mirror of
https://github.com/reactos/reactos.git
synced 2024-07-07 05:05:09 +00:00
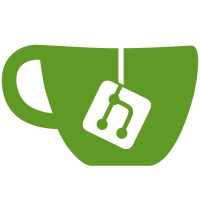
Added S_OK and S_FALSE modified Resources/ntstatus.xml Added all possible STATUS_WAIT_XX codes as STATUS_WAIT_0 + XX modified TechBot.Console/App.config Don't use hard-coded paths, please! modified TechBot.Library/ErrorCommand.cs New and improved !error command, now performs heuristics to catch all possible or likely uses modified TechBot.Library/HresultCommand.cs Removed useless field modified TechBot.Library/NumberParser.cs Made a couple of methods static modified TechBot.Library/TechBotService.cs Disable !api until it fails gracefully svn path=/trunk/; revision=23984
63 lines
1.2 KiB
C#
63 lines
1.2 KiB
C#
using System;
|
|
using System.Globalization;
|
|
|
|
namespace TechBot.Library
|
|
{
|
|
public class NumberParser
|
|
{
|
|
public bool Error = false;
|
|
|
|
private const string SpecialHexCharacters = "ABCDEF";
|
|
|
|
private static bool IsSpecialHexCharacter(char ch)
|
|
{
|
|
foreach (char specialChar in SpecialHexCharacters)
|
|
{
|
|
if (ch.ToString().ToUpper() == specialChar.ToString())
|
|
return true;
|
|
}
|
|
return false;
|
|
}
|
|
|
|
private static bool HasSpecialHexCharacters(string s)
|
|
{
|
|
foreach (char ch in s)
|
|
{
|
|
if (IsSpecialHexCharacter(ch))
|
|
return true;
|
|
}
|
|
return false;
|
|
}
|
|
|
|
public long Parse(string s)
|
|
{
|
|
try
|
|
{
|
|
Error = false;
|
|
bool useHex = false;
|
|
if (s.StartsWith("0x", StringComparison.InvariantCultureIgnoreCase))
|
|
{
|
|
s = s.Substring(2);
|
|
useHex = true;
|
|
}
|
|
if (HasSpecialHexCharacters(s))
|
|
useHex = true;
|
|
if (useHex)
|
|
return Int64.Parse(s,
|
|
NumberStyles.HexNumber);
|
|
else
|
|
return Int64.Parse(s);
|
|
}
|
|
catch (FormatException)
|
|
{
|
|
Error = true;
|
|
}
|
|
catch (OverflowException)
|
|
{
|
|
Error = true;
|
|
}
|
|
return -1;
|
|
}
|
|
}
|
|
}
|