mirror of
https://github.com/reactos/reactos.git
synced 2024-11-04 13:52:30 +00:00
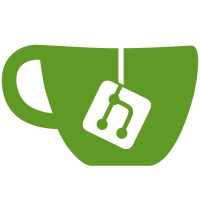
- Keep the directory lists only. - Don't remember file sizes and normal file paths. CORE-13950
93 lines
1.8 KiB
C++
93 lines
1.8 KiB
C++
#pragma once
|
|
|
|
#include <atlsimpcoll.h> // for CSimpleArray
|
|
|
|
//////////////////////////////////////////////////////////////////////////////
|
|
|
|
// A pathname with info
|
|
class CDirectoryItem
|
|
{
|
|
public:
|
|
CDirectoryItem() : m_pszPath(NULL)
|
|
{
|
|
}
|
|
|
|
CDirectoryItem(LPCWSTR pszPath)
|
|
{
|
|
m_pszPath = _wcsdup(pszPath);
|
|
}
|
|
|
|
CDirectoryItem(const CDirectoryItem& item)
|
|
: m_pszPath(_wcsdup(item.m_pszPath))
|
|
{
|
|
}
|
|
|
|
CDirectoryItem& operator=(const CDirectoryItem& item)
|
|
{
|
|
if (this != &item)
|
|
{
|
|
free(m_pszPath);
|
|
m_pszPath = _wcsdup(item.m_pszPath);
|
|
}
|
|
return *this;
|
|
}
|
|
|
|
~CDirectoryItem()
|
|
{
|
|
free(m_pszPath);
|
|
}
|
|
|
|
BOOL IsEmpty() const
|
|
{
|
|
return m_pszPath == NULL;
|
|
}
|
|
|
|
LPCWSTR GetPath() const
|
|
{
|
|
return m_pszPath;
|
|
}
|
|
|
|
void SetPath(LPCWSTR pszPath)
|
|
{
|
|
free(m_pszPath);
|
|
m_pszPath = _wcsdup(pszPath);
|
|
}
|
|
|
|
BOOL EqualPath(LPCWSTR pszPath) const
|
|
{
|
|
return m_pszPath != NULL && lstrcmpiW(m_pszPath, pszPath) == 0;
|
|
}
|
|
|
|
protected:
|
|
LPWSTR m_pszPath; // A full path, malloc'ed
|
|
};
|
|
|
|
// the directory list
|
|
class CDirectoryList
|
|
{
|
|
public:
|
|
CDirectoryList() : m_fRecursive(FALSE)
|
|
{
|
|
}
|
|
|
|
CDirectoryList(LPCWSTR pszDirectoryPath, BOOL fRecursive)
|
|
: m_fRecursive(fRecursive)
|
|
{
|
|
AddPathsFromDirectory(pszDirectoryPath);
|
|
}
|
|
|
|
BOOL ContainsPath(LPCWSTR pszPath) const;
|
|
BOOL AddPath(LPCWSTR pszPath);
|
|
BOOL AddPathsFromDirectory(LPCWSTR pszDirectoryPath);
|
|
BOOL RenamePath(LPCWSTR pszPath1, LPCWSTR pszPath2);
|
|
BOOL DeletePath(LPCWSTR pszPath);
|
|
|
|
void RemoveAll()
|
|
{
|
|
m_items.RemoveAll();
|
|
}
|
|
|
|
protected:
|
|
BOOL m_fRecursive;
|
|
CSimpleArray<CDirectoryItem> m_items;
|
|
};
|