mirror of
https://github.com/reactos/reactos.git
synced 2025-07-03 20:01:25 +00:00
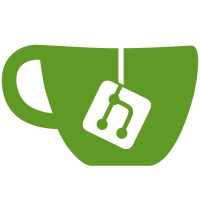
* subsys/win32k/include/callback.h: Fixed callback argument definitions. * subsys/win32k/ntuser/winpos.c: Implemented some more of the windows sizing/moving code. * subsys/win32k/ntuser/painting.c: Implemented some more of the window painting code. * subsys/win32k/objects/coord.c: Implemented LPtoDP and DPtoLP. * subsys/win32k/objects/region.c: Added stubs for some more region functions. 2002-07-04 David Welch <welch@computer2.darkstar.org> * ntoskrnl/ps/process.c (NtCreateProcess): Duplicate the process desktop handle as well. 2002-07-04 David Welch <welch@computer2.darkstar.org> * ntoskrnl/se/token.c: Don't call the ZwXXX variant of system calls when in system context. 2002-07-04 David Welch <welch@computer2.darkstar.org> * ntoskrnl/Makefile: Added file with MDA output code. * ntoskrnl/kd/kdebug.c: Recognize MDA as a destination for debug output. 2002-07-04 David Welch <welch@computer2.darkstar.org> * lib/user32/windows/defwnd.c: Implemented some more of the default window handler. 2002-07-04 David Welch <welch@computer2.darkstar.org> * lib/user32/misc/stubs.c: Removed some stubs to seperate files. 2002-07-04 David Welch <welch@computer2.darkstar.org> * lib/user32/user32.def: Export ScreenToClient otherwise we get problems when code in user32 tries to call it. 2002-07-04 David Welch <welch@computer2.darkstar.org> * include/win32k/region.h: Added prototypes for some missing region functions. 2002-07-04 David Welch <welch@computer2.darkstar.org> * include/win32k/ntuser.h: Added prototypes for some missing NtUserXXX functions. 2002-07-04 David Welch <welch@computer2.darkstar.org> * include/user32/wininternal.h: Added some constants for private GetDCEx styles that WINE needs. 2002-07-04 David Welch <welch@computer2.darkstar.org> * include/user32/callback.h: Fixed callbacks for messages with parameters. 2002-07-04 David Welch <welch@computer2.darkstar.org> * include/napi/win32.h (W32THREAD): Added pointer to the thread's desktop. * include/napi/win32.h (W32PROCESS): Removed handle table, added a pointer to the process's window station. * subsys/win32k/ntuser/guicheck.c (W32kGuiCheck): Reference a process's window station on the first win32k system call. Reference a thread's desktop on the first win32k system call. 2002-07-04 David Welch <welch@computer2.darkstar.org> * include/messages.h: Added some missing WM_XXX constants. 2002-07-04 David Welch <welch@computer2.darkstar.org> * drivers/dd/ide/makefile: Compiling with debugging messages needs libgcc to be linked in. 2002-07-04 David Welch <welch@computer2.darkstar.org> * iface/addsys/genw32k.c: Generate a variable with the number of system calls. * iface/native/genntdll.c: Generate a proper stack frame for the user system call stubs. * ntoskrnl/ke/i386/syscall.S: Generate a proper stack frame for the handler for system calls. 2002-07-04 David Welch <welch@computer2.darkstar.org> * Makefile: Build the GUI startup application. * subsys/system/gstart/gstart.c: Application to start up the GUI. svn path=/trunk/; revision=3179
111 lines
2.9 KiB
C
111 lines
2.9 KiB
C
#ifndef __WIN32K_MSGQUEUE_H
