mirror of
https://github.com/reactos/reactos.git
synced 2025-05-25 12:14:32 +00:00
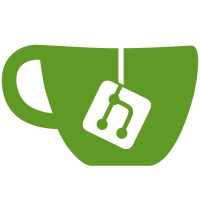
Imported from https://www.nuget.org/packages/Microsoft.Windows.SDK.CRTSource/10.0.22621.3 License: MIT
56 lines
1.3 KiB
C++
56 lines
1.3 KiB
C++
//
|
|
// cscanf.cpp
|
|
//
|
|
// Copyright (c) Microsoft Corporation. All rights reserved.
|
|
//
|
|
// The core formatted input functions for direct console I/O.
|
|
//
|
|
#define _ALLOW_OLD_VALIDATE_MACROS
|
|
#include <corecrt_internal_stdio_input.h>
|
|
|
|
using namespace __crt_stdio_input;
|
|
|
|
template <typename Character>
|
|
static int __cdecl common_cscanf(
|
|
unsigned __int64 const options,
|
|
Character const* const format,
|
|
_locale_t const locale,
|
|
va_list const arglist
|
|
) throw()
|
|
{
|
|
typedef input_processor<
|
|
Character,
|
|
console_input_adapter<Character>
|
|
> processor_type;
|
|
|
|
_LocaleUpdate locale_update(locale);
|
|
|
|
processor_type processor(
|
|
console_input_adapter<Character>(),
|
|
options,
|
|
format,
|
|
locale_update.GetLocaleT(),
|
|
arglist);
|
|
|
|
return processor.process();
|
|
}
|
|
|
|
extern "C" int __cdecl __conio_common_vcscanf(
|
|
unsigned __int64 const options,
|
|
char const* const format,
|
|
_locale_t const locale,
|
|
va_list const arglist
|
|
)
|
|
{
|
|
return common_cscanf(options, format, locale, arglist);
|
|
}
|
|
|
|
extern "C" int __cdecl __conio_common_vcwscanf(
|
|
unsigned __int64 const options,
|
|
wchar_t const* const format,
|
|
_locale_t const locale,
|
|
va_list const arglist
|
|
)
|
|
{
|
|
return common_cscanf(options, format, locale, arglist);
|
|
}
|