mirror of
https://github.com/reactos/reactos.git
synced 2024-08-29 06:38:52 +00:00
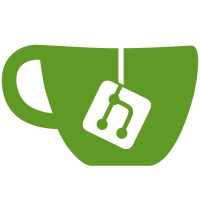
* [COMPILER_APITEST] Import MS EH/SEH tests Taken from https://github.com/microsoft/compiler-tests * [CRT] Add missing declaration of _longjmpex * [COMPILER_APITEST] Add cmake build files for MS SEH test It is built as a static library * [COMPILER_APITEST] Fix GCC build of MS SEH tests There are a number of hacks in there now. Also the volatile hacks should be separated and sent upstream. * [COMPILER_APITEST] Fix x64 build of MS SEH tests * [COMPILER_APITEST] Fix clang build of MS SEH tests * [COMPILER_APITEST] Include MS SEH tests
59 lines
1.1 KiB
C++
59 lines
1.1 KiB
C++
// Copyright (c) Microsoft. All rights reserved.
|
|
// Licensed under the MIT license. See LICENSE file in the project root for
|
|
// full license information.
|
|
|
|
#include <stdio.h>
|
|
|
|
typedef void (*OperationF)();
|
|
void TestFunction() { throw 1; }
|
|
void RethrowIt() { throw; }
|
|
|
|
int HandleRethrown1(OperationF perform) {
|
|
int ret = 0;
|
|
try {
|
|
perform();
|
|
return 0;
|
|
} catch (...) {
|
|
// ellipsis catch
|
|
try {
|
|
RethrowIt();
|
|
} catch (...) {
|
|
ret = 1;
|
|
}
|
|
}
|
|
|
|
return ret;
|
|
}
|
|
|
|
int HandleRethrown2(OperationF perform) {
|
|
int ret = 0;
|
|
try {
|
|
perform();
|
|
return 0;
|
|
} catch (int j) {
|
|
// non-ellipsis catch
|
|
try {
|
|
RethrowIt();
|
|
} catch (int i) {
|
|
ret = i;
|
|
}
|
|
}
|
|
|
|
return ret;
|
|
}
|
|
|
|
int main() {
|
|
int exit_code1;
|
|
int exit_code2;
|
|
exit_code1 = HandleRethrown1(TestFunction);
|
|
exit_code2 = HandleRethrown2(TestFunction);
|
|
|
|
if (exit_code1 == 1 && exit_code2 == 1) {
|
|
printf("passed");
|
|
} else {
|
|
printf("failed");
|
|
}
|
|
|
|
return 0;
|
|
}
|