|
|
|
MMRESULT
|
|
WdmAudGetWavePositionByMMixer(
|
|
IN struct _SOUND_DEVICE_INSTANCE* SoundDeviceInstance,
|
|
IN MMTIME* Time);
|
|
|
|
MMRESULT
|
|
WdmAudGetVolumeByMMixer(
|
|
_In_ PSOUND_DEVICE_INSTANCE SoundDeviceInstance,
|
|
_In_ DWORD DeviceId,
|
|
_Out_ PDWORD pdwVolume);
|
|
|
|
MMRESULT
|
|
WdmAudSetVolumeByMMixer(
|
|
_In_ PSOUND_DEVICE_INSTANCE SoundDeviceInstance,
|
|
_In_ DWORD DeviceId,
|
|
_In_ DWORD dwVolume);
|
|
|
|
MMRESULT
|
|
WdmAudCommitWaveBufferByMMixer(
|
|
IN PSOUND_DEVICE_INSTANCE SoundDeviceInstance,
|
|
IN PVOID OffsetPtr,
|
|
IN DWORD Length,
|
|
IN PSOUND_OVERLAPPED Overlap,
|
|
IN LPOVERLAPPED_COMPLETION_ROUTINE CompletionRoutine);
|
|
|
|
MMRESULT
|
|
WdmAudCleanupByMMixer(VOID);
|
|
|
|
/* legacy.c */
|
|
|
|
MMRESULT
|
|
WdmAudCleanupByLegacy(VOID);
|
|
|
|
MMRESULT
|
|
WdmAudGetCapabilitiesByLegacy(
|
|
IN PSOUND_DEVICE SoundDevice,
|
|
IN DWORD DeviceId,
|
|
OUT PVOID Capabilities,
|
|
IN DWORD CapabilitiesSize);
|
|
|
|
MMRESULT
|
|
WdmAudOpenSoundDeviceByLegacy(
|
|
IN PSOUND_DEVICE SoundDevice,
|
|
OUT PVOID *Handle
|
|
);
|
|
|
|
MMRESULT
|
|
WdmAudCloseSoundDeviceByLegacy(
|
|
IN struct _SOUND_DEVICE_INSTANCE* SoundDeviceInstance,
|
|
IN PVOID Handle);
|
|
|
|
MMRESULT
|
|
WdmAudGetDeviceInterfaceStringByLegacy(
|
|
IN MMDEVICE_TYPE DeviceType,
|
|
IN DWORD DeviceId,
|
|
IN LPWSTR Interface,
|
|
IN DWORD InterfaceLength,
|
|
OUT DWORD * InterfaceSize);
|
|
|
|
MMRESULT
|
|
WdmAudSetMixerDeviceFormatByLegacy(
|
|
IN PSOUND_DEVICE_INSTANCE Instance,
|
|
IN DWORD DeviceId,
|
|
IN PWAVEFORMATEX WaveFormat,
|
|
IN DWORD WaveFormatSize);
|
|
|
|
MMRESULT
|
|
WdmAudQueryMixerInfoByLegacy(
|
|
IN struct _SOUND_DEVICE_INSTANCE* SoundDeviceInstance,
|
|
IN DWORD DeviceId,
|
|
IN UINT uMsg,
|
|
IN LPVOID Parameter,
|
|
IN DWORD Flags);
|
|
|
|
MMRESULT
|
|
WdmAudSetWaveDeviceFormatByLegacy(
|
|
IN PSOUND_DEVICE_INSTANCE Instance,
|
|
IN DWORD DeviceId,
|
|
IN PWAVEFORMATEX WaveFormat,
|
|
IN DWORD WaveFormatSize);
|
|
|
|
MMRESULT
|
|
WdmAudSetWaveStateByLegacy(
|
|
IN struct _SOUND_DEVICE_INSTANCE* SoundDeviceInstance,
|
|
IN BOOL bStart);
|
|
|
|
MMRESULT
|
|
WdmAudResetStreamByLegacy(
|
|
IN struct _SOUND_DEVICE_INSTANCE* SoundDeviceInstance,
|
|
IN MMDEVICE_TYPE DeviceType,
|
|
IN BOOLEAN bStartReset);
|
|
|
|
MMRESULT
|
|
WdmAudGetWavePositionByLegacy(
|
|
IN struct _SOUND_DEVICE_INSTANCE* SoundDeviceInstance,
|
|
IN MMTIME* Time);
|
|
|
|
MMRESULT
|
|
WdmAudGetVolumeByLegacy(
|
|
_In_ PSOUND_DEVICE_INSTANCE SoundDeviceInstance,
|
|
_In_ DWORD DeviceId,
|
|
_Out_ PDWORD pdwVolume);
|
|
|
|
MMRESULT
|
|
WdmAudSetVolumeByLegacy(
|
|
_In_ PSOUND_DEVICE_INSTANCE SoundDeviceInstance,
|
|
_In_ DWORD DeviceId,
|
|
_In_ DWORD dwVolume);
|
|
|
|
MMRESULT
|
|
WriteFileEx_Committer2(
|
|
IN PSOUND_DEVICE_INSTANCE SoundDeviceInstance,
|
|
IN PVOID OffsetPtr,
|
|
IN DWORD Length,
|
|
IN PSOUND_OVERLAPPED Overlap,
|
|
IN LPOVERLAPPED_COMPLETION_ROUTINE CompletionRoutine);
|
|
|
|
MMRESULT
|
|
WdmAudGetNumWdmDevsByLegacy(
|
|
IN MMDEVICE_TYPE DeviceType,
|
|
OUT DWORD* DeviceCount);
|
|
|
|
DWORD
|
|
WINAPI
|
|
MixerEventThreadRoutine(
|
|
LPVOID Parameter);
|
|
|
|
#endif /* __WDMAUD_H__ */
|