mirror of
https://github.com/reactos/reactos.git
synced 2024-11-01 04:11:30 +00:00
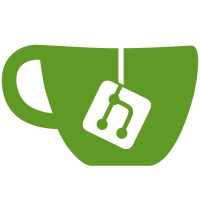
- Import cmdcnst.h and vga.h headers from the 'vga_new' VGA Miniport Driver, that contain definitions related to VGA registers as well as command-stream functionality. - Replace a bunch of hardcoded values by their corresponding defintions. - Replace "Captain-Obvious" comments in VgaIsPresent() with actual explanations from the corresponding function in 'vga_new'. - Simplify the VgaInterpretCmdStream() function, based on the corresponding one from 'vga_new'. - Use concise comments in the 'AT_Initialization' command stream definition. - Import the 'VGA_640x480' initialization command stream from 'vga_new' and use it as the full VGA initialization stream whenever the HAL does not handle the VGA display (HalResetDisplay() returning FALSE). Otherwise we just use the 'AT_Initialization' command stream that performs minimal initialization. - Remove unused AT_Initialization and other declarations from ARM build.
93 lines
3.3 KiB
C
93 lines
3.3 KiB
C
/*
|
|
* PROJECT: ReactOS VGA Miniport Driver
|
|
* LICENSE: Microsoft NT4 DDK Sample Code License
|
|
* PURPOSE: Command Code Definitions for VGA Command Streams
|
|
* PROGRAMMERS: Copyright (c) 1992 Microsoft Corporation
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
//--------------------------------------------------------------------------
|
|
// Definition of the set/clear mode command language.
|
|
//
|
|
// Each command is composed of a major portion and a minor portion.
|
|
// The major portion of a command can be found in the most significant
|
|
// nibble of a command byte, while the minor portion is in the least
|
|
// significant portion of a command byte.
|
|
//
|
|
// maj minor Description
|
|
// ---- ----- --------------------------------------------
|
|
// 00 End of data
|
|
//
|
|
// 10 in and out type commands as described by flags
|
|
// flags:
|
|
//
|
|
// xxxx
|
|
// ||||
|
|
// |||+-------- unused
|
|
// ||+--------- 0/1 single/multiple values to output (in's are always
|
|
// |+---------- 0/1 8/16 bit operation single)
|
|
// +----------- 0/1 out/in instruction
|
|
//
|
|
// Outs
|
|
// ----------------------------------------------
|
|
// 0 reg:W val:B
|
|
// 2 reg:W cnt:W val1:B val2:B...valN:B
|
|
// 4 reg:W val:W
|
|
// 6 reg:W cnt:W val1:W val2:W...valN:W
|
|
//
|
|
// Ins
|
|
// ----------------------------------------------
|
|
// 8 reg:W
|
|
// a reg:W cnt:W
|
|
// c reg:W
|
|
// e reg:W cnt:W
|
|
//
|
|
// 20 Special purpose outs
|
|
// 00 do indexed outs for seq, crtc, and gdc
|
|
// indexreg:W cnt:B startindex:B val1:B val2:B...valN:B
|
|
// 01 do indexed outs for atc
|
|
// index-data_reg:W cnt:B startindex:B val1:B val2:B...valN:B
|
|
// 02 do masked outs
|
|
// indexreg:W andmask:B xormask:B
|
|
//
|
|
// F0 Nop
|
|
//
|
|
//---------------------------------------------------------------------------
|
|
|
|
// some useful equates - major commands
|
|
|
|
#define EOD 0x000 // end of data
|
|
#define INOUT 0x010 // do ins or outs
|
|
#define METAOUT 0x020 // do special types of outs
|
|
#define NCMD 0x0f0 // Nop command
|
|
|
|
|
|
// flags for INOUT major command
|
|
|
|
//#define UNUSED 0x01 // reserved
|
|
#define MULTI 0x02 // multiple or single outs
|
|
#define BW 0x04 // byte/word size of operation
|
|
#define IO 0x08 // out/in instruction
|
|
|
|
// minor commands for METAOUT
|
|
|
|
#define INDXOUT 0x00 // do indexed outs
|
|
#define ATCOUT 0x01 // do indexed outs for atc
|
|
#define MASKOUT 0x02 // do masked outs using and-xor masks
|
|
|
|
|
|
// composite INOUT type commands
|
|
|
|
#define OB (INOUT) // output 8 bit value
|
|
#define OBM (INOUT+MULTI) // output multiple bytes
|
|
#define OW (INOUT+BW) // output single word value
|
|
#define OWM (INOUT+BW+MULTI) // output multiple words
|
|
|
|
#define IB (INOUT+IO) // input byte
|
|
#define IBM (INOUT+IO+MULTI) // input multiple bytes
|
|
#define IW (INOUT+IO+BW) // input word
|
|
#define IWM (INOUT+IO+BW+MULTI) // input multiple words
|
|
|
|
/* EOF */
|