mirror of
https://github.com/reactos/reactos.git
synced 2024-08-02 17:40:58 +00:00
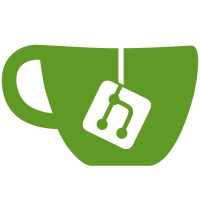
Added S_OK and S_FALSE modified Resources/ntstatus.xml Added all possible STATUS_WAIT_XX codes as STATUS_WAIT_0 + XX modified TechBot.Console/App.config Don't use hard-coded paths, please! modified TechBot.Library/ErrorCommand.cs New and improved !error command, now performs heuristics to catch all possible or likely uses modified TechBot.Library/HresultCommand.cs Removed useless field modified TechBot.Library/NumberParser.cs Made a couple of methods static modified TechBot.Library/TechBotService.cs Disable !api until it fails gracefully svn path=/trunk/; revision=23984
118 lines
3.4 KiB
C#
118 lines
3.4 KiB
C#
using System;
|
|
using System.Collections;
|
|
using System.IO;
|
|
using System.Data;
|
|
using System.Threading;
|
|
using TechBot.IRCLibrary;
|
|
|
|
namespace TechBot.Library
|
|
{
|
|
public class TechBotService
|
|
{
|
|
private IServiceOutput serviceOutput;
|
|
private string chmPath;
|
|
private string mainChm;
|
|
private string ntstatusXml;
|
|
private string winerrorXml;
|
|
private string hresultXml;
|
|
private string wmXml;
|
|
private string svnCommand;
|
|
private string bugUrl, WineBugUrl, SambaBugUrl;
|
|
private ArrayList commands = new ArrayList();
|
|
|
|
public TechBotService(IServiceOutput serviceOutput,
|
|
string chmPath,
|
|
string mainChm,
|
|
string ntstatusXml,
|
|
string winerrorXml,
|
|
string hresultXml,
|
|
string wmXml,
|
|
string svnCommand,
|
|
string bugUrl,
|
|
string WineBugUrl,
|
|
string SambaBugUrl)
|
|
{
|
|
this.serviceOutput = serviceOutput;
|
|
this.chmPath = chmPath;
|
|
this.mainChm = mainChm;
|
|
this.ntstatusXml = ntstatusXml;
|
|
this.winerrorXml = winerrorXml;
|
|
this.hresultXml = hresultXml;
|
|
this.wmXml = wmXml;
|
|
this.svnCommand = svnCommand;
|
|
this.bugUrl = bugUrl;
|
|
this.WineBugUrl = WineBugUrl;
|
|
this.SambaBugUrl = SambaBugUrl;
|
|
}
|
|
|
|
public void Run()
|
|
{
|
|
commands.Add(new HelpCommand(serviceOutput,
|
|
commands));
|
|
/*commands.Add(new ApiCommand(serviceOutput,
|
|
chmPath,
|
|
mainChm));*/
|
|
commands.Add(new NtStatusCommand(serviceOutput,
|
|
ntstatusXml));
|
|
commands.Add(new WinerrorCommand(serviceOutput,
|
|
winerrorXml));
|
|
commands.Add(new HresultCommand(serviceOutput,
|
|
hresultXml));
|
|
commands.Add(new ErrorCommand(serviceOutput,
|
|
ntstatusXml,
|
|
winerrorXml,
|
|
hresultXml));
|
|
commands.Add(new WmCommand(serviceOutput,
|
|
wmXml));
|
|
commands.Add(new SvnCommand(serviceOutput,
|
|
svnCommand));
|
|
commands.Add(new BugCommand(serviceOutput,
|
|
bugUrl,
|
|
WineBugUrl,
|
|
SambaBugUrl));
|
|
}
|
|
|
|
public void InjectMessage(MessageContext context,
|
|
string message)
|
|
{
|
|
if (message.StartsWith("!"))
|
|
ParseCommandMessage(context,
|
|
message);
|
|
}
|
|
|
|
private bool IsCommandMessage(string message)
|
|
{
|
|
return message.StartsWith("!");
|
|
}
|
|
|
|
public void ParseCommandMessage(MessageContext context,
|
|
string message)
|
|
{
|
|
if (!IsCommandMessage(message))
|
|
return;
|
|
|
|
message = message.Substring(1).Trim();
|
|
int index = message.IndexOf(' ');
|
|
string commandName;
|
|
string parameters = "";
|
|
if (index != -1)
|
|
{
|
|
commandName = message.Substring(0, index).Trim();
|
|
parameters = message.Substring(index).Trim();
|
|
}
|
|
else
|
|
commandName = message.Trim();
|
|
|
|
foreach (ICommand command in commands)
|
|
{
|
|
if (command.CanHandle(commandName))
|
|
{
|
|
command.Handle(context,
|
|
commandName, parameters);
|
|
return;
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|