mirror of
https://github.com/reactos/reactos.git
synced 2024-09-18 00:33:04 +00:00
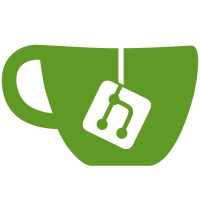
Rewrite the bitmap API. There were a lot of bugs. NtGdiCreateBitmap allowed a negative height, leading to either topdown or bottomup bitmaps, a behaviour that Windows doesn't have. The function copied the bitmap bits directly from the caller to the bitmap using RtlCopyMemory, ignoring different scanline length and direction (resulting in bitmaps being upside down), not SEH protected. This function (IntSetBitmapBits) is replaced by a better solution UnsafeSetBitmapBits, that takes these things into account. The name is chosen to give a hint that the function can/should be SEH protected. IntSetBitmapBits is still there, as its retarded behaviour is actually required in some places. There were also IntCreateBitmap and IntGdiCreateBitmap, now both being replaced by GreCreateBitmap. The code that set the palette is removed, as it's already done in SURFACE_AllocSurface, here gpalRGB is replaced with gpalBGR, fixing some inverted color issues. The alignment correction in SURFACE_bSetBitmapBits is reapplied, now that the callers are behaving as they are supposed to do. svn path=/branches/reactos-yarotows/; revision=47641
133 lines
4.3 KiB
C
133 lines
4.3 KiB
C
#pragma once
|
|
|
|
#include "win32.h"
|
|
#include "gdiobj.h"
|
|
|
|
#define PDEV_SURFACE 0x80000000
|
|
|
|
/* GDI surface object */
|
|
typedef struct _SURFACE
|
|
{
|
|
BASEOBJECT BaseObject;
|
|
|
|
SURFOBJ SurfObj;
|
|
//XDCOBJ * pdcoAA;
|
|
FLONG flags;
|
|
struct _PALETTE *ppal;
|
|
//UINT unk_050;
|
|
|
|
union
|
|
{
|
|
HANDLE hSecureUMPD; // if UMPD_SURFACE set
|
|
HANDLE hMirrorParent;// if MIRROR_SURFACE set
|
|
HANDLE hDDSurface; // if DIRECTDRAW_SURFACE set
|
|
};
|
|
|
|
SIZEL sizlDim; /* For SetBitmapDimension(), do NOT use
|
|
to get width/height of bitmap, use
|
|
bitmap.bmWidth/bitmap.bmHeight for
|
|
that */
|
|
|
|
HDC hdc; // Doc in "Undocumented Windows", page 546, seems to be supported with XP.
|
|
ULONG cRef;
|
|
HPALETTE hpalHint;
|
|
|
|
/* For device-independent bitmaps: */
|
|
HANDLE hDIBSection;
|
|
HANDLE hSecure;
|
|
DWORD dwOffset;
|
|
//UINT unk_078;
|
|
|
|
/* reactos specific */
|
|
DWORD dsBitfields[3]; // hack, should probably use palette instead
|
|
DWORD biClrUsed;
|
|
DWORD biClrImportant;
|
|
} SURFACE, *PSURFACE;
|
|
|
|
// flags field:
|
|
//#define HOOK_BITBLT 0x00000001
|
|
//#define HOOK_STRETCHBLT 0x00000002
|
|
//#define HOOK_PLGBLT 0x00000004
|
|
//#define HOOK_TEXTOUT 0x00000008
|
|
//#define HOOK_PAINT 0x00000010
|
|
//#define HOOK_STROKEPATH 0x00000020
|
|
//#define HOOK_FILLPATH 0x00000040
|
|
//#define HOOK_STROKEANDFILLPATH 0x00000080
|
|
//#define HOOK_LINETO 0x00000100
|
|
//#define SHAREACCESS_SURFACE 0x00000200
|
|
//#define HOOK_COPYBITS 0x00000400
|
|
//#define REDIRECTION_SURFACE 0x00000800 // ?
