mirror of
https://github.com/reactos/reactos.git
synced 2024-07-05 12:15:46 +00:00
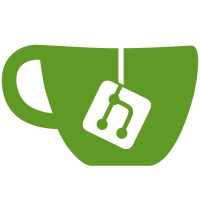
* [RAPPS] Display custom applications icons for installed applications - Implement `RetrieveIcon` helper function in `CInstalledApplicationInfo` class, which retrueves the current app's icon from registry, same as it done for `CAvailableApplicationInfo`. - Use it for loading the icon in `CAppsListView::AddInstalledApplication` function, via `ExtractIconW`. Load default Rapps icon only when the app has no its custom icon. - Retrieve `DisplayIcon` value from registry in `CInstalledApps::Enum` function, same as other registry values (like app name, description, etc).Store it in `szDisplayIcon` string, which is used in `CInstalledApplicationInfo::RetrieveIcon` for retrieving the data of that value. - Increase `LISTVIEW_ICON_SIZE` macro from 24 to 32, so 32x32 icon size is now used instead of 24x24. This makes displayed icons more accurate, since most of apps contain 32x32 icon, so they look a bit distorted with 24x24 size.
57 lines
1.4 KiB
C++
57 lines
1.4 KiB
C++
#pragma once
|
|
|
|
#include <windef.h>
|
|
#include <atlstr.h>
|
|
|
|
class CInstalledApplicationInfo
|
|
{
|
|
public:
|
|
BOOL IsUserKey;
|
|
REGSAM WowKey;
|
|
HKEY hSubKey;
|
|
BOOL bIsUpdate = FALSE;
|
|
|
|
ATL::CStringW szKeyName;
|
|
|
|
CInstalledApplicationInfo(BOOL bIsUserKey, REGSAM RegWowKey, HKEY hKey);
|
|
BOOL GetApplicationRegString(LPCWSTR lpKeyName, ATL::CStringW& String);
|
|
BOOL GetApplicationRegDword(LPCWSTR lpKeyName, DWORD *lpValue);
|
|
BOOL RetrieveIcon(ATL::CStringW& IconLocation);
|
|
BOOL UninstallApplication(BOOL bModify);
|
|
LSTATUS RemoveFromRegistry();
|
|
|
|
ATL::CStringW szDisplayIcon;
|
|
ATL::CStringW szDisplayName;
|
|
ATL::CStringW szDisplayVersion;
|
|
ATL::CStringW szPublisher;
|
|
ATL::CStringW szRegOwner;
|
|
ATL::CStringW szProductID;
|
|
ATL::CStringW szHelpLink;
|
|
ATL::CStringW szHelpTelephone;
|
|
ATL::CStringW szReadme;
|
|
ATL::CStringW szContact;
|
|
ATL::CStringW szURLUpdateInfo;
|
|
ATL::CStringW szURLInfoAbout;
|
|
ATL::CStringW szComments;
|
|
ATL::CStringW szInstallDate;
|
|
ATL::CStringW szInstallLocation;
|
|
ATL::CStringW szInstallSource;
|
|
ATL::CStringW szUninstallString;
|
|
ATL::CStringW szModifyPath;
|
|
|
|
~CInstalledApplicationInfo();
|
|
};
|
|
|
|
typedef BOOL(CALLBACK *APPENUMPROC)(CInstalledApplicationInfo * Info, PVOID param);
|
|
|
|
class CInstalledApps
|
|
{
|
|
ATL::CAtlList<CInstalledApplicationInfo *> m_InfoList;
|
|
|
|
public:
|
|
BOOL Enum(INT EnumType, APPENUMPROC lpEnumProc, PVOID param);
|
|
|
|
VOID FreeCachedEntries();
|
|
};
|
|
|