mirror of
https://github.com/reactos/reactos.git
synced 2024-07-05 04:06:22 +00:00
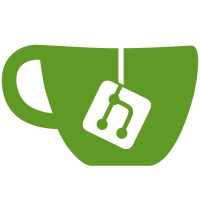
CORE-18221 Load the DirectX graphics kernel driver (dxg.sys) by win32k at WINSRV initialization time, in NtUserInitialize(). Keep it always loaded in memory, as on Windows, instead of loading it only by DirectX dlls. This fixes the problem of acessing this driver: we need only to call DxDdEnableDirectDraw() and do other stuff when DirectDraw/Direct3D is required by anything. In other cases, it is called from win32k PDEV functions when changing display mode (as in Windows). Since it's used by other things too, it needs to be always loaded. Otherwise, if it's not loaded, its APIs are not accessible when needed, and execution fails. For example, it fixes display mode change problem in VMWare, when a new mode fails to be applied. Indeed, when it manages DirectDraw stuff, it calls DXG routines, and therefore fails if dxg.sys isn't loaded in memory at this moment. - Implement InitializeGreCSRSS() initialization routine, that initializes supplemental NTGDI/GRE data once CSRSS and WINSRV are loaded: * Call DxDdStartupDxGraphics() inside it, which loads dxg.sys. * Additionally, move fonts and language ID initialization there, from win32k!DriverEntry. Confirmed by analysis on Windows. - Call InitializeGreCSRSS() in NtUserInitialize() main initialization routine (called by WINSRV initialization). Moved to NTGDI from previously NTUSER place: Co-authored-by: Hermès Bélusca-Maïto <hermes.belusca-maito@reactos.org>
147 lines
3.2 KiB
C
147 lines
3.2 KiB
C
#pragma once
|
|
|
|
typedef struct INTENG_ENTER_LEAVE_TAG
|
|
{
|
|
/* Contents is private to EngEnter/EngLeave */
|
|
SURFOBJ *DestObj;
|
|
SURFOBJ *OutputObj;
|
|
HBITMAP OutputBitmap;
|
|
CLIPOBJ *TrivialClipObj;
|
|
RECTL DestRect;
|
|
BOOL ReadOnly;
|
|
} INTENG_ENTER_LEAVE, *PINTENG_ENTER_LEAVE;
|
|
|
|
extern BOOL APIENTRY IntEngEnter(PINTENG_ENTER_LEAVE EnterLeave,
|
|
SURFOBJ *DestObj,
|
|
RECTL *DestRect,
|
|
BOOL ReadOnly,
|
|
POINTL *Translate,
|
|
SURFOBJ **OutputObj);
|
|
|
|
extern BOOL APIENTRY IntEngLeave(PINTENG_ENTER_LEAVE EnterLeave);
|
|
|
|
extern HGDIOBJ StockObjects[];
|
|
extern USHORT gusLanguageID;
|
|
|
|
BOOL InitializeGreCSRSS(VOID);
|
|
USHORT FASTCALL UserGetLanguageID(VOID);
|
|
|
|
PVOID APIENTRY HackSecureVirtualMemory(IN PVOID,IN SIZE_T,IN ULONG,OUT PVOID *);
|
|
VOID APIENTRY HackUnsecureVirtualMemory(IN PVOID);
|
|
|
|
NTSTATUS
|
|
