mirror of
https://github.com/reactos/reactos.git
synced 2024-07-11 23:25:09 +00:00
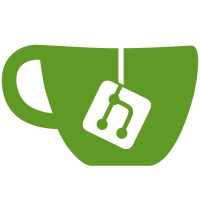
Add an include directory for all printing components containing definitions not found in the public headers. For now, that's just PackStrings in spoolss.h. [SPOOLSS_APITEST] Use the new spoolss.h header. [WINPRINT_APITEST] Add an API-Test for the winprint Print Processor, so far for its EnumPrintProcessorDatatypesW function. Tests succeed in Windows Server 2003. winspool.drv also provides functions that go into winprint.dll, but as these tests show, they behave slightly different in terms of error codes due to the involved RPC and routing. Windows Server 2003 has winprint functions in localspl.dll, so you have to copy its localspl.dll to winprint.dll for testing. [WINPRINT] - Use PackStrings to simplify the code. - Fix test failures. svn path=/branches/colins-printing-for-freedom/; revision=68025
82 lines
4.7 KiB
C
82 lines
4.7 KiB
C
/*
|
|
* PROJECT: ReactOS Standard Print Processor API Tests
|
|
* LICENSE: GNU GPLv2 or any later version as published by the Free Software Foundation
|
|
* PURPOSE: Tests for EnumPrintProcessorDatatypesW
|
|
* COPYRIGHT: Copyright 2015 Colin Finck <colin@reactos.org>
|
|
*/
|
|
|
|
#include <apitest.h>
|
|
|
|
#define WIN32_NO_STATUS
|
|
#include <windef.h>
|
|
#include <winbase.h>
|
|
#include <wingdi.h>
|
|
#include <winspool.h>
|
|
|
|
START_TEST(EnumPrintProcessorDatatypesW)
|
|
{
|
|
DWORD cbNeeded;
|
|
DWORD cbTemp;
|
|
DWORD dwReturned;
|
|
PDATATYPES_INFO_1W pDatatypesInfo1;
|
|
|
|
// Try with an invalid level. The error needs to be set by winspool, but not by the Print Processor.
|
|
SetLastError(0xDEADBEEF);
|
|
ok(!EnumPrintProcessorDatatypesW(NULL, NULL, 0, NULL, 0, NULL, NULL), "EnumPrintProcessorDatatypesW returns TRUE!\n");
|
|
ok(GetLastError() == 0xDEADBEEF, "EnumPrintProcessorDatatypesW returns error %lu!\n", GetLastError());
|
|
|
|
// Now try with valid level, but no pcbNeeded and no pcReturned. The error needs to be set by RPC.
|
|
SetLastError(0xDEADBEEF);
|
|
ok(!EnumPrintProcessorDatatypesW(NULL, NULL, 1, NULL, 0, NULL, NULL), "EnumPrintProcessorDatatypesW returns TRUE!\n");
|
|
ok(GetLastError() == 0xDEADBEEF, "EnumPrintProcessorDatatypesW returns error %lu!\n", GetLastError());
|
|
|
|
// Now try with pcbNeeded and pcReturned, but give no Print Processor. Show that winprint actually ignores the given Print Processor.
|
|
SetLastError(0xDEADBEEF);
|
|
ok(!EnumPrintProcessorDatatypesW(NULL, NULL, 1, NULL, 0, &cbNeeded, &dwReturned), "EnumPrintProcessorDatatypesW returns TRUE!\n");
|
|
ok(GetLastError() == ERROR_INSUFFICIENT_BUFFER, "EnumPrintProcessorDatatypesW returns error %lu!\n", GetLastError());
|
|
ok(cbNeeded > 0, "cbNeeded is 0!\n");
|
|
ok(dwReturned == 0, "dwReturned is %lu!\n", dwReturned);
|
|
|
|
// Same error has to occur when looking for an invalid Print Processor.
