mirror of
https://github.com/reactos/reactos.git
synced 2025-04-05 21:21:33 +00:00
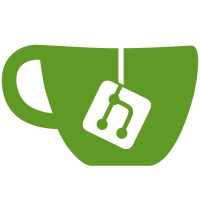
- Support loading protocols like XP - Support most of the relevant EditFlags - Support reading/writing the AlwaysShowExt and BrowserFlags properties - Loads the list much faster with optimized code and delaying the large icon and description string - Reduce the number of magic buffer sizes by replacing them with defines that are in the ballpark of being correct - Implemented column sorting - Removed custom icon extraction code - Removed IDS_FILE_EXT_TYPE string because it must be the same as IDS_ANY_FILE - Don't touch verb keys that are not edited to retain the original REG type - Don't clobber unchanged %1 icon location when editing a type CORE-19756
47 lines
1.2 KiB
C
47 lines
1.2 KiB
C
/*
|
|
* PROJECT: shell32
|
|
* LICENSE: LGPL-2.1+ (https://spdx.org/licenses/LGPL-2.1+)
|
|
* PURPOSE: Utility functions
|
|
* COPYRIGHT: ReactOS Team
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
inline BOOL
|
|
RegValueExists(HKEY hKey, LPCWSTR Name)
|
|
{
|
|
return RegQueryValueExW(hKey, Name, NULL, NULL, NULL, NULL) == ERROR_SUCCESS;
|
|
}
|
|
|
|
inline BOOL
|
|
RegKeyExists(HKEY hKey, LPCWSTR Path)
|
|
{
|
|
BOOL ret = !RegOpenKeyExW(hKey, Path, 0, MAXIMUM_ALLOWED, &hKey);
|
|
if (ret)
|
|
RegCloseKey(hKey);
|
|
return ret;
|
|
}
|
|
|
|
inline DWORD
|
|
RegSetOrDelete(HKEY hKey, LPCWSTR Name, DWORD Type, LPCVOID Data, DWORD Size)
|
|
{
|
|
if (Data)
|
|
return RegSetValueExW(hKey, Name, 0, Type, LPBYTE(Data), Size);
|
|
else
|
|
return RegDeleteValueW(hKey, Name);
|
|
}
|
|
|
|
inline DWORD
|
|
RegSetString(HKEY hKey, LPCWSTR Name, LPCWSTR Str, DWORD Type = REG_SZ)
|
|
{
|
|
return RegSetValueExW(hKey, Name, 0, Type, LPBYTE(Str), (lstrlenW(Str) + 1) * sizeof(WCHAR));
|
|
}
|
|
|
|
// SHExtractIconsW is a forward, use this function instead inside shell32
|
|
inline HICON
|
|
SHELL32_SHExtractIcon(LPCWSTR File, int Index, int cx, int cy)
|
|
{
|
|
HICON hIco;
|
|
int r = PrivateExtractIconsW(File, Index, cx, cy, &hIco, NULL, 1, 0);
|
|
return r > 0 ? hIco : NULL;
|
|
}
|