|
|
//#define HOOK_MOVEPANNING 0x00000800
|
|
//#define HOOK_SYNCHRONIZE 0x00001000
|
|
//#define HOOK_STRETCHBLTROP 0x00002000
|
|
//#define HOOK_SYNCHRONIZEACCESS 0x00004000
|
|
//#define USE_DEVLOCK_SURFACE 0x00004000
|
|
//#define HOOK_TRANSPARENTBLT 0x00008000
|
|
//#define HOOK_ALPHABLEND 0x00010000
|
|
//#define HOOK_GRADIENTFILL 0x00020000
|
|
//#if (NTDDI_VERSION < 0x06000000)
|
|
// #define HOOK_FLAGS 0x0003B5FF
|
|
//#else
|
|
// #define HOOK_FLAGS 0x0003B5EF
|
|
//#endif
|
|
#define UMPD_SURFACE 0x00040000
|
|
#define MIRROR_SURFACE 0x00080000
|
|
#define DIRECTDRAW_SURFACE 0x00100000
|
|
#define DRIVER_CREATED_SURFACE 0x00200000
|
|
#define ENG_CREATE_DEVICE_SURFACE 0x00400000
|
|
#define DDB_SURFACE 0x00800000
|
|
#define LAZY_DELETE_SURFACE 0x01000000
|
|
#define BANDING_SURFACE 0x02000000
|
|
#define API_BITMAP 0x04000000
|
|
#define PALETTE_SELECT_SET 0x08000000
|
|
#define UNREADABLE_SURFACE 0x10000000
|
|
#define DYNAMIC_MODE_PALETTE 0x20000000
|
|
#define ABORT_SURFACE 0x40000000
|
|
#define PDEV_SURFACE 0x80000000
|
|
|
|
|
|
#define BMF_DONT_FREE 0x100
|
|
#define BMF_RLE_HACK 0x200
|
|
|
|
|
|
/* Internal interface */
|
|
|
|
#define SURFACE_AllocSurfaceWithHandle() ((PSURFACE) GDIOBJ_AllocObjWithHandle(GDI_OBJECT_TYPE_BITMAP))
|
|
#define SURFACE_FreeSurface(pBMObj) GDIOBJ_FreeObj((POBJ) pBMObj, GDIObjType_SURF_TYPE)
|
|
#define SURFACE_FreeSurfaceByHandle(hBMObj) GDIOBJ_FreeObjByHandle((HGDIOBJ) hBMObj, GDI_OBJECT_TYPE_BITMAP)
|
|
|
|
/* NOTE: Use shared locks! */
|
|
#define SURFACE_LockSurface(hBMObj) \
|
|
((PSURFACE) GDIOBJ_LockObj ((HGDIOBJ) hBMObj, GDI_OBJECT_TYPE_BITMAP))
|
|
#define SURFACE_ShareLockSurface(hBMObj) \
|
|
((PSURFACE) GDIOBJ_ShareLockObj ((HGDIOBJ) hBMObj, GDI_OBJECT_TYPE_BITMAP))
|
|
#define SURFACE_UnlockSurface(pBMObj) \
|
|
GDIOBJ_UnlockObjByPtr ((POBJ)pBMObj)
|
|
#define SURFACE_ShareUnlockSurface(pBMObj) \
|
|
GDIOBJ_ShareUnlockObjByPtr ((POBJ)pBMObj)
|
|
|
|
BOOL INTERNAL_CALL SURFACE_Cleanup(PVOID ObjectBody);
|
|
|
|
PSURFACE
|
|
NTAPI
|
|
SURFACE_AllocSurface(
|
|
IN ULONG iType,
|
|
IN ULONG cx,
|
|
IN ULONG cy,
|
|
IN ULONG iFormat);
|
|
|
|
BOOL
|
|
NTAPI
|
|
SURFACE_bSetBitmapBits(
|
|
IN PSURFACE psurf,
|
|
IN USHORT fjBitmap,
|
|
IN ULONG ulWidth,
|
|
IN PVOID pvBits OPTIONAL);
|
|
|
|
#define GDIDEV(SurfObj) ((PDEVOBJ *)((SurfObj)->hdev))
|
|
#define GDIDEVFUNCS(SurfObj) ((PDEVOBJ *)((SurfObj)->hdev))->DriverFunctions
|
|
|
|
ULONG FASTCALL BitmapFormat (WORD Bits, DWORD Compression);
|
|
extern UCHAR gajBitsPerFormat[];
|
|
#define BitsPerFormat(Format) gajBitsPerFormat[Format]
|