mirror of
https://github.com/reactos/reactos.git
synced 2024-07-05 12:15:46 +00:00
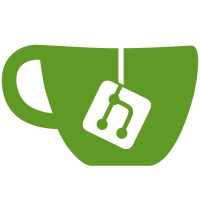
- Add support for recovering from crashed tests - Add check to prevent us from running the test every boot - Delete some useless code - Record test result information in the registry - Under the Kmtest\Parameters key, you will find CurrentStage which is the stage that testing is on (almost always 8 if it boots). You will also find <Test Name>SuccessCount which is the number of successful tests, <Test Name>FailureCount which is the number of failed tests, <Test Name>TotalCount which is the total number of tests, and <Test Name>SkippedCount which is the number of tests that have been skipped - Enjoy your reg testing! :) svn path=/trunk/; revision=47309
142 lines
4.3 KiB
C
142 lines
4.3 KiB
C
/*
|
|
* PnP Test
|
|
* Device Interface functions test
|
|
*
|
|
* Copyright 2004 Filip Navara <xnavara@volny.cz>
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Library General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2 of the License, or (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Library General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Library General Public
|
|
* License along with this library; see the file COPYING.LIB.
|
|
* If not, write to the Free Software Foundation,
|
|
* 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
|
|
*/
|
|
|
|
/* INCLUDES *******************************************************************/
|
|
|
|
#include <ddk/ntifs.h>
|
|
#include <ndk/iotypes.h>
|
|
#include "kmtest.h"
|
|
|
|
#define NDEBUG
|
|
#include "debug.h"
|
|
|
|
/* PRIVATE FUNCTIONS **********************************************************/
|
|
|
|
NTSTATUS
|
|
(NTAPI *IoGetDeviceInterfaces_Func)(
|
|
IN CONST GUID *InterfaceClassGuid,
|
|
IN PDEVICE_OBJECT PhysicalDeviceObject OPTIONAL,
|
|
IN ULONG Flags,
|
|
OUT PWSTR *SymbolicLinkList);
|
|
|
|
NTSTATUS NTAPI
|
|
ReactOS_IoGetDeviceInterfaces(
|
|
IN CONST GUID *InterfaceClassGuid,
|
|
IN PDEVICE_OBJECT PhysicalDeviceObject OPTIONAL,
|
|
IN ULONG Flags,
|
|
OUT PWSTR *SymbolicLinkList);
|
|
|
|
VOID DeviceInterfaceTest_Func()
|
|
{
|
|
NTSTATUS Status;
|
|
PWSTR SymbolicLinkList;
|
|
PWSTR SymbolicLinkListPtr;
|
|
GUID Guid = {0x378de44c, 0x56ef, 0x11d1, {0xbc, 0x8c, 0x00, 0xa0, 0xc9, 0x14, 0x05, 0xdd}};
|
|
|
|
Status = IoGetDeviceInterfaces(
|
|
&Guid,
|
|
NULL,
|
|
0,
|
|
&SymbolicLinkList);
|
|
|
|
ok(NT_SUCCESS(Status),
|
|
"IoGetDeviceInterfaces failed with status 0x%X\n",
|
|
(unsigned int)Status);
|
|
if (!NT_SUCCESS(Status))
|
|
{
|
|
return;
|
|
}
|
|
|
|
DPRINT("IoGetDeviceInterfaces results:\n");
|
|
for (SymbolicLinkListPtr = SymbolicLinkList;
|
|
SymbolicLinkListPtr[0] != 0 && SymbolicLinkListPtr[1] != 0;
|
|
SymbolicLinkListPtr += wcslen(SymbolicLinkListPtr) + 1)
|
|
{
|
|
DPRINT1("Symbolic Link: %S\n", SymbolicLinkListPtr);
|
|
}
|
|
|
|
#if 0
|
|
DPRINT("[PnP Test] Trying to get aliases\n");
|
|
|
|
for (SymbolicLinkListPtr = SymbolicLinkList;
|
|
SymbolicLinkListPtr[0] != 0 && SymbolicLinkListPtr[1] != 0;
|
|
SymbolicLinkListPtr += wcslen(SymbolicLinkListPtr) + 1)
|
|
{
|
|
UNICODE_STRING SymbolicLink;
|
|
UNICODE_STRING AliasSymbolicLink;
|
|
|
|
SymbolicLink.Buffer = SymbolicLinkListPtr;
|
|
SymbolicLink.Length = SymbolicLink.MaximumLength = wcslen(SymbolicLinkListPtr);
|
|
RtlInitUnicodeString(&AliasSymbolicLink, NULL);
|
|
IoGetDeviceInterfaceAlias(
|
|
&SymbolicLink,
|
|
&AliasGuid,
|
|
&AliasSymbolicLink);
|
|
if (AliasSymbolicLink.Buffer != NULL)
|
|
{
|
|
DPRINT("[PnP Test] Original: %S\n", SymbolicLinkListPtr);
|
|
DPRINT("[PnP Test] Alias: %S\n", AliasSymbolicLink.Buffer);
|
|
}
|
|
}
|
|
#endif
|
|
|
|
ExFreePool(SymbolicLinkList);
|
|
}
|
|
|
|
VOID RegisterDI_Test(HANDLE KeyHandle)
|
|
{
|
|
GUID Guid = {0x378de44c, 0x56ef, 0x11d1, {0xbc, 0x8c, 0x00, 0xa0, 0xc9, 0x14, 0x05, 0xdd}};
|
|
DEVICE_OBJECT DeviceObject;
|
|
EXTENDED_DEVOBJ_EXTENSION DeviceObjectExtension;
|
|
DEVICE_NODE DeviceNode;
|
|
UNICODE_STRING SymbolicLinkName;
|
|
NTSTATUS Status;
|
|
|
|
StartTest();
|
|
|
|
RtlInitUnicodeString(&SymbolicLinkName, L"");
|
|
|
|
// Prepare our surrogate of a Device Object
|
|
DeviceObject.DeviceObjectExtension = (PDEVOBJ_EXTENSION)&DeviceObjectExtension;
|
|
|
|
// 1. DeviceNode = NULL
|
|
DeviceObjectExtension.DeviceNode = NULL;
|
|
Status = IoRegisterDeviceInterface(&DeviceObject, &Guid, NULL,
|
|
&SymbolicLinkName);
|
|
|
|
ok(Status == STATUS_INVALID_DEVICE_REQUEST,
|
|
"IoRegisterDeviceInterface returned 0x%08lX\n", Status);
|
|
|
|
// 2. DeviceNode->InstancePath is of a null length
|
|
DeviceObjectExtension.DeviceNode = &DeviceNode;
|
|
DeviceNode.InstancePath.Length = 0;
|
|
Status = IoRegisterDeviceInterface(&DeviceObject, &Guid, NULL,
|
|
&SymbolicLinkName);
|
|
|
|
ok(Status == STATUS_INVALID_DEVICE_REQUEST,
|
|
"IoRegisterDeviceInterface returned 0x%08lX\n", Status);
|
|
|
|
DeviceInterfaceTest_Func();
|
|
|
|
FinishTest(KeyHandle, L"IoDeviceInterfaceTest");
|
|
}
|