|
|
SetLastError(0xDEADBEEF);
|
|
ok(!EnumPrintProcessorDatatypesW(NULL, L"invalid", 1, NULL, 0, &cbNeeded, &dwReturned), "EnumPrintProcessorDatatypesW returns TRUE!\n");
|
|
ok(GetLastError() == ERROR_INSUFFICIENT_BUFFER, "EnumPrintProcessorDatatypesW returns error %lu!\n", GetLastError());
|
|
ok(cbNeeded > 0, "cbNeeded is 0!\n");
|
|
ok(dwReturned == 0, "dwReturned is %lu!\n", dwReturned);
|
|
|
|
// Now get the required buffer size by supplying all information. This needs to fail with ERROR_INSUFFICIENT_BUFFER.
|
|
SetLastError(0xDEADBEEF);
|
|
ok(!EnumPrintProcessorDatatypesW(NULL, L"winprint", 1, NULL, 0, &cbNeeded, &dwReturned), "EnumPrintProcessorDatatypesW returns TRUE!\n");
|
|
ok(GetLastError() == ERROR_INSUFFICIENT_BUFFER, "EnumPrintProcessorDatatypesW returns error %lu!\n", GetLastError());
|
|
ok(cbNeeded > 0, "cbNeeded is 0!\n");
|
|
ok(dwReturned == 0, "dwReturned is %lu!\n", dwReturned);
|
|
|
|
// Same error has to occur with a size to small.
|
|
SetLastError(0xDEADBEEF);
|
|
ok(!EnumPrintProcessorDatatypesW(NULL, L"winprint", 1, NULL, 1, &cbNeeded, &dwReturned), "EnumPrintProcessorDatatypesW returns TRUE!\n");
|
|
ok(GetLastError() == ERROR_INSUFFICIENT_BUFFER, "EnumPrintersW returns error %lu!\n", GetLastError());
|
|
ok(cbNeeded > 0, "cbNeeded is 0!\n");
|
|
ok(dwReturned == 0, "dwReturned is %lu!\n", dwReturned);
|
|
|
|
// Now provide the demanded size, but no buffer. Show that winprint returns a different error than the same function in winspool.
|
|
SetLastError(0xDEADBEEF);
|
|
ok(!EnumPrintProcessorDatatypesW(NULL, L"winprint", 1, NULL, cbNeeded, &cbTemp, &dwReturned), "EnumPrintProcessorDatatypesW returns TRUE!\n");
|
|
ok(GetLastError() == ERROR_INVALID_PARAMETER, "EnumPrintProcessorDatatypesW returns error %lu!\n", GetLastError());
|
|
ok(cbTemp == cbNeeded, "cbTemp is %lu!\n", cbTemp);
|
|
ok(dwReturned == 0, "dwReturned is %lu!\n", dwReturned);
|
|
|
|
// This also has to fail the same way when no Print Processor was given at all.
|
|
SetLastError(0xDEADBEEF);
|
|
ok(!EnumPrintProcessorDatatypesW(NULL, NULL, 1, NULL, cbNeeded, &cbTemp, &dwReturned), "EnumPrintProcessorDatatypesW returns TRUE!\n");
|
|
ok(GetLastError() == ERROR_INVALID_PARAMETER, "EnumPrintProcessorDatatypesW returns error %lu!\n", GetLastError());
|
|
ok(cbTemp == cbNeeded, "cbTemp is %lu!\n", cbTemp);
|
|
ok(dwReturned == 0, "dwReturned is %lu!\n", dwReturned);
|
|
|
|
// Finally use the function as intended and aim for success! Show that winprint doesn't modify the error code at all.
|
|
pDatatypesInfo1 = HeapAlloc(GetProcessHeap(), 0, cbNeeded);
|
|
SetLastError(0xDEADBEEF);
|
|
ok(EnumPrintProcessorDatatypesW(NULL, L"winprint", 1, (PBYTE)pDatatypesInfo1, cbNeeded, &cbNeeded, &dwReturned), "EnumPrintProcessorDatatypesW returns FALSE!\n");
|
|
ok(GetLastError() == 0xDEADBEEF, "EnumPrintProcessorDatatypesW returns error %lu!\n", GetLastError());
|
|
HeapFree(GetProcessHeap(), 0, pDatatypesInfo1);
|
|
}
|