mirror of
https://github.com/reactos/reactos.git
synced 2025-07-06 02:51:23 +00:00
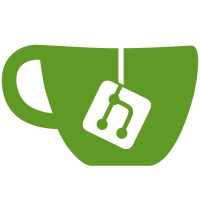
- made more and more easily extensible: * commands automatically loaded from plugins dlls * declarative and automatic command parameter parsing * common code moved to base classes - other fixes svn path=/trunk/; revision=33344
52 lines
1.2 KiB
C#
52 lines
1.2 KiB
C#
using System;
|
|
using System.Reflection;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
|
|
namespace TechBot.Library
|
|
{
|
|
public class CommandBuilder
|
|
{
|
|
private Type m_CommandType;
|
|
private string m_CommandName;
|
|
private string m_CommandHelp;
|
|
private string m_CommandDesc;
|
|
|
|
public CommandBuilder(Type commandType)
|
|
{
|
|
m_CommandType = commandType;
|
|
|
|
CommandAttribute commandAttribute = (CommandAttribute)
|
|
Attribute.GetCustomAttribute(commandType, typeof(CommandAttribute));
|
|
|
|
m_CommandName = commandAttribute.Name;
|
|
m_CommandHelp = commandAttribute.Help;
|
|
m_CommandDesc = commandAttribute.Description;
|
|
}
|
|
|
|
public string Name
|
|
{
|
|
get { return m_CommandName; }
|
|
}
|
|
|
|
public string Help
|
|
{
|
|
get { return m_CommandHelp; }
|
|
}
|
|
|
|
public string Description
|
|
{
|
|
get { return m_CommandDesc; }
|
|
}
|
|
|
|
public Type Type
|
|
{
|
|
get { return m_CommandType; }
|
|
}
|
|
|
|
public Command CreateCommand()
|
|
{
|
|
return (Command)Type.Assembly.CreateInstance(Type.FullName, true);
|
|
}
|
|
}
|
|
}
|