NTAPI
|
|
RegOpenKey(
|
|
LPCWSTR pwszKeyName,
|
|
PHKEY phkey);
|
|
|
|
NTSTATUS
|
|
NTAPI
|
|
RegQueryValue(
|
|
IN HKEY hkey,
|
|
IN PCWSTR pwszValueName,
|
|
IN ULONG ulType,
|
|
OUT PVOID pvData,
|
|
IN OUT PULONG pcbValue);
|
|
|
|
VOID
|
|
NTAPI
|
|
RegWriteSZ(HKEY hkey, PWSTR pwszValue, PWSTR pwszData);
|
|
|
|
VOID
|
|
NTAPI
|
|
RegWriteDWORD(HKEY hkey, PWSTR pwszValue, DWORD dwData);
|
|
|
|
BOOL
|
|
NTAPI
|
|
RegReadDWORD(HKEY hkey, PWSTR pwszValue, PDWORD pdwData);
|
|
|
|
DWORD
|
|
NTAPI
|
|
RegGetSectionDWORD(LPCWSTR pszSection, LPWSTR pszValue, DWORD dwDefault);
|
|
|
|
VOID FASTCALL
|
|
SetLastNtError(
|
|
NTSTATUS Status);
|
|
|
|
typedef struct _GDI_POOL *PGDI_POOL;
|
|
|
|
PGDI_POOL
|
|
NTAPI
|
|
GdiPoolCreate(
|
|
ULONG cjAllocSize,
|
|
ULONG ulTag);
|
|
|
|
VOID
|
|
NTAPI
|
|
GdiPoolDestroy(PGDI_POOL pPool);
|
|
|
|
PVOID
|
|
NTAPI
|
|
GdiPoolAllocate(
|
|
PGDI_POOL pPool);
|
|
|
|
VOID
|
|
NTAPI
|
|
GdiPoolFree(
|
|
PGDI_POOL pPool,
|
|
PVOID pvAlloc);
|
|
|
|
FORCEINLINE
|
|
VOID
|
|
ExAcquirePushLockExclusive(PEX_PUSH_LOCK PushLock)
|
|
{
|
|
/* Try acquiring the lock */
|
|
if (InterlockedBitTestAndSet((PLONG)PushLock, EX_PUSH_LOCK_LOCK_V))
|
|
{
|
|
/* Someone changed it, use the slow path */
|
|
ExfAcquirePushLockExclusive(PushLock);
|
|
}
|
|
}
|
|
|
|
FORCEINLINE
|
|
BOOLEAN
|
|
ExTryAcquirePushLockExclusive(PEX_PUSH_LOCK PushLock)
|
|
{
|
|
/* Try acquiring the lock */
|
|
return !InterlockedBitTestAndSet((PLONG)PushLock, EX_PUSH_LOCK_LOCK_V);
|
|
}
|
|
|
|
FORCEINLINE
|
|
VOID
|
|
ExReleasePushLockExclusive(PEX_PUSH_LOCK PushLock)
|
|
{
|
|
EX_PUSH_LOCK OldValue;
|
|
|
|
/* Unlock the pushlock */
|
|
OldValue.Value = InterlockedExchangeAddSizeT((PSIZE_T)PushLock,
|
|
-(SSIZE_T)EX_PUSH_LOCK_LOCK);
|
|
/* Check if anyone is waiting on it and it's not already waking */
|
|
if ((OldValue.Waiting) && !(OldValue.Waking))
|
|
{
|
|
/* Wake it up */
|
|
ExfTryToWakePushLock(PushLock);
|
|
}
|
|
}
|
|
|
|
FORCEINLINE
|
|
VOID
|
|
_ExInitializePushLock(PEX_PUSH_LOCK Lock)
|
|
{
|
|
*(PULONG_PTR)Lock = 0;
|
|
}
|
|
#define ExInitializePushLock _ExInitializePushLock
|
|
|
|
NTSTATUS FASTCALL
|
|
IntSafeCopyUnicodeString(PUNICODE_STRING Dest,
|
|
PUNICODE_STRING Source);
|
|
|
|
NTSTATUS FASTCALL
|
|
IntSafeCopyUnicodeStringTerminateNULL(PUNICODE_STRING Dest,
|
|
PUNICODE_STRING Source);
|
|
|
|
HBITMAP NTAPI UserLoadImage(PCWSTR);
|
|
|
|
BOOL NTAPI W32kDosPathNameToNtPathName(PCWSTR, PUNICODE_STRING);
|
|
|