|
|
#define __WIN32K_MSGQUEUE_H
|
|
|
|
#include <windows.h>
|
|
|
|
typedef struct _USER_MESSAGE
|
|
{
|
|
LIST_ENTRY ListEntry;
|
|
MSG Msg;
|
|
} USER_MESSAGE, *PUSER_MESSAGE;
|
|
|
|
struct _USER_MESSAGE_QUEUE;
|
|
|
|
typedef struct _USER_SENT_MESSAGE
|
|
{
|
|
LIST_ENTRY ListEntry;
|
|
MSG Msg;
|
|
PKEVENT CompletionEvent;
|
|
LRESULT* Result;
|
|
struct _USER_MESSAGE_QUEUE* CompletionQueue;
|
|
SENDASYNCPROC CompletionCallback;
|
|
ULONG_PTR CompletionCallbackContext;
|
|
} USER_SENT_MESSAGE, *PUSER_SENT_MESSAGE;
|
|
|
|
typedef struct _USER_SENT_MESSAGE_NOTIFY
|
|
{
|
|
SENDASYNCPROC CompletionCallback;
|
|
ULONG_PTR CompletionCallbackContext;
|
|
LRESULT Result;
|
|
HWND hWnd;
|
|
UINT Msg;
|
|
LIST_ENTRY ListEntry;
|
|
} USER_SENT_MESSAGE_NOTIFY, *PUSER_SENT_MESSAGE_NOTIFY;
|
|
|
|
typedef struct _USER_MESSAGE_QUEUE
|
|
{
|
|
/* Queue of messages sent to the queue. */
|
|
LIST_ENTRY SentMessagesListHead;
|
|
/* Queue of messages posted to the queue. */
|
|
LIST_ENTRY PostedMessagesListHead;
|
|
/* Queue of hardware messages for the queue. */
|
|
LIST_ENTRY HardwareMessagesListHead;
|
|
/* Queue of sent-message notifies for the queue. */
|
|
LIST_ENTRY NotifyMessagesListHead;
|
|
/* Lock for the queue. */
|
|
FAST_MUTEX Lock;
|
|
/* True if a WM_QUIT message is pending. */
|
|
BOOLEAN QuitPosted;
|
|
/* The quit exit code. */
|
|
ULONG QuitExitCode;
|
|
/* Set if there are new messages in any of the queues. */
|
|
KEVENT NewMessages;
|
|
/* FIXME: Unknown. */
|
|
ULONG QueueStatus;
|
|
/* Current window with focus for this queue. */
|
|
HWND FocusWindow;
|
|
/* True if a window needs painting. */
|
|
BOOLEAN PaintPosted;
|
|
/* Count of paints pending. */
|
|
ULONG PaintCount;
|
|
} USER_MESSAGE_QUEUE, *PUSER_MESSAGE_QUEUE;
|
|
|
|
VOID
|
|
MsqSendMessage(PUSER_MESSAGE_QUEUE MessageQueue,
|
|
PUSER_SENT_MESSAGE Message);
|
|
VOID
|
|
MsqInitializeMessage(PUSER_MESSAGE Message,
|
|
LPMSG Msg);
|
|
PUSER_MESSAGE
|
|
MsqCreateMessage(LPMSG Msg);
|
|
VOID
|
|
MsqDestroyMessage(PUSER_MESSAGE Message);
|
|
VOID
|
|
MsqPostMessage(PUSER_MESSAGE_QUEUE MessageQueue,
|
|
PUSER_MESSAGE Message,
|
|
BOOLEAN Hardware);
|
|
BOOLEAN
|
|
MsqFindMessage(IN PUSER_MESSAGE_QUEUE MessageQueue,
|
|
IN BOOLEAN Hardware,
|
|
IN BOOLEAN Remove,
|
|
IN HWND Wnd,
|
|
IN UINT MsgFilterLow,
|
|
IN UINT MsgFilterHigh,
|
|
OUT PUSER_MESSAGE* Message);
|
|
VOID
|
|
MsqInitializeMessageQueue(PUSER_MESSAGE_QUEUE MessageQueue);
|
|
VOID
|
|
MsqFreeMessageQueue(PUSER_MESSAGE_QUEUE MessageQueue);
|
|
PUSER_MESSAGE_QUEUE
|
|
MsqCreateMessageQueue(VOID);
|
|
VOID
|
|
MsqDestroyMessageQueue(PUSER_MESSAGE_QUEUE MessageQueue);
|
|
PUSER_MESSAGE_QUEUE
|
|
MsqGetHardwareMessageQueue(VOID);
|
|
NTSTATUS
|
|
MsqWaitForNewMessage(PUSER_MESSAGE_QUEUE MessageQueue);
|
|
NTSTATUS
|
|
MsqInitializeImpl(VOID);
|
|
BOOLEAN
|
|
MsqDispatchOneSentMessage(PUSER_MESSAGE_QUEUE MessageQueue);
|
|
NTSTATUS
|
|
MsqWaitForNewMessages(PUSER_MESSAGE_QUEUE MessageQueue);
|
|
VOID
|
|
MsqSendNotifyMessage(PUSER_MESSAGE_QUEUE MessageQueue,
|
|
PUSER_SENT_MESSAGE_NOTIFY NotifyMessage);
|
|
|
|
#define MAKE_LONG(x, y) ((((y) & 0xFFFF) << 16) | ((x) & 0xFFFF))
|
|
|
|
#endif /* __WIN32K_MSGQUEUE_H */
|
|
|
|
/* EOF */
|