mirror of
https://github.com/reactos/reactos.git
synced 2024-07-02 02:34:53 +00:00
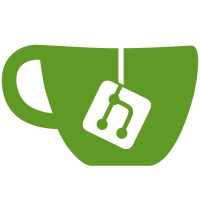
next few weeks. I'm going through the DFLAT api doc and fixing the export names and then going to try to build a simple hello dflat application. svn path=/trunk/; revision=2844
86 lines
1.7 KiB
C
86 lines
1.7 KiB
C
/* ------------- menu.c ------------- */
|
|
|
|
#include "dflat32/dflat.h"
|
|
|
|
static struct PopDown *FindCmd(MBAR *mn, int cmd)
|
|
{
|
|
MENU *mnu = mn->PullDown;
|
|
while (mnu->Title != (void *)-1) {
|
|
struct PopDown *pd = mnu->Selections;
|
|
while (pd->SelectionTitle != NULL) {
|
|
if (pd->ActionId == cmd)
|
|
return pd;
|
|
pd++;
|
|
}
|
|
mnu++;
|
|
}
|
|
return NULL;
|
|
}
|
|
|
|
char *GetCommandText(MBAR *mn, int cmd)
|
|
{
|
|
struct PopDown *pd = FindCmd(mn, cmd);
|
|
if (pd != NULL)
|
|
return pd->SelectionTitle;
|
|
return NULL;
|
|
}
|
|
|
|
BOOL isCascadedCommand(MBAR *mn, int cmd)
|
|
{
|
|
struct PopDown *pd = FindCmd(mn, cmd);
|
|
if (pd != NULL)
|
|
return pd->Attrib & CASCADED;
|
|
return FALSE;
|
|
}
|
|
|
|
void ActivateCommand(MBAR *mn, int cmd)
|
|
{
|
|
struct PopDown *pd = FindCmd(mn, cmd);
|
|
if (pd != NULL)
|
|
pd->Attrib &= ~INACTIVE;
|
|
}
|
|
|
|
void DeactivateCommand(MBAR *mn, int cmd)
|
|
{
|
|
struct PopDown *pd = FindCmd(mn, cmd);
|
|
if (pd != NULL)
|
|
pd->Attrib |= INACTIVE;
|
|
}
|
|
|
|
BOOL isActive(MBAR *mn, int cmd)
|
|
{
|
|
struct PopDown *pd = FindCmd(mn, cmd);
|
|
if (pd != NULL)
|
|
return !(pd->Attrib & INACTIVE);
|
|
return FALSE;
|
|
}
|
|
|
|
BOOL GetCommandToggle(MBAR *mn, int cmd)
|
|
{
|
|
struct PopDown *pd = FindCmd(mn, cmd);
|
|
if (pd != NULL)
|
|
return (pd->Attrib & CHECKED) != 0;
|
|
return FALSE;
|
|
}
|
|
|
|
void SetCommandToggle(MBAR *mn, int cmd)
|
|
{
|
|
struct PopDown *pd = FindCmd(mn, cmd);
|
|
if (pd != NULL)
|
|
pd->Attrib |= CHECKED;
|
|
}
|
|
|
|
void ClearCommandToggle(MBAR *mn, int cmd)
|
|
{
|
|
struct PopDown *pd = FindCmd(mn, cmd);
|
|
if (pd != NULL)
|
|
pd->Attrib &= ~CHECKED;
|
|
}
|
|
|
|
void InvertCommandToggle(MBAR *mn, int cmd)
|
|
{
|
|
struct PopDown *pd = FindCmd(mn, cmd);
|
|
if (pd != NULL)
|
|
pd->Attrib ^= CHECKED;
|
|
}
|