mirror of
https://github.com/reactos/reactos.git
synced 2024-11-01 12:26:32 +00:00
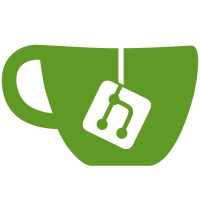
Use new-style file open/save-as dialog. - Add OFN_EXPLORER flag to OPENFILENAME structure in notepad, wordpad, mspaint, clipbrd, mmc, mplay32, mscutils, regedit, winhlp32, progman, shellbtrfs, cryptui, shell32, setupapi, vgafontedit, infinst, and vfdlib modules.
77 lines
1.6 KiB
C
77 lines
1.6 KiB
C
/*
|
|
* PROJECT: ReactOS VGA Font Editor
|
|
* LICENSE: GPL-2.0+ (https://spdx.org/licenses/GPL-2.0+)
|
|
* PURPOSE: Functions for opening and saving files
|
|
* COPYRIGHT: Copyright 2008 Colin Finck (colin@reactos.org)
|
|
*/
|
|
|
|
#include "precomp.h"
|
|
|
|
static OPENFILENAMEW ofn;
|
|
|
|
VOID
|
|
FileInitialize(IN HWND hwnd)
|
|
{
|
|
ZeroMemory( &ofn, sizeof(ofn) );
|
|
ofn.lStructSize = sizeof(ofn);
|
|
ofn.hwndOwner = hwnd;
|
|
ofn.nMaxFile = MAX_PATH;
|
|
ofn.lpstrDefExt = L"bin";
|
|
}
|
|
|
|
static __inline VOID
|
|
PrepareFilter(IN PWSTR pszFilter)
|
|
{
|
|
// RC strings can't be double-null terminated, so we use | instead to separate the entries.
|
|
// Convert them back to null characters here.
|
|
do
|
|
{
|
|
if(*pszFilter == '|')
|
|
*pszFilter = 0;
|
|
}
|
|
while(*++pszFilter);
|
|
}
|
|
|
|
BOOL
|
|
DoOpenFile(OUT PWSTR pszFileName)
|
|
{
|
|
BOOL bRet;
|
|
PWSTR pszFilter;
|
|
|
|
if( AllocAndLoadString(&pszFilter, IDS_OPENFILTER) )
|
|
{
|
|
PrepareFilter(pszFilter);
|
|
ofn.lpstrFilter = pszFilter;
|
|
ofn.lpstrFile = pszFileName;
|
|
ofn.Flags = OFN_EXPLORER | OFN_FILEMUSTEXIST;
|
|
|
|
bRet = GetOpenFileNameW(&ofn);
|
|
HeapFree(hProcessHeap, 0, pszFilter);
|
|
|
|
return bRet;
|
|
}
|
|
|
|
return FALSE;
|
|
}
|
|
|
|
BOOL
|
|
DoSaveFile(IN OUT PWSTR pszFileName)
|
|
{
|
|
BOOL bRet;
|
|
PWSTR pszFilter;
|
|
|
|
if( AllocAndLoadString(&pszFilter, IDS_SAVEFILTER) )
|
|
{
|
|
PrepareFilter(pszFilter);
|
|
ofn.lpstrFilter = pszFilter;
|
|
ofn.lpstrFile = pszFileName;
|
|
|
|
bRet = GetSaveFileNameW(&ofn);
|
|
HeapFree(hProcessHeap, 0, pszFilter);
|
|
|
|
return bRet;
|
|
}
|
|
|
|
return FALSE;
|
|
}
|