mirror of
https://github.com/reactos/reactos.git
synced 2024-06-01 02:01:57 +00:00
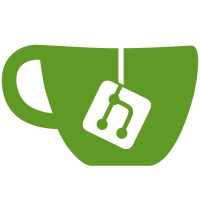
- Implement caching of individual (newline-separated) text lines; this behaviour can be enabled with a flag (enabled by MORE): CON_PAGER_CACHE_INCOMPLETE_LINE. This feature is necessary when reading a text file, whose text lines may span across two or more successive temporary read buffers, and is required for correctly determining whether the lines being read are blank and may be squeezed. - When squeezing blank lines, the blank-line check must be done for each line segment corresponding to the screen line (and following) that need to be displayed. This matches the behaviour of MS MORE.COM. - Fix the IsBlankLine() check to not consider FORM-FEEDs as being blank characters: This is necessary for correctly handling FORM-FEED expansion. Also note that MS MORE.COM only checks for spaces and TABs, so we are slightly overdoing these checks (considering other types of whitespace). - Get rid of ConCallPagerLine() and the intermediate CON_PAGER_DONT_OUTPUT state flag that were used repeatedly for each and every small line chunks. Instead, call directly the user-specified 'PagerLine' callback when we are about to start treating the next line segment to be displayed (see comment above). - Fix the exit return condition of ConPagerWorker(): it should return TRUE whenever we displayed all the required lines, and FALSE otherwise. Otherwise, the previous (buggy) condition on the data being read from the text file, may lead to the prompt not showing when a screenful of text has been displayed, if it happened that the current text buffer becomes empty at the same time (even if, overall, the text file hasn't been fully displayed). - In MorePagerLine(), when we encounter for the first time a blank line that will be squeezed with other successive ones, display a single blank line. But for that, just display one space and a newline: this single space is especially needed in order to force line wrapping when the ENABLE_VIRTUAL_TERMINAL_PROCESSING or DISABLE_NEWLINE_AUTO_RETURN console modes are enabled. Otherwise the cursor remains at the previous line (without wrapping), and just outputting one newline will not make it move past 2 lines as one would naively expect.
125 lines
3 KiB
C
125 lines
3 KiB
C
/*
|
|
* PROJECT: ReactOS Console Utilities Library
|
|
* LICENSE: GPL-2.0+ (https://spdx.org/licenses/GPL-2.0+)
|
|
* PURPOSE: Console/terminal paging functionality.
|
|
* COPYRIGHT: Copyright 2017-2021 Hermes Belusca-Maito
|
|
* Copyright 2021 Katayama Hirofumi MZ
|
|
*/
|
|
|
|
/**
|
|
* @file pager.h
|
|
* @ingroup ConUtils
|
|
*
|
|
* @brief Console/terminal paging functionality.
|
|
**/
|
|
|
|
#ifndef __PAGER_H__
|
|
#define __PAGER_H__
|
|
|
|
#pragma once
|
|
|
|
#ifndef _UNICODE
|
|
#error The ConUtils library at the moment only supports compilation with _UNICODE defined!
|
|
#endif
|
|
|
|
#ifdef __cplusplus
|
|
extern "C" {
|
|
#endif
|
|
|
|
// #include <wincon.h>
|
|
|
|
struct _CON_PAGER;
|
|
|
|
typedef BOOL
|
|
(__stdcall *CON_PAGER_LINE_FN)(
|
|
IN OUT struct _CON_PAGER *Pager,
|
|
IN PCTCH line,
|
|
IN DWORD cch);
|
|
|
|
/* Flags for CON_PAGER */
|
|
#define CON_PAGER_EXPAND_TABS (1 << 0)
|
|
#define CON_PAGER_EXPAND_FF (1 << 1)
|
|
// Whether or not the pager will cache the line if it's incomplete (not NEWLINE-terminated).
|
|
#define CON_PAGER_CACHE_INCOMPLETE_LINE (1 << 2)
|
|
|
|
typedef struct _CON_PAGER
|
|
{
|
|
/* Console screen properties */
|
|
PCON_SCREEN Screen;
|
|
DWORD PageColumns;
|
|
DWORD PageRows;
|
|
|
|
/* Paging parameters */
|
|
CON_PAGER_LINE_FN PagerLine; /* The line function */
|
|
DWORD dwFlags; /* The CON_PAGER_... flags */
|
|
LONG nTabWidth;
|
|
DWORD ScrollRows;
|
|
|
|
/* Data buffer */
|
|
PCTCH CachedLine; /* Cached line, HeapAlloc'ated */
|
|
SIZE_T cchCachedLine; /* Its length (number of characters) */
|
|
SIZE_T ich; /* The current index of character in TextBuff (a user-provided source buffer) */
|
|
|
|
/* Paging state */
|
|
PCTCH CurrentLine; /* Pointer to the current line (either within a user-provided source buffer, or to CachedLine) */
|
|
SIZE_T ichCurr; /* The current index of character in CurrentLine */
|
|
SIZE_T iEndLine; /* End (length) of CurrentLine */
|
|
DWORD nSpacePending; /* Pending spaces for TAB expansion */
|
|
DWORD iColumn; /* The current index of column */
|
|
DWORD iLine; /* The physical output line count of screen */
|
|
DWORD lineno; /* The logical line number */
|
|
} CON_PAGER, *PCON_PAGER;
|
|
|
|
#define INIT_CON_PAGER(pScreen) {(pScreen), 0}
|
|
|
|
#define InitializeConPager(pPager, pScreen) \
|
|
do { \
|
|
ZeroMemory((pPager), sizeof(*(pPager))); \
|
|
(pPager)->Screen = (pScreen); \
|
|
} while (0)
|
|
|
|
|
|
typedef BOOL
|
|
(__stdcall *PAGE_PROMPT)(
|
|
IN PCON_PAGER Pager,
|
|
IN DWORD Done,
|
|
IN DWORD Total);
|
|
|
|
BOOL
|
|
ConWritePaging(
|
|
IN PCON_PAGER Pager,
|
|
IN PAGE_PROMPT PagePrompt,
|
|
IN BOOL StartPaging,
|
|
IN PCTCH szStr,
|
|
IN DWORD len);
|
|
|
|
BOOL
|
|
ConPutsPaging(
|
|
IN PCON_PAGER Pager,
|
|
IN PAGE_PROMPT PagePrompt,
|
|
IN BOOL StartPaging,
|
|
IN PCTSTR szStr);
|
|
|
|
BOOL
|
|
ConResPagingEx(
|
|
IN PCON_PAGER Pager,
|
|
IN PAGE_PROMPT PagePrompt,
|
|
IN BOOL StartPaging,
|
|
IN HINSTANCE hInstance OPTIONAL,
|
|
IN UINT uID);
|
|
|
|
BOOL
|
|
ConResPaging(
|
|
IN PCON_PAGER Pager,
|
|
IN PAGE_PROMPT PagePrompt,
|
|
IN BOOL StartPaging,
|
|
IN UINT uID);
|
|
|
|
#ifdef __cplusplus
|
|
}
|
|
#endif
|
|
|
|
#endif /* __PAGER_H__ */
|
|
|
|
/* EOF */
|